Given Problem
Imagine that you are going fishing at the local pond. The size of the bait must be strictly smaller than the size of the fish for it to catch. Once the fish is caught, it is removed from the pond and cannot be caught again. However, each bait can be used up to 3 times before depletion.
Given two arrays of fish
and baits
, where fish[i]
corresponds to the size of the iᵗʰ fish in the pond, and baits[j]
corresponds to the size of the jᵗʰ bait, your task is to return the maximum number of fish you can catch from the pond with the given baits.
To compute the answer, you need to use each bait to its possible extent, going from the largest bait to the smallest bait. Use each bait to catch the largest fish remaining in the pond and move to the next bait if the current bait was used three times or if it is not strictly smaller than the largest remaining fish. When you run out of baits, return the number of caught fish.
Note: You are not expected to provide the most optimal solution, but a solution with time complexity not worse thanO(max(fish.length, baits.length)³)
will fit within the execution time limit.
Example
Example 1
fish = [1, 2, 3]
baits = [1]
The output should be
solution(fish, baits) = 2
Explanation
Using baits[0] = 1
, it is possible to catch 2 fish fish[2] = 3
and fish[1] = 2
, since 1 < 3
and 1 < 2
. The remaining fish fish[0] = 1
can’t be caught using the given bait.
Example 2
fish = [2, 2, 3, 4]
baits = [1]
Problem Description
Given a string s
consisting of lowercase English letters, find the number of consecutive triplets within s
formed by unique characters. In other words, count the number of indices i
such that s[i]
, s[i + 1]
, and s[i + 2]
are all pairwise distinct.
Note: You are not expected to provide the most optimal solution, but a solution with time complexity not worse thanO(s.length²)
will fit within the execution time limit.
Example
Example 1
s = "abcdaaae"
The output should be
solution(s) = 3
Explanation
- For
i = 0
,s[0] = 'a'
,s[1] = 'b'
, ands[2] = 'c'
, which are pairwise distinct. - For
i = 1
,s[1] = 'b'
,s[2] = 'c'
, ands[3] = 'd'
, which are pairwise distinct. - For
i = 2
,s[2] = 'c'
,s[3] = 'd'
, ands[4] = 'a'
, which are pairwise distinct. - For
i = 3
,s[3] = 'd'
,s[4] = 'a'
, ands[5] = 'a'
, which are not pairwise distinct becauses[4]
ands[5]
are equal. - For
i = 4
,s[4] = 'a'
,s[5] = 'a'
, ands[6] = 'a'
, which are not pairwise distinct.
Example 2
s = "abacaba"
The output should be
solution(s) = 2
Explanation
There are only 2 indices meeting the criteria:
i = 1
:s[1] = 'b'
,s[2] = 'a'
, ands[3] = 'c'
i = 3
:s[3] = 'c'
,s[4] = 'a'
, ands[5] = 'b'
All other triplets will contain more than one 'a'
character.
Input/Output
- [execution time limit] 3 seconds (java)
- [memory limit] 1 GB
- [input] string
s
A string consisting of lowercase English letters.
Guaranteed constraints:
1 ≤ s.length ≤ 10⁵
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
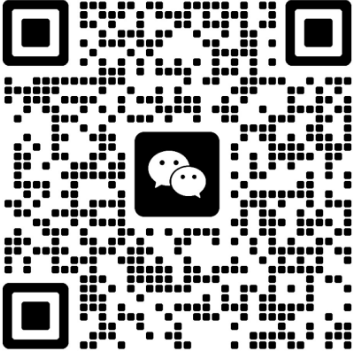