Question 1 / 3 - Language independent
Write a program which computes the price of a macaron order.
- Each macaron has the same unit price (given as input).
- Each macaron has a specific flavor.
- There are at most 5 distinct flavors among all the macarons.
An order is made of one or more sets
A specific set can contain up to 5 macarons but cannot contain macarons of identical flavors.
The baker always tries to fill up his 5-slots sets at the maximum. The order of macarons inside a set doesn't matter, and the order of sets doesn't matter either.
The baker offers a discount based on the number of different flavors in a set:
- 2 different flavors in the set = -10% on the price of the set
- 3 different flavors in the set = -20% on the price of the set
- 4 different flavors in the set = -30% on the price of the set
- 5 different flavors in the set = -40% on the price of the set
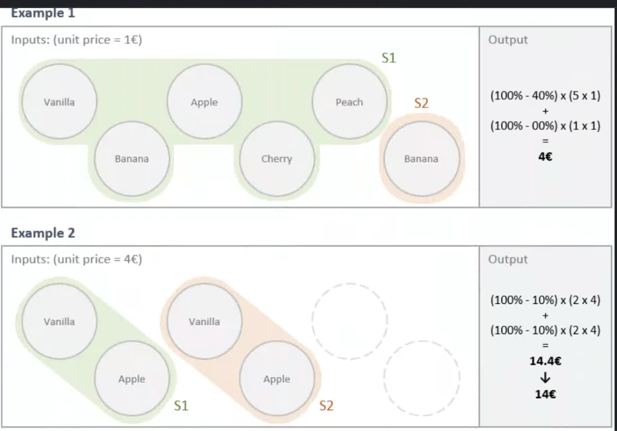
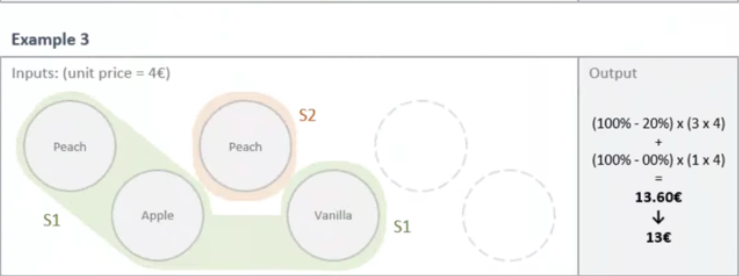
Function Specification
def compute_total_price(unit_price, macarons):
- Parameters:
unit_price
: a macaron unit pricemacarons
: a list of strings corresponding to each macaron flavor
- Returns:
- the total price of the order as an integer (rounded down if the total is a float number)
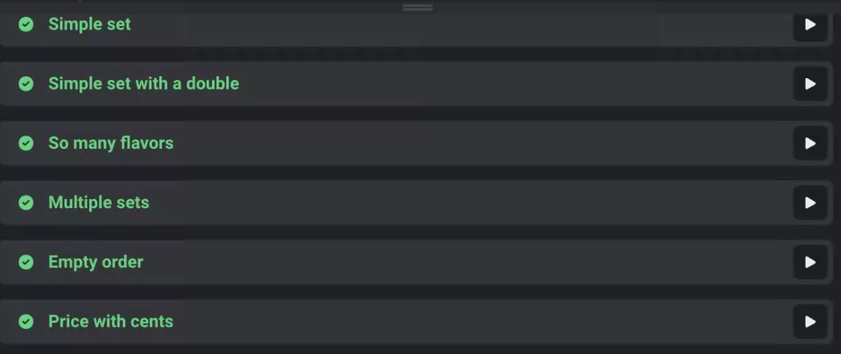
Question 2 / 3 - Language independent
Objective
It is a windy day today and many tree leaves are covering the garden. Write a program that calculates the number of leaves left in the garden based on their location and a series of wind gusts.
How it works
- The ground is represented by a grid that is
height
squares high andwidth
squares wide. - The top left box is located at (0, 0) where the first integer represents the row and the second the column.
- Each element of the grid represents the number of leaves on the ground.
- The series of gusts,
winds
, is a string composed of the charactersU
(top),D
(bottom),L
(left),R
(right). - Each gust of wind moves the leaves on the grid one square in the direction of the wind.
- The leaves fly out of the garden when the wind pushes them out of the grid.
Implementation
Implement the function remaining_leaves(width, height, leaves, winds)
which:
- Takes as inputs the integers
width
andheight
, the 2D integer arrayleaves
, and the stringwinds
with:- 0 <
width
< 20 - 0 <
height
< 20 - 0 <=
leaves
< 20 - 0 <= number of characters in
winds
< 20
- 0 <
- Returns the number of leaves left on the floor as an integer.
Important note: in
leaves
, the first integer represents rows and the second integer represents columns.
Example
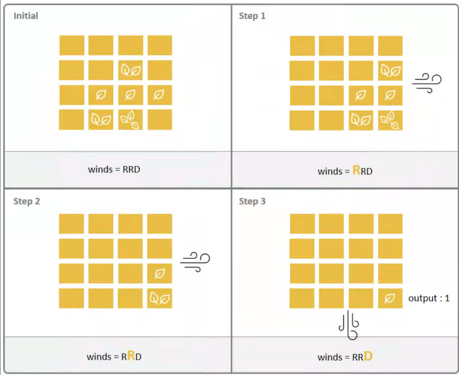
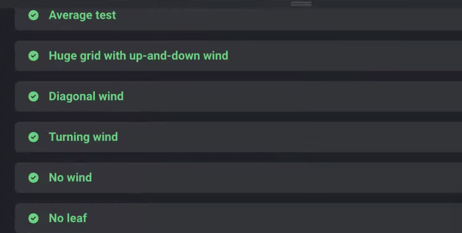
Question 3 / 3 - Language independent
Objective
You are given a list of integers, which represent the monthly net profits of a company. The company wants you to look for the longest sequence of chronologically ordered months that have increasing profit, and return the length. The sequence can include non-adjacent months.
Implementation
Implement the function longest_profit(data)
which takes as input a list of integers data
, representing a given set of consecutive monthly profit values. An example input would look like this:
[-1, 9, 0, 8, -5, 6, -24]
Your longest_profit
function should return an integer representing the length of the longest sequence of increasing monthly profits. For the above example, the correct output would be the following integer:
3
(the sequence could be [-1, 0, 6]
or [-1, 0, 8]
)
More examples
[1, -4, 5, -3] => 2
[-3, -4, -5, -6] => 1
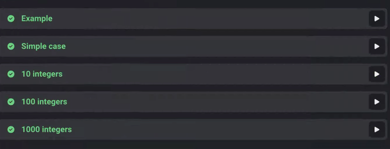
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
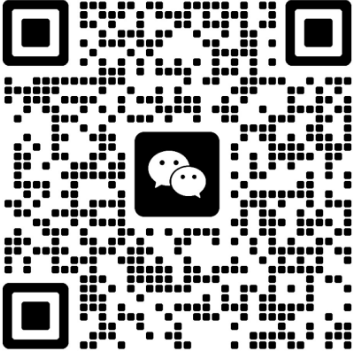