Implement a prototype of a friend recommendation system for a social media application.
There are n users indexed from 1 to n, and m friends represented as a 2d array, friendships
, where the ith friendship is a connection between users friendships[i][0]
and friendships[i][1]
.
User x is suggested as a friend to user y if x and y are not friends and have the maximum number of common friends, i.e., a friend of both x and y. If there are multiple possible such users x, the one with the minimum index is suggested.
Given n and friendships, for each of the n users, find the index of the friend that should be recommended to them. If there is no recommendation available, report -1.
Example
Suppose n = 5
, m = 5
, and connections = [[0, 1], [0, 2], [1, 3], [2, 3], [3, 4]]
.
2
/ \
0 3
\ /
1 4
User | Max Common Friends With | Recommendation |
---|---|---|
0 | 3 (1, 2) | 3 |
1 | 2 (0, 3) | 2 |
2 | 1 (0, 3) | 1 |
3 | 0 (1, 2) | 0 |
4 | 2 (3), 1 (3) | 1 (minimum index) |
Hence the answer returned is [3, 2, 1, 0, 1]
.
Function Description
Complete the function getRecommendedFriends
in the editor below.
getRecommendedFriends
has the following parameters:
int n
: the number of usersint friendships[m][2]
: the friendships between the users
Returns
int[]
: the recommended friends for each user
Constraints
1 ≤ n ≤ 10⁵
0 ≤ m ≤ 2.5 × 10⁵
0 ≤ friendships[i][0], friendships[i][1] < n
- There are no self-loops or multiple edges.
- Each user has a maximum of 15 friends.
- The network of friends might be disconnected.
以下是题目的排版:
Problem: Bitwise-OR Goodness
A coding competition organized to hire software engineers includes an interesting problem on Bitwise-OR.
The goodness of a sequence is defined as the bitwise-OR of its elements. Given an array arr
of length n
, find all possible distinct values of goodness that can be obtained by choosing any strictly increasing subsequence of the array. Sort the returned array in non-decreasing order.
Note:
A subsequence is a sequence that can be derived from the given sequence by deleting zero or more elements without changing the order of the remaining elements.
Function Description
Complete the function getGoodnessValue
in the editor.
Function Parameters:
int arr[n]
: an array of integers
Returns:
int[]
: all possible distinct values of goodness
Examples
Example 1
Input:arr = [4, 2, 4, 1]
Output:[0, 1, 2, 4, 6]
Explanation:
The strictly increasing subsequences which can be chosen to have distinct goodness values are:
- Empty subsequence; goodness = 0
[1]
; goodness = 1[2]
; goodness = 2[4]
; goodness = 4[2, 4]
; goodness = 6
There are no other strictly increasing subsequences that yield a different goodness value. So, the answer is [0, 1, 2, 4, 6]
, which is sorted in non-decreasing order.
Example 2
Input:arr = [3, 2, 4, 6]
Output:[0, 2, 3, 4, 6, 7]
Explanation:
The strictly increasing subsequences which can be chosen to have distinct goodness values are:
- Empty subsequence; goodness = 0
[2]
; goodness = 2[3]
; goodness = 3[4]
; goodness = 4[6]
; goodness = 6[3, 4]
; goodness = 7
Example 3
Input:arr = [3, 5, 5, 1]
Output:[0, 1, 3, 5, 7]
Explanation:
Given n = 4
, arr = [3, 5, 5, 1]
Strictly increasing subsequences that have distinct goodness values are:
- Empty subsequence; goodness = 0
[1]
; goodness = 1[3]
; goodness = 3[5]
; goodness = 5[3, 5]
; goodness = 7
Constraints
1 ≤ n ≤ 10⁴
1 ≤ arr[i] < 1024
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
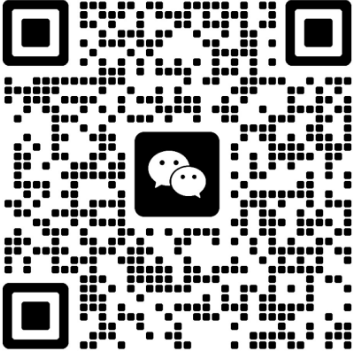