Task 1
Estimate the AUROC value using the trapezoidal rule
Write a function:
def solution(X, Y)
that receives two arguments, X
and Y
(both arrays of size N), such that the i-th point on the ROC curve has coordinates (X[i], Y[i])
, and, using the trapezoidal rule, estimates the AUROC value. The result will be accepted if its absolute error is at most 10⁻⁹.
For example for:
X = [0.00, 0.2, 0.33, 0.43, 0.63, 0.66, 1.00]
Y = [0.00, 0.25, 0.25, 0.50, 0.50, 1.00, 1.00]
your function should return:
0.5575
Assume that:
N
is an integer within the range [2..100]- Each element of arrays
X
andY
is a value within the range [0..1] X
andY
arrays have equal lengthsX
coordinates are distinctX
andY
coordinates are sorted non-decreasingly- Both arrays start with 0 and end with 1
Note: Only standard Python libraries can be used. NumPy and pandas are not available.
Task 2
Determine the winner of each group in a tournament
Write an SQL query that returns a table containing the winner of each group. Each record should contain the ID of the group and the ID of the winner in this group. Records should be ordered by increasing ID number of the group.
Example data
players
player_id | group_id
----------+----------
20 | 2
30 | 1
40 | 3
45 | 1
50 | 2
65 | 1
matches
match_id | first_player | second_player | first_score | second_score
---------+--------------+---------------+-------------+--------------
1 | 30 | 45 | 10 | 12
2 | 20 | 50 | 5 | 5
13 | 65 | 45 | 10 | 10
5 | 30 | 65 | 3 | 15
42 | 45 | 65 | 8 | 4
Your query should return:
group_id | winner_id
---------+-----------
1 | 45
2 | 20
3 | 40
Schema
create table players (
player_id integer not null unique,
group_id integer not null
);
create table matches (
match_id integer not null unique,
first_player integer not null,
second_player integer not null,
first_score integer not null,
second_score integer not null
);
Notes
In group 1, the winner is player 45 with the total score of 30 points.
In group 2, both players scored 5 points, but player 20 has lower ID and is the winner.
In group 3, there is only one player, and although she didn't play any matches, she is a winner.
Assume that:
- Groups are numbered with consecutive integers beginning from 1
- Every player from table
matches
occurs in tableplayers
- In each match, players belong to the same group
Task 3
Remove defective rows from a CSV table
Write a function:
def solution(S)
which, given a string S
of length N
, returns the table without the defective rows in CSV format.
Example 1
S = "id,name,age,score\n1,Jack,NULL,12\n17,Betty,28,11"
Return:
"id,name,age,score\n17,Betty,28,11"
The table with data from string S
looks as follows:
+----+-------+-----+-------+
| id | name | age | score |
+----+-------+-----+-------+
| 1 | Jack | NULL| 12 |
| 17 | Betty | 28 | 11 |
+----+-------+-----+-------+
Example 2
S = "country,population,area\nUK,67m,242000km2"
Return:
"country,population,area\nUK,67m,242000km2"
Explanation: There are no defective rows.
Notes
- The first row is never defective
- A row is defective if it contains at least one cell with the exact value
"NULL"
(case-sensitive) - Lowercase
"null"
is allowed
Assumptions
N
is an integer within the range [1..200000]S
is a string of lengthN
in CSV format- There is at least one row
- Each row has the same, positive number of cells
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
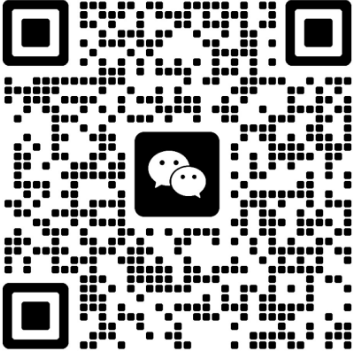