Question 1
TikTok Headline Keyword Detection
Given a list of article headlines and a keyword, which of the following pseudocode snippets correctly uses the Knuth-Morris-Pratt (KMP) algorithm to check if the keyword exists in any headline?
def KMP_Headline_Matching(headlines, keyword):
for headline in headlines:
if KMP(keyword, headline) == True:
return True
return False
def KMP(text, pattern):
lps = computeLPSArray(pattern)
i = 0
j = 0
while i < len(text):
if pattern[j] == text[i]:
i += 1
j += 1
if j == len(pattern):
return True
elif i < len(text) and pattern[j] != text[i]:
if j != 0:
j = lps[j - 1]
else:
i += 1
return False
Question 2
TikTok Comment Moderation
TikTok wants to delete inappropriate comments from a doubly linked list of comments. Write a pseudo-code to delete a target node from the list.
if (target.prev != null) {
target.prev.next = target.next;
}
if (target.next != null) {
target.next.prev = target.prev;
}
target = null;
Question 5
Implementing Load Balancing in a Microservices Architecture
TikTok is adopting a microservices architecture for its applications. Efficient load balancing is critical to its success.
Which load balancing strategy would best distribute requests across microservices?
- Round Robin
- Least Connections
- IP Hash
- Random
Question 6
TikRouter Delivers
Imagine you're in charge of TikTok's servers, where millions of videos, comments, and likes are constantly flowing between users.
TikTok needs to send these small packets of data across its network to keep users' feeds smooth and up-to-date. However, there is a catch! The TikTok delivery drone, which we will call TikRouter, can only carry a limited amount of data on each trip between servers.
- Each "like", comment, or part of a video clip is between 1.01 bytes and 3.00 bytes in size.
- TikRouter is responsible for delivering these packets of interactions to TikTok's users but can only pick up to 3.00 bytes per trip.
Your task is to implement a function that determines the minimum number of trips required to deliver n packets of sizes within the given range.
Example
n = 3
packet_sizes = [1.01, 1.99, 2.5]
TikRouter can deliver all the data packets in two trips: [1.01, 1.99] and [2.5]
Thus, the answer is 2
.
Function Description
Complete the function minimumTripsByTikRouter
in the editor below.
minimumTripsByTikRouter(packet_sizes: List[float]) -> int
Question 7
Interaction Strength on TikTok
TikTok’s algorithm team is developing a feature to improve how videos are recommended to users based on content engagement. The team is analyzing engagement patterns to rank which video combinations create the highest overall user interaction.
Each video has an engagement score. Your goal is to help TikTok determine the k-th highest engagement combination from any possible sequence of videos in the feed.
The engagement score of any sequence is calculated by applying the bitwise OR on the engagement scores of all the videos in that sequence.
Task
Implement a function:
findKthLargestEngagement(n: int, engagement_scores: List[int], k: int) -> int
- Returns the k-th highest interaction strength that can be achieved by combining scores of any sequence of videos from the list.
The interaction strength of a sequence of videos starting from video i
to j
(i ≤ j) is given by:
engagement_scores[i] OR engagement_scores[i+1] OR ... OR engagement_scores[j]
Example
n = 4
engagement_scores = [3, 2, 7, 1]
k = 5
All possible subarrays and their interaction strengths:
- [3] = 3
- [3,2] = 3
- [3,2,7] = 7
- [3,2,7,1] = 7
- [2] = 2
- [2,7] = 7
- [2,7,1] = 7
- [7] = 7
- [7,1] = 7
- [1] = 1
Sorted unique values: 7, 3, 2, 1
k = 5 → not enough distinct values, so return as per rules specified in full prompt (not shown).
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
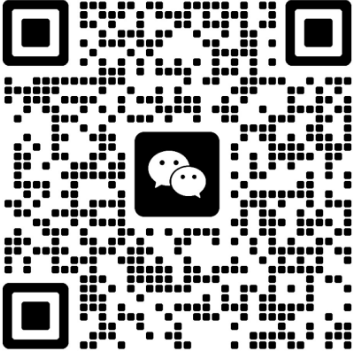