onsider a non-binary tree data structure. One representation of this might be (in pseudo-code):
class Node:
key: int (0 <= key <= 10,000)
value: int (0 <= value <= 1,000,000,000)
children: list[Node] (or Node pointers in C++)
Each node of the tree has a key, value, and references to each of its children. Children are ordered—the order of the children list matters. A node with no children will have an empty list for its children
attribute. Throughout this explanation, we will use the shorthand a@b
to refer to a node with key a
and value b
.
We will pass in trees as a list of lists of ints. Each row of the input is itself a list of ints that represents a node. The format of this list of ints is as follows:
- The first element represents that node's key.
- The second element represents that node's value.
- All subsequent elements represent the keys of this node's children (in order).
The rows may be passed in any arbitrary order, but you can assume that they form a well-formed tree with a unique root. For any given node, all its children must have unique keys (the input is guaranteed to satisfy this). This is the only uniqueness guarantee—two nodes that are not children of the same parent can have the same key, and any two nodes can have the same value.
Example Input:
[
[4, 2],
[1, 15, 3, 2, 0],
[6, 3, 2],
[0, 4],
[3, 42]
]
Interpretation:
- Row
[4, 2]
: Node with key 4 and value 2. - Row
[1, 15, 3, 2, 0]
: Node with key 1, value 15, and children corresponding to rows with keys 3, 2, and 0. - Row
[6, 3, 2]
: Node with key 6, value 3, and child corresponding to row with key 2. - Row
[0, 4]
: Node with key 0 and value 4. - Row
[3, 42]
: Node with key 3 and value 42.
The resultant tree looks something like this (where the node names are formatted key@value
):
1@15
├── 3@42
├── 2@3
│ └── 6@3
└── 0@4
Recap of Previous Part
Write code to parse a list of lists into a tree. Then, traverse the tree pre-order and return a list of the node keys and values along this traversal—formatted [key_1, value_1, key_2, value_2, ..., key_n, value_n]
.
A pre-order traversal is a traversal of all the nodes in the tree that satisfies the following:
- A parent node is traversed before any of its children.
- Suppose Node A and Node B are children of the same parent, and A occurs before B in the parent's children list. Then Node A and all elements of Node A's subtree must occur before Node B and all elements of Node B's subtree in the traversal.
Example output for the input above:[1, 15, 6, 3, 1, 0, 2]
.
You will receive as input:
- n, an integer, denoting the shape of the board (n × n).
- moves, of the form
P X Y
, whereP ∈ {W, B}
specifies the player,X
andY
are 0-indexed coordinates, where right > left and bottom > top. The moves are in order, so you should parse them from the first element to the last. There is no guarantee that the two players will alternate.
As output, you should produce a single line b w
, where b
and w
are the number of Black and White pieces remaining on the board.
Execution Constraints:
- Execution time limit: 4 seconds (py3).
- Memory limit: 1 GB.
Input:
- integer n: The size of the board (which is of shape n × n).
- array[string] moves: A list of strings
P X Y
, whereP ∈ {W, B}
specifies the player, andX
andY
are 0-indexed coordinates.
Output:
- string: Two integers separated by a space, representing the number of Black and White pieces at the end of the game respectively.
Example Board Representation:
Initial board state:
0 1 2 3
0 . . . .
1 . W W W
2 . . . .
3 . . . .
Move:
- Black plays at (2, 2), flipping two White pieces at (1, 1) and (2, 1).
Resulting board state:
0 1 2 3
0 B . . .
1 B B . W
2 . B B .
3 . . . .
Python 3 Syntax Tips:
# Prints help message to the console
# Returns a string
def helloWorld(name):
print("This prints to the console when you run Testa")
return "Hello, " + name
Part 1
Write code to parse a list of lists into a tree. Then, traverse the tree pre-order and return a list of the node keys and values along this traversal—formatted [key_1, value_1, key_2, value_2, ..., key_n, value_n]
.
A pre-order traversal is a traversal of all the nodes in the tree that satisfies the following:
- A parent node is traversed before any of its children.
- Suppose Node A and Node B are children of the same parent, and A occurs before B in the parent’s children list. Then Node A and all elements of Node A's subtree must occur before Node B and all elements of Node B's subtree in the traversal.
For example, the preorder traversal of the tree from the example input would be [1015, 63, 100, 203, 402]
.
Since you are asked to output the flattened traversal in key-value format, the output should be [1, 15, 6, 3, 1, 0, 2]
.
For this level, your method signature should look like (in C++/Python, respectively):
C++:
vector<int> solution(vector<vector<int>> arg1);
Python:
def solution(arg1: list[list[int]]) -> list[int]:
pass
[Execution time limit] 4 seconds (py3)
[Memory limit] 1 GB
[Input] array.array.integer arg1
- (list of list of ints): List of rows where every row is a list of integers that corresponds to a node in the tree.
[Output] array.integer
- Sequence of values
[key_1, value_1, key_2, value_2, ..., key_n, value_n]
from traversing the tree pre-order.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
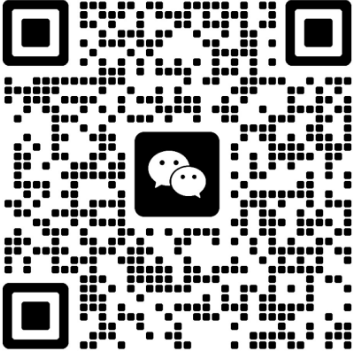