Amazon is known for its rigorous interview process, designed to evaluate candidates' technical skills, problem-solving abilities, and cultural fit. Here, we'll dive into some real Amazon interview questions, providing a glimpse into what candidates might face.
1. String Manipulation
Question: Given a string of even length, return the first half. So the string "WooHoo" yields "Woo".
Solution: This problem tests your basic understanding of string manipulation. You need to find the midpoint of the string and return the substring from the start to the midpoint.
Example Code:
def first_half(string):
mid = len(string) // 2
return string[:mid]
print(first_half("WooHoo")) # Output: "Woo"
2. Working with Dictionaries
Question: Having the following list of dictionaries, print the id with the largest amount.
values = [
{'id': 1, 'date': '2018-10-30', 'amount': 100},
{'id': 2, 'date': '2018-10-30', 'amount': 500},
{'id': 3, 'date': '2018-10-30', 'amount': 240},
{'id': 4, 'date': '2018-10-30', 'amount': 300},
{'id': 5, 'date': '2018-10-01', 'amount': None},
]
Solution: This problem evaluates your ability to handle dictionaries and perform operations like finding the maximum value.
Example Code:
def max_amount_id(values):
max_value = max([v for v in values if v['amount'] is not None], key=lambda x: x['amount'])
return max_value['id']
print(max_amount_id(values)) # Output: 2
3. Prime Number Check
Question: Given a positive number, return if it is prime or not.
Solution: This problem tests your ability to implement a basic algorithm to check for prime numbers.
Example Code:
def is_prime(n):
if n <= 1:
return False
for i in range(2, int(n ** 0.5) + 1):
if n % i == 0:
return False
return True
print(is_prime(11)) # Output: True
print(is_prime(4)) # Output: False
4. SQL Queries
Scenario: Given the following tables:
- Employee: Contains employee details including employeeid, employeename, and departmentid.
- Department: Contains department details including departmentid and departmentname.
- Salary: Contains salary details including salaryid, employeeid, date, and salary.
Questions:
- Write an SQL query to identify the maximum salary per month.
- Write an SQL query to identify the maximum salary per department per pay month.
Solutions:
- Maximum Salary per Month:
SELECT DATE_FORMAT(date, '%Y-%m') AS month, MAX(salary) AS max_salary
FROM Salary
GROUP BY DATE_FORMAT(date, '%Y-%m');
- Maximum Salary per Department per Pay Month:
d.departmentname, DATE_FORMAT(s.date, '%Y-%m') AS month, MAX(s.salary) AS max_salary
FROM Salary s
JOIN Employee e ON s.employeeid = e.employeeid
JOIN Department d ON e.departmentid = d.departmentid
GROUP BY d.departmentname, DATE_FORMAT(s.date, '%Y-%m');
Conclusion
Amazon's interview questions span a wide range of topics, from basic string manipulation and working with data structures to more complex algorithmic challenges and SQL queries. These examples illustrate the depth and variety of problems you may encounter during the interview process. Preparing for these types of questions will help you develop the problem-solving skills and technical knowledge necessary to succeed.
In conclusion, Amazon's interview process involves a variety of technical and problem-solving questions designed to assess a candidate's skills comprehensively. From string manipulation and dictionary operations to prime number checking and SQL queries, the questions require a solid understanding of core programming concepts and the ability to apply them effectively.
If you need assistance preparing for your Amazon interview, we offer interview coaching and support services. Our team is available to provide real-time support during your online interviews. Contact us to purchase our services and enhance your interview readiness.
我们提供代面试服务,面试辅助服务,能够即时的支持您的线上面试,欢迎联系我们以购买服务。
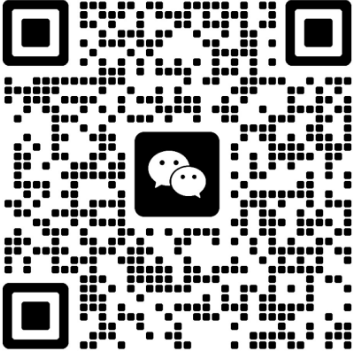