For many candidates, a Microsoft technical interview is not just a test of skills—it’s a psychological challenge. Even after practicing hundreds of LeetCode problems, many candidates still struggle under pressure, losing their train of thought, getting stuck, or failing to articulate their ideas clearly.
Today, we’ll walk through a real Microsoft interview experience from a CSOAHELP client, showing how remote real-time interview assistance transformed a difficult situation into a smooth success.
This was a virtual interview conducted via Microsoft Teams. After a brief introduction, the interviewer went straight to the coding question, displaying the following prompt in the shared code editor:
Problem Statement:
Terms related to accessibility are often abbreviated, such as i18n (internationalization), l10n (localization), and a11y (accessibility). The abbreviation rule is to keep the first and last character while replacing the middle characters with the number of omitted letters.Given a list of words, determine the most frequently occurring abbreviation(s).
Example input:
string[] Words = new string[]{
"internationalization",
"localization",
"accessibility",
"kubernetes",
"hands",
"chase",
"taxes",
"homes",
"apple",
"water",
"hello",
"world",
"hoops",
};
The candidate froze as he read the problem. This was not a typical LeetCode question; it required a combination of string manipulation and frequency counting, a style commonly used in Microsoft’s interviews to test engineering thinking. His first instinct was to use a dictionary to track abbreviation frequencies, but the pressure of the interview made his thought process disorganized, and he struggled to write even the basic logic.
He hesitated and said, “Uh… I think we need to generate abbreviations first and then… uh… count them.” The interviewer waited for a moment and, seeing that he wasn’t continuing, suggested, “You can start by writing a function that converts a word into its abbreviation.”
However, the candidate remained stuck, his fingers frozen on the keyboard.
At this crucial moment, CSOAHELP’s remote interview assistance team provided a complete breakdown of the problem and a code template on his secondary screen.
Full Solution Breakdown and Code:
Start by implementing a function that converts a word into an abbreviation:
- If the word length is 2 or less, return the word as-is.
- Otherwise, return first letter + (length - 2) + last letter.
Then, iterate through the list, convert words into abbreviations, and use a dictionary to count their occurrences.
Finally, find the most frequently occurring abbreviation(s).Code:
string Abbreviate(string word) {
if (word.Length <= 2) return word;
return word[0] + (word.Length - 2).ToString() + word[word.Length - 1];
}
Dictionary<string, int> CountAbbreviations(string[] words) {
Dictionary<string, int> abbreviationCounts = new Dictionary<string, int>();
foreach (string word in words) {
string abbr = Abbreviate(word);
if (!abbreviationCounts.ContainsKey(abbr))
abbreviationCounts[abbr] = 0;
abbreviationCounts[abbr]++;
}
return abbreviationCounts;
}
List<string> MostCommonAbbreviations(string[] words) {
Dictionary<string, int> counts = CountAbbreviations(words);
int maxCount = counts.Values.Max();
return counts.Where(pair => pair.Value == maxCount)
.Select(pair => pair.Key)
.ToList();
}
Upon seeing the complete code, the candidate regained his confidence. He began repeating the logic aloud while implementing his own version.
He started by writing the abbreviation function:
string Abbreviate(string word) {
if (word.Length <= 2) return word;
return word[0] + (word.Length - 2).ToString() + word[word.Length - 1];
}
The interviewer nodded and said, “Good, now let’s count the frequencies.”
Following CSOAHELP’s code, he implemented the frequency count logic:
Dictionary<string, int> CountAbbreviations(string[] words) {
Dictionary<string, int> abbreviationCounts = new Dictionary<string, int>();
foreach (string word in words) {
string abbr = Abbreviate(word);
if (!abbreviationCounts.ContainsKey(abbr))
abbreviationCounts[abbr] = 0;
abbreviationCounts[abbr]++;
}
return abbreviationCounts;
}
The interviewer acknowledged the progress and said, “Nice. Now, how do you find the most common abbreviation?”
The candidate then implemented the final part:
List<string> MostCommonAbbreviations(string[] words) {
Dictionary<string, int> counts = CountAbbreviations(words);
int maxCount = counts.Values.Max();
return counts.Where(pair => pair.Value == maxCount)
.Select(pair => pair.Key)
.ToList();
}
The interviewer smiled, indicating approval—the candidate had successfully implemented the complete solution.
Microsoft interviews don’t end at the first solution. The interviewer followed up with another question: “How would you optimize this solution for large datasets?”
CSOAHELP’s real-time assistance once again provided a complete response:
Optimization Ideas:
- If the dataset is large, use a Trie structure to store abbreviations and reduce redundant computations.
- Implement streaming processing to handle large input efficiently.
- Use LRU caching to store recently computed abbreviations for improved performance.
Optimized Code Example (LRU Cache):
using System.Collections.Generic;
class AbbreviationCache {
private Dictionary<string, string> cache = new Dictionary<string, string>();
public string GetAbbreviation(string word) {
if (!cache.ContainsKey(word)) {
cache[word] = Abbreviate(word);
}
return cache[word];
}
}
The candidate repeated the reasoning and expanded on some points with his own insights. The interviewer nodded in approval, signaling the successful conclusion of the interview.
Shortly after, the candidate received an email from Microsoft inviting him to the next interview round! He later shared his thoughts: “Without CSOAHELP, I would have been stuck on the first step. This remote assistance allowed me not only to complete the coding task but also to confidently answer the follow-up questions.”
Many candidates fail Microsoft interviews not because of a lack of technical skills, but because of difficulty organizing thoughts, articulating ideas clearly, and handling unexpected questions under pressure. CSOAHELP’s remote interview assistance provides:
✔ Complete coding solutions to prevent getting stuck
✔ Ideal answers to anticipated follow-up questions
✔ Code optimization strategies to demonstrate engineering skills
✔ Seamless, undetectable support that enhances real interview performance
If you don’t want to lose a top-tier offer due to anxiety or disorganized thinking, CSOAHELP is your ultimate interview partner! 🚀
Get in touch with CSOAHELP today and secure your offer from Microsoft, Google, or Meta!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
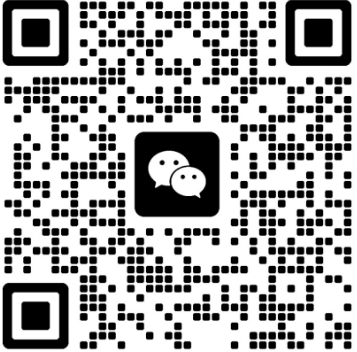