Code Question 1
Amazon stores its data on different servers at different locations. From time to time, due to various factors, Amazon needs to move its data from one location to another. This challenge involves keeping track of the locations of Amazon's data and reporting them at the end of the year.
Problem Statement
At the start of the year, Amazon's data was located at n
different locations. Over the course of the year, Amazon's data was moved from one server to another m
times. Specifically, in the iᵗʰ
operation, the data was moved from movedFrom[i]
to movedTo[i]
.
Find the locations of the data after all m
moving operations. Return the locations in ascending order.
Constraints
- It is guaranteed that for any movement of data:
- There is data at
movedFrom[i]
. - There is no data at
movedTo[i]
.
- There is data at
Example
Input:
locations = [1, 7, 6, 8]
movedFrom = [1, 7, 2]
movedTo = [2, 9, 5]
Output:
[5, 6, 8, 9]
Explanation:
- Initially, data is present at locations [1, 7, 6, 8].
- Move 1: Data moves from 1 → 2.
- Move 2: Data moves from 7 → 9.
- Move 3: Data moves from 2 → 5.
- Final locations are [5, 6, 8, 9] in ascending order.
Function Description
Complete the function getFinalLocations
in the editor below.
Function Signature:
def getFinalLocations(locations: List[int], movedFrom: List[int], movedTo: List[int]) -> List[int]:
Parameters:
locations (List[int])
: The initial locations of the data.movedFrom (List[int])
: The locations data was moved from.movedTo (List[int])
: The locations data was moved to.
Returns:
List[int]
: The locations of data in ascending order after all moves.
Code Question 2
You are part of Amazon's Cloud Infrastructure Team, working on optimizing the data flow through its network of storage servers.
Problem Statement
You are given n
storage servers, and the throughput capacity of each server is represented by an integer array throughput
. There are pipelineCount
data pipelines, each requiring a connection to two servers: one as the primary and the other as the backup.
The transfer rate for a data pipeline is the sum of the throughput of its primary and backup servers. The goal is to maximize the total transfer rate by optimally selecting unique pairs of connections for each pipeline.
Constraints
- A pair of servers
(x, y)
is unique if no other pipeline selects the same pair. However,(y, x)
and(x, y)
are treated as different pairs. - It is valid to use the same server for both primary and backup connections, i.e.,
(x, x)
is a valid pair.
Example
Input:
throughput = [4, 2, 5]
pipelineCount = 4
Output:
36
Explanation:
Possible pairs (1-based indexing):
[1, 1], [1, 2], [1, 3], [2, 2], [2, 3], [3, 1], [3, 2], [3, 3]
Optimal pairs:
- [3, 3] → Transfer Rate = 5 + 5
- [1, 3] → Transfer Rate = 5 + 4
- [3, 1] → Transfer Rate = 4 + 5
- [1, 1] → Transfer Rate = 4 + 4
Total = (5+5) + (5+4) + (4+5) + (4+4) = 36.
Function Description
Complete the function maxTransferRate
in the editor below.
Function Signature:
def maxTransferRate(throughput: List[int], pipelineCount: int) -> int:
Parameters:
throughput (List[int])
: The throughput of each server.pipelineCount (int)
: The number of pipelines requiring connections.
Returns:
int
: The maximum total transfer rate.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
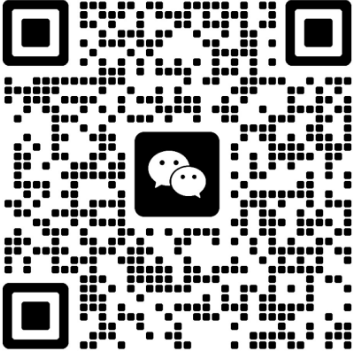