Question 1
Given: The initial setup of a match between two players. Your task is to evaluate the match's outcome.
Description: There are two players, and there is a number sequence of size n
. Players alternate turns for n
rounds. Each round, a player removes the first number from the sequence and adds its value to their score. After that, if the 'removed' number is even, the remaining sequence is reversed. The goal is to determine the difference in scores between the two players after the game.
More precisely, suppose first_score
and second_score
are the final scores of the first and second player, respectively. The goal is to calculate the value of first_score - second_score
.
Example: The number of elements is n = 5
and numSeq = [3, 6, 2, 3, 5]
.
- 1st round: The first player picks
3
,first_score = 3
. The remaining sequence:[6, 2, 3, 5]
. - 2nd round: The second player picks
6
,second_score = 6
. Since 6 is even, the remaining sequence is reversed:[5, 3, 2, 1]
. - 3rd round: The first player picks
5
,first_score = 3 + 5
. The remaining sequence:[3, 2, 1]
.
考察点 (Key Points)
- 数组操作 (Array Manipulation): 这道题目要求对数组进行多次操作,如取首个元素并在某些情况下对其进行翻转。
- 条件判断 (Conditional Statements): 根据选取的数字是否为偶数来决定是否翻转数组。
- 循环控制 (Loop Control): 通过循环来实现玩家轮流选择数字。
详细题解 (Detailed Solution)
- 初始化 (Initialization): 为了控制从数组的哪一端取数字,我们需要初始化两个指针
l
和r
,分别表示数组的开始和结束。此外,还需要一个flag
来标记当前是哪个玩家的回合,以及cnt
来记录已经进行了多少回合。 - 玩家选择 (Player's Choice): 在每个回合中,根据
flag
的值从数组的开始或结束取出一个数字,然后更新l
或r
的值。 - 更新得分 (Update Scores): 如果是第一个玩家的回合,就将选取的数字加到答案中;如果是第二个玩家的回合,就从答案中减去选取的数字。
- 翻转数组 (Reverse Array): 如果选取的数字是偶数,就翻转
flag
的值,这样下一个玩家将从数组的另一端取数字。 - 结束判断 (Termination): 当
l
大于r
时,所有数字都已被选取,循环结束。
注意事项 (Notes)
- 正确翻转 (Correct Reversion): 需要确保只有在选取偶数时才翻转数组。这一点在代码中通过
flag
实现。 - 确保交替 (Ensure Alternation): 要确保玩家们能够正确交替进行。这可以通过检查
cnt
的值是否为偶数来实现。 - 边界条件 (Boundary Conditions): 要确保在取数字时不超出数组的边界,这是通过在循环中检查
l
和r
的关系来实现的。
- Initialization: To control from which end of the array we're drawing numbers, we initialize two pointers,
l
andr
, representing the start and the end of the array respectively. Additionally, we'll need aflag
to indicate whose turn it is (which end of the array to draw from) and acnt
to keep track of the number of turns passed. - Player's Choice: In each round, based on the value of the
flag
, we pick a number from either the start or the end of the array. We then update the value ofl
orr
accordingly. - Update Scores: If it's the first player's turn (determined by whether the value of
cnt
is even), the chosen number is added to the score (stored in theanswer
variable). If it's the second player's turn, the chosen number is subtracted from the score. - Array Reversion: If the chosen number is even, we flip the value of
flag
. This ensures that the next number will be picked from the other end of the array on the next turn. - Termination: The loop terminates when all numbers from the array have been chosen, which is determined by the condition
l > r
.
Notes:
- Correct Reversion: Ensure that the array is reversed only when an even number is picked. This is achieved in the code using the
flag
. - Ensure Alternation: Ensure that the players alternate turns correctly. This is achieved by checking if the value of
cnt
is even. - Boundary Conditions: Make sure not to go beyond the array's boundaries when picking numbers. This is achieved by checking the relationship between
l
andr
in the loop condition.
This explanation should provide a comprehensive understanding of the problem and the provided code.
参考答案
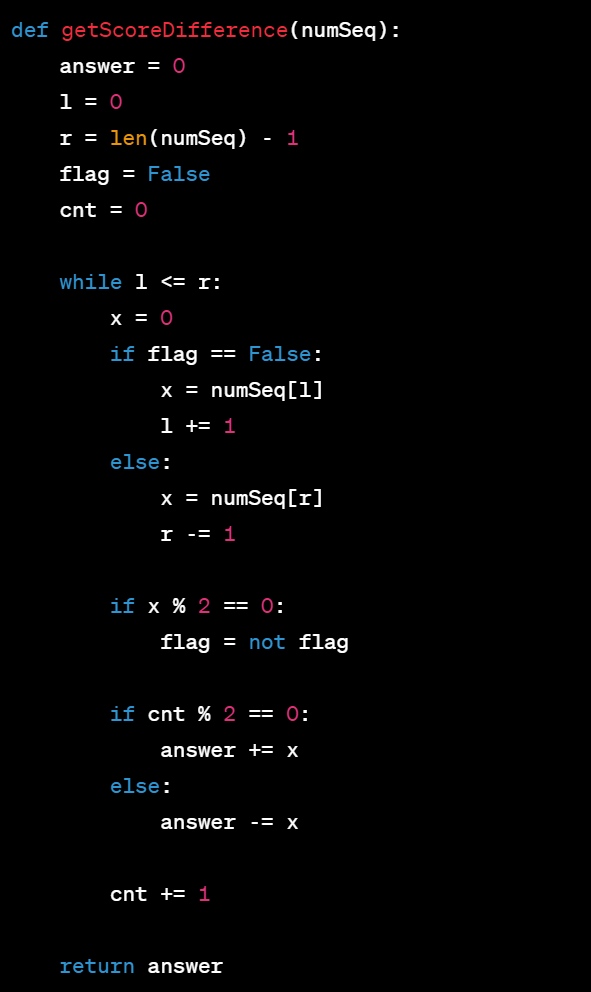
Question 2
You are given a log file with a list of GET requests delimited with double quotes and spaces.
A sample and the structure of the text file containing the log entries are given below.
Sample log record:
arduinoCopy code
unicomp6.unicomp.net - - [01/Jul/1995:00:00:06 -0400] "GET /shuttle/countdown/ HTTP/1.0" 200 3985
Log file structure:
Hostname | - | - | Timestamp | Request | Status | Bytes |
---|---|---|---|---|---|---|
unicomp6.unicomp.net | - | - | [01/Jul/1995:00:00:06 -0400] | "GET /shuttle/countdown/ HTTP/1.0" | 200 | 3985 |
Given a filename
that denotes a text file in the current working directory. Create an output file with the name "bytes_" prefixed to the filename (bytes_filename
) which stores the information about large responses.
Example: filename = "hosts_access_log_00.txt"; process the records in hosts_access_log_00.txt and create an output file named bytes_hosts_access_log_00.txt.
Write the following to the output file:
- The first line must contain the number of requests that have more than 5000 bytes sent in their response.
- The second line must contain the total sum of bytes sent by all responses sending more than 5000 bytes.
Note: The output file has to be written to the current directory.
Constraints: It is guaranteed that the total number of bytes in the log file does not exceed 2^31.
题解:
问题: 读取指定的日志文件,查找那些响应字节数大于5000的GET请求,然后将这些大请求的数量和它们的总字节数写入一个新的输出文件。
解题步骤:
- 打开并读取指定的文件。
- 对于文件中的每一行,按照空格进行分割,从而获取请求的各个部分。
- 从分割后的数据中,取出最后一个元素,即响应的字节数。
- 检查此响应的字节数是否大于5000。如果是,更新大请求的计数器和字节总数。
- 读取完文件后,创建一个新的输出文件,其文件名前缀为"bytes_"。
- 将大请求的数量写入输出文件的第一行,将字节总数写入第二行。
Solution:
Problem: Read the specified log file, identify the GET requests with a response size greater than 5000 bytes, and write the count of these large requests and their total byte size to a new output file.
Steps to solve:
- Open and read the specified file.
- For each line in the file, split by spaces to get the components of the request.
- From the split data, retrieve the last element, which represents the byte size of the response.
- Check if this response size is greater than 5000. If so, update the counter for large requests and the total bytes.
- After reading the entire file, create a new output file with a "bytes_" prefix to its name.
- Write the count of large requests to the first line of the output file and the total bytes to the second line.
参考答案
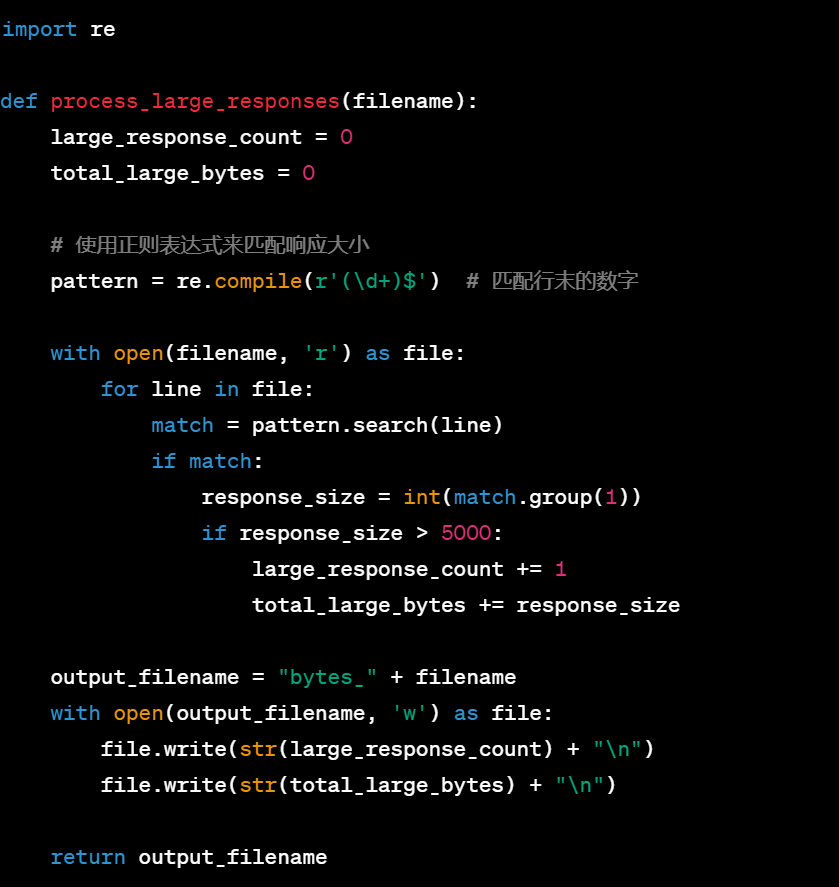
担心拿不到offer,OA不会做,VO没准备怎么办?请联系我
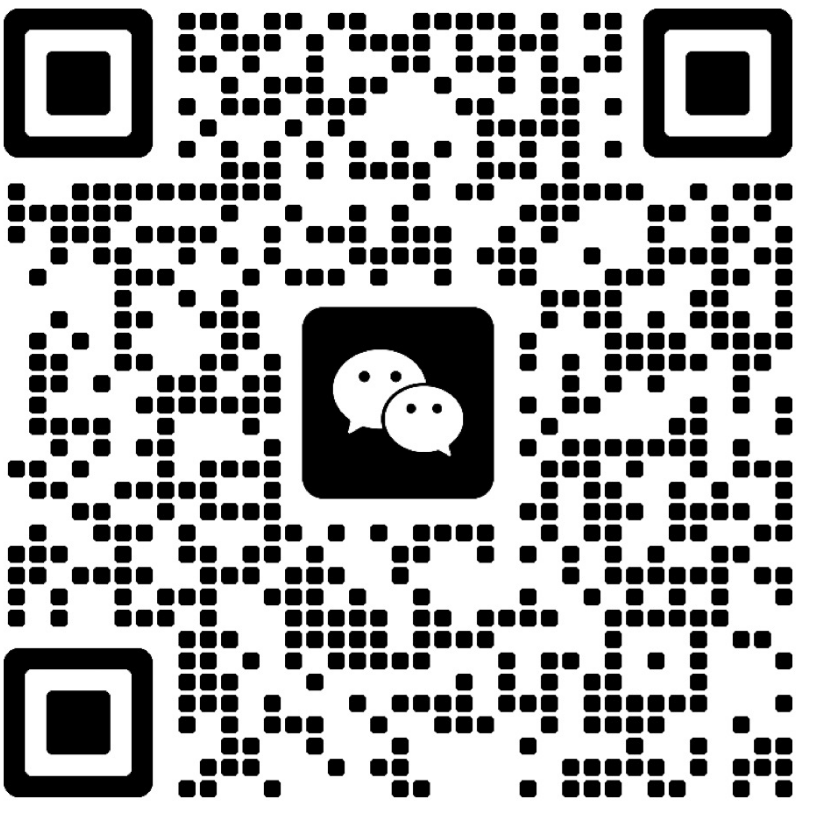