Problem Statement (Original Question)
Given a list of student test scores, find the best average grade.
- Each student may have more than one test score in the list.
- Complete the
bestAverageGrade
function in the editor below.- It has one parameter,
scores
, which is an array of student test scores.- Each element in the array is a two-element array of the form
[student name, test score]
.- Test scores may be positive or negative integers.
- If you end up with an average grade that is not an integer, you should:
- Use a floor function to return the largest integer less than or equal to the average.
- Return
0
for an empty input.Example:
Input:
[["Bobby", "87"],
["Charles", "100"],
["Eric", "64"],
["Charles", "22"]]
Expected Output:
87
Explanation: The average scores are 87, 61, and 64 for Bobby, Charles, and Eric, respectively. The best average is 87.
CSOAHelp's Comprehensive Support
In this Amazon online assessment, the candidate faced a challenge that required calculating the best average grade among multiple students based on their test scores. With CSOAHelp's assistance, the candidate was able to confidently complete the task and effectively explain their approach during the interview. Here's a detailed breakdown of the problem, solution, and how CSOAHelp guided the candidate to success.
Step 1: Clarification Questions
Before diving into the problem, the candidate was guided by us to ask crucial clarifying questions to ensure a complete understanding of the requirements:
- Input Format:
- "Can we assume that all inputs are valid, consisting of student names and scores?"
- "What should the output be if the input is an empty array?"
- Score Range:
- "Are the scores guaranteed to be within a reasonable range, e.g., between -100 and 100?"
- Output Details:
- "Should non-integer average scores be floored using the
math.floor
function?" - "If multiple students have the same best average, is there any specific way to handle it?"
- "Should non-integer average scores be floored using the
- Performance Constraints:
- "Is an O(n) solution sufficient, or are there stricter performance requirements?"
These questions helped the candidate avoid common misunderstandings and define the problem scope accurately.
Step 2: Algorithm Design and Explanation
We helped the candidate design an efficient algorithm and guided them to articulate the solution step-by-step.
Solution Approach:
- Data Aggregation:
- Use a dictionary (
dict
) to group all scores for each student by their names. This allows O(1) insertion and retrieval for each student.
- Use a dictionary (
- Calculate Averages:
- Iterate through the dictionary to compute each student’s average score. Use the
math.floor()
function to round down non-integer averages.
- Iterate through the dictionary to compute each student’s average score. Use the
- Handle Edge Cases:
- If the input is empty, return 0 immediately.
- Properly handle negative scores.
- Ensure the algorithm accommodates varying numbers of scores per student.
Complexity Analysis:
- Time Complexity: O(n), where n is the total number of scores. The dictionary construction and iteration are both linear.
- Space Complexity: O(k), where k is the number of unique students, as we store scores for each student in a dictionary.
Step 3: Example Walkthrough
Input:
scores = [["Bobby", "87"],
["Charles", "100"],
["Eric", "64"],
["Charles", "22"]]
Execution Steps:
- Build the Dictionary:
- Start with an empty dictionary:
student_scores = {}
. - Insert scores into the dictionary:
{ "Bobby": [87], "Charles": [100, 22], "Eric": [64] }
- Start with an empty dictionary:
- Calculate Averages:
- For each student:
- Bobby:
floor(87) = 87
- Charles:
floor((100 + 22) / 2) = 61
- Eric:
floor(64) = 64
- Bobby:
- Identify the highest average:
87
.
- For each student:
- Output:
- Return the highest average:
87
.
- Return the highest average:
Step 4: Handling Interview Follow-Ups
Anticipated Questions:
- Edge Cases:
- "What happens if a student has no scores?"
- "How do you handle all negative scores?"
- Performance Optimization:
- "Can you optimize further for a large dataset?"
- "How can the solution handle a streaming input efficiently?"
- Extending Functionality:
- "What changes would you make to return all students with the best average?"
We prepared the candidate with clear, concise answers to handle such follow-ups confidently.
Step 5: CSOAHelp's Contributions
Here’s how CSOAHelp supported the candidate throughout the process:
- Code Implementation:
- We provided a clean and efficient implementation with detailed inline comments to help the candidate fully grasp the solution.
- Optimization Suggestions:
- Highlighted potential areas for improvement, such as reducing memory usage for extremely large inputs.
- Mock Interview Practice:
- Conducted multiple mock sessions simulating real interview scenarios, focusing on articulating the algorithm and handling edge cases.
- Confidence Building:
- Encouraged the candidate to approach each question methodically, ensuring they explained the "why" behind every step.
Conclusion
With CSOAHelp’s guidance, the candidate not only completed the problem efficiently but also demonstrated excellent communication skills during the interview. This collaboration is another example of how CSOAHelp empowers candidates to excel in high-stakes interviews by providing tailored support and practical solutions.
如果您也想在面试中脱颖而出,欢迎联系我们。CSOAHelp 提供全面的面试辅导与代面服务,帮助您成功拿到梦寐以求的 Offer!
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
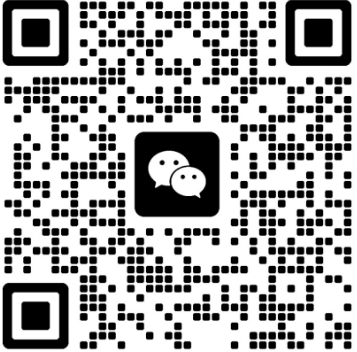