Level 1: Basic Account Operations
Implement three functions: CreateAccount
, Deposit
, and Withdraw
.
CreateAccount
def CreateAccount(timestamp, accountId):
# Create a new account with the given accountId and initialize balance to 0
Deposit
def Deposit(timestamp, accountId, amount):
# Deposit the given amount into the account
# Edge Case: If the account does not exist, handle appropriately
Withdraw
def Withdraw(timestamp, accountId, amount):
# Withdraw the given amount from the account
# Edge Case: If the account does not exist or balance is insufficient, handle appropriately
Level 2: TopActivity
Implement a function to return the top N accounts based on the number of operations (both deposit and withdraw).
TopActivity
def TopActivity(n):
# Return a list of top n accounts sorted by the number of operations
# Each operation counts equally, whether it is deposit or withdraw
Note: Initially it might seem like the sorting is by balance, but it is actually by operation count.
Level 3: Transfer with Acceptance
Implement transfer-related functionality with a 24-hour acceptance window.
Transfer
def Transfer(timestamp, sourceAccountId, targetAccountId, amount):
# Initiate a transfer of amount from source to target
# Balance of source is immediately reduced upon calling Transfer
# Transfer must be accepted within 24 hours by the target
AcceptTransfer
def AcceptTransfer(timestamp, targetAccountId, amount):
# Target account explicitly accepts the transfer within 24 hours
# If not accepted, the transfer becomes invalid
Explanation
- When
Transfer
is called, the source account’s balance is immediately deducted, even beforeAcceptTransfer
. - If
AcceptTransfer
is not called within 24 hours, the transfer is canceled or invalidated, but the balance handling logic might need compensation later. - Based on the unit tests, the expectation is that balance deduction happens immediately on transfer initiation, not after acceptance.
Level 4: Merge Accounts
Merge two accounts into one.
Although details were not provided, typically a MergeAccount
function would look like:
MergeAccount
def MergeAccount(timestamp, sourceAccountId, targetAccountId):
# Merge source account into target account
# Transfer balance and possibly transaction history
# Source account is closed after merging
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
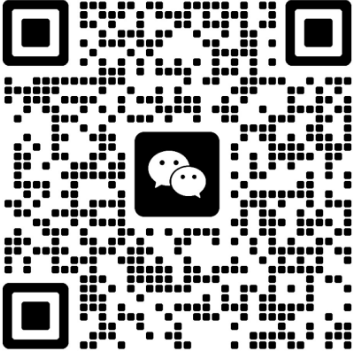