Problem 1: Product Data Management
Design a prototype data structure to efficiently manage the product data of an e-commerce website. Products are categorized by the product name, price, and manufacturing year, given as a 2D array of size n x 3
, where products[i]
represents the three parameters of a product: <name>
, <price>
, <year of manufacture>
.
There are q
queries in a 2D array of size q x 2
of the format <type> <param>
of the following three types:
- Type 1: Find the names of the products manufactured in the given year.
- Type 2: Find the names of the products below the price in their original order in
products
. - Type 3: Find the names of the products above the price in their original order in
products
.
Given products
and queries
, for each query, report an array of names of the corresponding products.
Note:
- For consistency, all data is given as strings. Convert them to integers as necessary.
Example:
Suppose n = 5
,products = [["key", "50", "2013"], ["fan", "100", "2012"], ["lock", "150", "2013"], ["table", "200", "2011"], ["toy", "500", "2011"]]
q = 4
andqueries = [["Type1", "2013"], ["Type2", "500"], ["Type3", "500"], ["Type1", "2011"]]
Output:
Query | Explanation | Answer |
---|---|---|
Type1, "2013" | Products manufactured in 2013 | ["key", "lock"] |
Type2, "500" | Products below price 500 | ["key", "fan", "lock", "table"] |
Type3, "500" | Products above price 500 | [] |
Type1, "2011" | Products manufactured in 2011 | ["table", "toy"] |
Problem 2: Compressing Array
Problem Statement:
Given an array of integers, a
, in one operation, you can select any two adjacent elements and replace them with their product. This operation can only be applied if the product of those adjacent elements is less than or equal to k
.
The goal is to reduce the length of the array as much as possible by performing any number of operations. Return that minimum size.
Example:
Let array a = [2, 3, 3, 7, 3, 5]
and k = 20
.
This is the list of operations that will give us the smallest array (1-based indexing):
- Merge the elements at indices
(1, 2)
, resulting array will be[6, 3, 7, 3, 5]
- Merge the elements at indices
(1, 2)
, resulting array will be[18, 7, 3, 5]
- Merge the elements at indices
(3, 4)
, resulting array will be[18, 7, 15]
Hence, the answer is 3
.
Function Description:
Complete the function getMinLength
in the editor below.
Function Signature:
def getMinLength(a: List[int], k: int) -> int:
Parameters:
int a[n]
: An array of integersint k
: The constraint of the operation
Returns:
int
: The minimum length of the array after performing any number of operations.
Problem 3: SQL - Virtual Machine Deployment Report
Problem Statement:
While working on a new dashboard for a large cloud hosting company, an engineer needs a query that returns a list of CVM (Cloud Virtual Machine) configurations and their total number of deployments in 2021.
The result should have the following columns:
configuration
: Configuration namedeployments
: The total number of all deployments for a specific configuration
Notes:
- Only deployments in 2021 should be included in the report.
- The result should be sorted in descending order by
deployments
.
Schema:
Configurations Table:
Column | Type | Description |
---|---|---|
id | VARCHAR(64) | Unique ID, Primary Key |
name | VARCHAR(255) | Configuration name |
Deployments Table:
Column | Type | Description |
---|---|---|
configuration_id | VARCHAR(64) | Foreign Key to configurations.id |
date | DATE | Deployment date |
SQL Query:
To generate the desired report:
SELECT
c.name AS configuration,
COUNT(d.configuration_id) AS deployments
FROM
configurations c
JOIN
deployments d
ON
c.id = d.configuration_id
WHERE
YEAR(d.date) = 2021
GROUP BY
c.name
ORDER BY
deployments DESC;
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
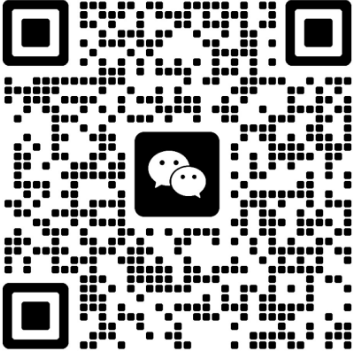