Bank System API Design
Level 1: Basic Account Operations
def createAccount(self, timestamp, accountId):
def deposit(self, timestamp, accountId, amount):
def transfer(self, timestamp, source, target, amount):
For these operations, use a simple hashmap:
accounts = { accountId: amount }
This structure handles account creation, deposits, and transfers with minimal logic.
Level 2: Top Spenders Tracking
def topSpenders(self, timestamp, n):
Introduce an additional hashmap to track total spend per account:
spending = { accountId: total_spent_amount }
Use this data to retrieve the top n
spenders efficiently.
Level 3: Scheduled Payments
def schedulePayment(self, timestamp, accountId, amount, delay):
def cancelPayment(self, timestamp, accountId, paymentId):
Use a min-heap to store scheduled payments by their execution time. Before processing any action (e.g., deposit or transfer), process and execute all due payments from the heap:
import heapq
scheduled = [] # heap of (exec_time, paymentId, accountId, amount)
Level 4: Account Merge and Historical Balance
def mergeAccount(self, timestamp, accountId1, accountId2):
def getBalance(self, timestamp, accountId, timeAt):
Handle various corner cases via careful conditional logic. Merging accounts requires combining balances and resolving identity. For getBalance
, maintain a timestamped transaction log to support time-based queries:
transactions = { accountId: [(timestamp, balance)] }
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
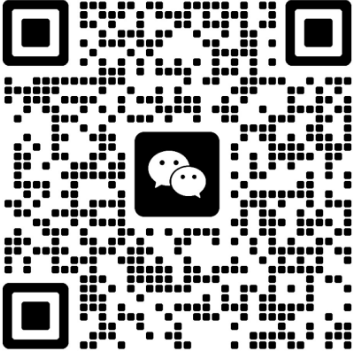