"I think I’ve seen this question before, should be able to code it up quickly." That was our candidate’s first thought when seeing this Atlassian interview question:
“Design a class RunningCommodityPrice with two methods:void upsertCommodityPrice(timestamp, price)
andint getMaxCommodityPrice()
to always return the latest highest commodity price.”
It looked clean, familiar, and somewhat like a "LeetCode easy" question. But the interview quickly showed that this wasn’t a speed coding test — it was a deep dive into thinking, reasoning, and real engineering sense. And this is exactly where CSOAHELP’s real-time interview assistance stepped in — helping the candidate stay calm, respond precisely, and ultimately secure a second-round invite from Atlassian.
Here’s how it all played out.
The interviewer opened the Zoom call with a quick greeting and then immediately presented the problem, without much context. The candidate began enthusiastically: “We can maintain the max price using a heap…” which, of course, is a valid starting point. But before he started coding, our remote assistant, monitoring his screen, pushed a timely prompt:
“The interviewer is likely focused on the semantics of 'updating'. Can timestamps repeat? Are older values overwritten? How is consistency handled? You should clarify these constraints first.”
Reading the note, the candidate quickly asked: “Just to clarify, if I insert a new price for the same timestamp, does it overwrite the previous value?” The interviewer nodded: “Yes, it replaces the old one.”
That simple clarification was key. Based on our experience, the simpler the question looks, the easier it is to overlook important edge cases or modeling decisions.
Once the scope was clear, the candidate started working on the first version of the code. We quickly pushed a clean, interview-ready code snippet as a reference — the candidate could read it, paraphrase it, or just type it in line by line:
import heapq
class RunningCommodityPrice:
def __init__(self):
self.commodityPrice = {}
self.max_price = []
def upsertCommodityPrice(self, timestamp, price):
self.commodityPrice[timestamp] = price
heapq.heappush(self.max_price, (-price, timestamp))
def getMaxCommodityPrice(self):
if not self.commodityPrice:
return -1
while self.max_price:
neg_price, timestamp_max = self.max_price[0]
if -neg_price == self.commodityPrice[timestamp_max]:
return -neg_price
else:
heapq.heappop(self.max_price)
return -1
The interviewer nodded at the logic and asked to test the solution with a few sample cases. The candidate, using pre-prepared test cases and our guidance, explained why the heap needed to store negative prices to simulate a max-heap using Python’s heapq
.
Then the interviewer took it up a notch: “Looks good. But what if our service is receiving thousands of price updates per second — can this structure scale?”
This is where Atlassian tends to shift gears: from algorithm to engineering. The candidate paused briefly. On our second screen, we immediately pushed a structured response:
“The bottleneck is that getMaxCommodityPrice
might need multiple pops to find a valid price. For high-frequency updates, you can use lazy deletion with a deletion tracking set to avoid upfront cleanup while preserving consistency.”
The candidate read the suggestion and replied fluently: “You're right, in high-frequency scenarios, the heap might accumulate outdated values. I’d maintain a set of invalidated entries and lazily clean the heap during queries, reducing update overhead while keeping consistent results.”
The interviewer responded positively: “Great. Can you implement that lazy cleanup logic?” We instantly sent over the optimized version of the relevant function:
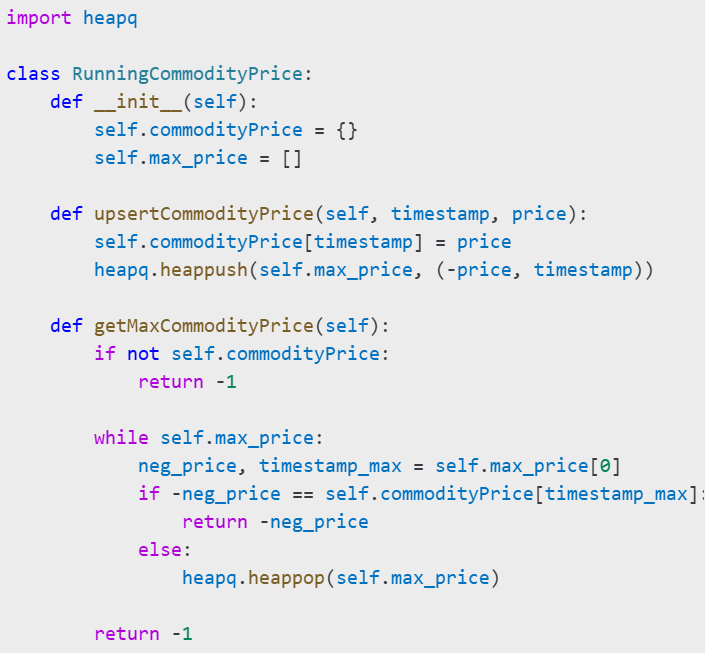
We reminded the candidate that the dictionary in upsertCommodityPrice
still handled the latest mapping, so no extra pre-cleaning was needed. He implemented it as shown and walked the interviewer through his reasoning. Approval granted.
Then came the final curveball. The interviewer asked: “If we need to support different commodity types in the future, how would you scale your design?”
We sent another structured talking point:
“Consider these options: One, use a nested dictionary with commodity type as the first key and timestamp as the second. Two, abstract a CommodityManager
class managing separate RunningCommodityPrice
instances. Three, if time-series querying is required later, integrate data structures like skip lists or time trees.”
The candidate incorporated the keywords and delivered a clear, modular expansion strategy. The interviewer seemed satisfied and wrapped up the session.
This was a real case from an Atlassian interview supported live by CSOAHELP. From interpreting the question to refining edge cases, writing code, optimizing performance, and presenting design extensions — our team was there at every step. The candidate’s raw skills were limited, but with structured nudges, relevant prompts, and ready-to-use code snippets, he looked confident and well-prepared.
The truth is, many people don’t fail interviews because they can’t solve problems — they fail because, under pressure, they struggle to communicate what they already know. What we do is help you clarify, organize, and say the right things. And when needed, we give you copy-ready logic or phrasing you can use directly.
Interviews today aren’t just coding battles. They're high-stakes conversations. Companies like Atlassian, Stripe, Apple, and Google care more about how you handle live challenges than about how many problems you’ve solved on LeetCode.
CSOAHELP’s real-time interview assistance isn’t about cheating. It’s about helping you think clearly, speak strategically, and show your best side — right when it matters most.
You just shine on camera. We’ve got your back off-screen.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
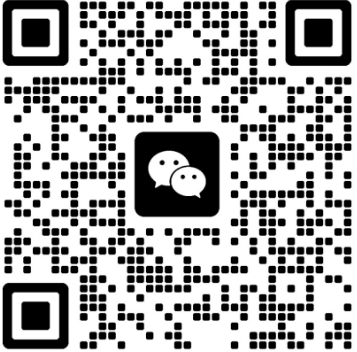