In high-stakes interviews at companies like Salesforce, technical questions are not just a test of coding skills—they’re designed to probe your ability to think critically, adapt under pressure, and articulate your reasoning. The Virtual Onsite (VO) stage is especially challenging, as candidates face layered questions and follow-ups that test their depth of understanding.
This article explores a real-life Salesforce interview question, “Two Operations,” and demonstrates how timely guidance from CSOAHELP can help candidates excel in such scenarios, all while maintaining a natural and confident demeanor.
The Question: Two Operations
The interview began with this deceptively simple problem:
Two integer operations are defined as:
1. ADD_1: Increment the integer by 1.
2. MULTIPLY_2: Multiply the integer by 2.
Given an integer value k, determine the minimum number of operations it takes to get from 0 to k using the two operations specified above.
Sample Input:
2
5
3
Sample Output:
4
3
Explanation:
- For
k = 5
:- The sequence of operations is
0 → 1 (ADD_1) → 2 (MULTIPLY_2) → 4 (MULTIPLY_2) → 5 (ADD_1)
, requiring 4 operations.
- The sequence of operations is
- For
k = 3
:- The sequence is
0 → 1 (ADD_1) → 2 (MULTIPLY_2) → 3 (ADD_1)
, requiring 3 operations.
- The sequence is
The problem is straightforward at first glance, but the real challenge lies in the follow-ups that demand deeper insight and optimization.
The Candidate's Solution
The candidate provided the following solution using a breadth-first search (BFS) approach:
from collections import deque
def getMinOperations(kValues):
def min_operations_to_target(k):
visited = set()
queue = deque([(k, 0)]) # (current value, steps)
while queue:
value, steps = queue.popleft()
if value == 0:
return steps
if value not in visited:
visited.add(value)
if value % 2 == 0:
queue.append((value // 2, steps + 1)) # Reverse MULTIPLY_2
queue.append((value - 1, steps + 1)) # Reverse ADD_1
return [min_operations_to_target(k) for k in kValues]
The candidate clearly explained their reasoning:
- Reverse Approach: Start from
k
and work backwards to0
. This ensures every step is optimal. - BFS: Guarantees the shortest path due to its level-wise exploration.
The solution passed the initial test cases, but Salesforce interviews rarely stop at implementation.
The Follow-Up Questions
After the initial solution, the interviewer pushed further:
- Why BFS over DFS?
- How would your solution scale if k is as large as 10^16?
- What if a new operation, such as DIVIDE_BY_2, is introduced?
These follow-ups are designed to probe the candidate’s problem-solving depth and ability to extend their solution. Such moments can be daunting, but with CSOAHELP providing real-time guidance, the candidate remained composed and delivered precise, professional responses.
CSOAHELP in Action
Unbeknownst to the interviewer, the candidate was equipped with CSOAHELP, a silent but powerful ally. Every response reflected the assistance provided by the system, enabling the candidate to address even the trickiest follow-ups with confidence.
When asked about the choice of BFS, the candidate promptly responded:
“BFS is ideal for finding the minimum number of operations because it explores all possibilities level by level, ensuring the shortest path to the solution.”
When pressed on optimizing the solution for large values of k
, the candidate replied:
“Caching intermediate results can help avoid redundant calculations, and limiting queue depth can reduce unnecessary exploration.”
Finally, when the interviewer introduced a new operation, DIVIDE_BY_2
, the candidate seamlessly integrated it:
if value % 2 == 0:
queue.append((value // 2, steps + 1))
They explained:
“Adding DIVIDE_BY_2 simply requires an additional condition in the BFS loop. The rest of the logic remains unchanged, showcasing the algorithm’s flexibility.”
The candidate’s composed demeanor and well-structured answers left the interviewer impressed, even though each response was guided by CSOAHELP.
Complexity Analysis
When prompted for a complexity analysis, the candidate delivered a thorough breakdown:
- Time Complexity: O(log k) for each value of
k
, as the reverse operations halve the value or decrement by 1 in each step. - Space Complexity: O(log k), accounting for the BFS queue and visited set.
This analysis further reinforced the candidate’s technical depth.
The Role of CSOAHELP
CSOAHELP played a pivotal role throughout the interview:
- Keyword Prompts: When the interviewer asked about BFS, CSOAHELP nudged the candidate to mention “level-wise exploration” and “guaranteed shortest path.”
- Follow-Up Guidance: For optimization and scalability questions, the system suggested strategies like caching and queue depth limitation.
- Code Adaptation: When new operations were introduced, CSOAHELP provided the precise logic needed to extend the algorithm.
Despite relying heavily on CSOAHELP, the candidate appeared entirely self-reliant and professional, thanks to the system’s discreet and seamless support.
The Takeaway
Salesforce interviews are as much about adaptability and critical thinking as they are about coding. The “Two Operations” problem demonstrates how a seemingly simple question can evolve into a complex discussion, testing the candidate’s ability to handle pressure and explore alternative solutions.
With CSOAHELP, candidates can navigate these challenges with confidence, presenting polished answers and well-reasoned explanations. By acting as an invisible safety net, CSOAHELP ensures that candidates perform at their best, even in the most demanding scenarios.
In a competitive landscape, CSOAHELP is the ultimate game-changer—helping candidates turn potential stress into an opportunity to shine.
csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
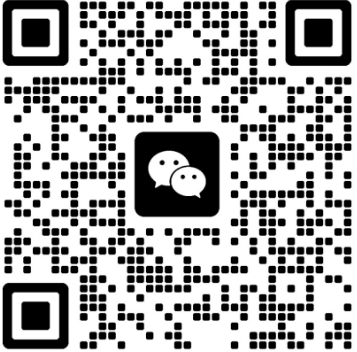