Implement support for different users sending queries to the system. All users share a common filesystem in the cloud storage, but each user is assigned an individual storage capacity limit.
- add_user(self, user_id: str, capacity: int) -> bool
Should add a new user to the system, withcapacity
as their storage limit in bytes. The total size of all files owned byuser_id
cannot exceedcapacity
. The operation fails if a user withuser_id
already exists. ReturnsTrue
if a user withuser_id
is successfully created, orFalse
otherwise. - add_file_by(self, user_id: str, name: str, size: int) -> int | None
Should behave in the same way as theadd_file
from Level 1, but the added file should be owned by the user withuser_id
. A new file cannot be added to the storage if doing so will exceed the user'scapacity
limit. Returns the remaining storage capacity foruser_id
if the file is successfully added orNone
otherwise. Note:- All queries calling the
add_file
operation implemented during Level 1 are run by the user withuser_id = "admin"
, who has unlimited storage capacity. - Assume that the
copy_file
operation preserves the ownership of the original file.
- All queries calling the
- update_capacity(self, user_id: str, capacity: int) -> int | None
Should change the maximum storage capacity for the user withuser_id
. If the total size of all user's files exceeds the newcapacity
, the largest files (sorted lexicographically in case of a tie) should be removed from the storage until the total size of all remaining files will no longer exceed the newcapacity
. Returns the number of removed files, orNone
if a user withuser_id
does not exist.
Examples
The example below shows how these operations should work:
Queries
add_user("user1", 125)
- Returns
True
; creates user "user1".
- Returns
add_user("user1", 100)
- Returns
False
; "user1" already exists.
- Returns
add_user("user2", 100)
- Returns
True
; creates user "user2".
- Returns
add_file_by("user2", "/file.med", 40)
- Returns
75
.
- Returns
add_file_by("user1", "/dir/file.big", 50)
- Returns
45
.
- Returns
add_file_by("user1", "/file.med", 30)
- Returns
None
; file named "/file.med" already exists.
- Returns
copy_file("/file.med", "/dir/another/file.med")
- Returns
True
; copying preserves ownership.
- Returns
copy_file("/dir/admin/file.med", "/dir/another/another/file.med")
- Returns
False
; "user1" does not exist.
- Returns
add_file_by("user1", "/dir/file.small", 10)
- Returns
5
.
- Returns
add_file_by("user1", "/my_folder/file.huge", 100)
- Returns
None
; "user1" does not have enough capacity.
- Returns
add_file_by("user3", "/my_folder/file.huge", 100)
- Returns
None
; "user3" doesn't exist.
- Returns
update_capacity("user1", 300)
- Returns
0
; all files owned by "user1" fit into the new capacity.
- Returns
update_capacity("user2", 50)
- Returns
2
; the files "/dir/file.big" and "/file.small" should be deleted to fit into the new capacity.
- Returns
update_capacity("user2", 1000)
- Returns
None
; "user2" does not exist.
- Returns
Level 4
Implement support for file compression.
- compress_file(self, user_id: str, name: str) -> int | None
Should compress the filename
if it belongs touser_id
. The compressed file should be replaced with a new file named<name>.COMPRESSED
. The size of the newly created file should be equal to half of the original file. The size of all files is guaranteed to be even, so there should be no fractional calculations. It is also guaranteed thatname
for this operation never points to a compressed file (i.e., it never ends with.COMPRESSED
). Compressed files should be owned byuser_id
—the owner of the original file. Returns the remaining storage capacity foruser_id
if the file was compressed successfully orNone
otherwise. Note:- Because file names can only contain lowercase letters, compressed files cannot be added via
add_file
. - It is guaranteed that all
copy_file
operations will preserve the suffix.COMPRESSED
.
- Because file names can only contain lowercase letters, compressed files cannot be added via
- decompress_file(self, user_id: str, name: str) -> int | None
Should revert the compression of the filename
if it belongs touser_id
. It is guaranteed thatname
for this operation always ends with.COMPRESSED
. If decompression results in theuser_id
exceeding their storage capacity limit or a decompressed version of the file with the given name already exists, the operation fails. Returns the remaining capacity ofuser_id
if the file was decompressed successfully orNone
otherwise.
Examples
The example below shows how these operations should work:
Queries
add_user("user1", 1000)
- Returns
True
.
- Returns
add_user("user2", 5000)
- Returns
True
.
- Returns
add_file_by("user1", "/dir/file.mp4", 500)
- Returns
500
.
- Returns
compress_file("user2", "/dir/file.mp4")
- Returns
None
; user2 does not own the file.
- Returns
compress_file("user1", "/dir/file.mp4")
- Returns
750
; the file is compressed to 250 bytes.
- Returns
compress_file("user1", "/folder/non_existing_file")
- Returns
None
; the file does not exist.
- Returns
compress_file("user1", "/dir/file.mp4")
- Returns
None
; the file is already compressed.
- Returns
get_file_size("/dir/file.mp4.COMPRESSED")
- Returns
250
.
- Returns
get_file_size("/dir/file.mp4")
- Returns
None
; the file no longer exists.
- Returns
copy_file("/dir/file.mp4.COMPRESSED", "/file.mp4.COMPRESSED")
- Returns
True
.
- Returns
add_file_by("user1", "/dir/file.mp4", 500)
- Returns
0
.
- Returns
decompress_file("user1", "/dir/file.mp4.COMPRESSED")
- Returns
None
; not enough capacity for decompression.
- Returns
update_capacity("user1", 2000)
- Returns
0
.
- Returns
decompress_file("user1", "/dir/file.mp4.COMPRESSED")
- Returns
750
.
- Returns
decompress_file("user2", "/dir/file.mp4.COMPRESSED")
- Returns
None
; user2 does not own the file.
- Returns
decompress_file("user1", "/dir/file.mp4.COMPRESSED")
- Returns
None
; the file is already decompressed.
- Returns
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
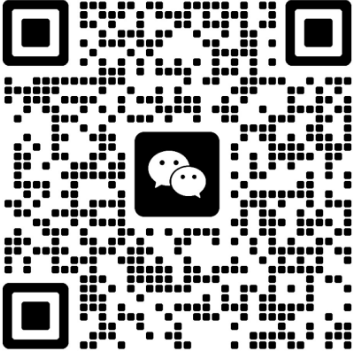