1. String Patterns
Given the length of a word (wordLen) and the maximum number of consecutive vowels that it can contain (maxVowels), determine how many unique words can be generated. Words will consist of English alphabetic letters a through z only. Vowels are V (a, e, i, o, u); consonants are C, the remaining 21 letters. In the explanations, V and C represent vowels and consonants.
Example 1: wordLen = 1, maxVowels = 1
Patterns:{V, C}
That means there are 26 possibilities, one for each letter in the alphabet:
- V (5 choices)
- C (21 choices)
Example 2: wordLen = 4, maxVowels = 1
Patterns:{CCCC, VCCC, CVCC, CCVC, CCCV, VCVC, CVCV, VCVV}
There are 412,776 possibilities—see below:
Example combinations:
C C C C
V C C C
C V C C
C C V C
C C C V
V C V C
C V C V
V C V V
Calculation:
(21 * 21 * 21 * 21) = 194481
(5 * 21 * 21 * 21) + (21 * 5 * 21 * 21) + (21 * 21 * 5 * 21) + (21 * 21 * 21 * 5) = 4 * 46305 = 185220
Thus, the total number of possible words is:
194481 + 185220 = 412,776
2. Vowel Substring
Given a string composed of lowercase letters within the ASCII range 'a'-'z'
, determine the number of substrings that consist solely of vowels, where each vowel appears at least once.
The vowels are { 'a', 'e', 'i', 'o', 'u' }
.
A substring is defined as a contiguous sequence of characters within the string.
Example
Input:s = "aeioexaaeuiou"
Explanation:
There is a substring to the left that is made of vowels, "aeioe"
, which is followed by an 'x'
.
Since 'x'
is not a vowel, it cannot be included in the substring, and this substring does not contain all of the vowels (a, e, i, o, u
).
Thus, "aeioe"
is not a qualifying substring.
Moving to the right, there are four substrings that do qualify:
"aaeuiou"
"aaeui"
"aeuio"
"aeuio"
Function Description
Implement the function vowelSubstring
with the following parameter:
def vowelSubstring(s: str) -> int:
Returns
int
: The number of substrings that consist of only vowels ('a', 'e', 'i', 'o', 'u'
), where every vowel appears at least once.
3. Task Scheduling
The data analysts of Hackerland want to schedule some long-running tasks on remote servers optimally to minimize the cost of running them locally. The analysts have two servers:
- A paid server
- A free server
The free server can be used only if the paid server is occupied.
Each task i is expected to take time[i] units of time to complete, and the cost of processing the task on the paid server is cost[i].
A task can run on the free server only if some task is already running on the paid server.
- The cost of the free server is 0.
- The free server processes any task in exactly 1 unit of time.
Objective
Find the minimum cost to complete all the tasks if they are scheduled optimally.
Example
Input:
n = 4
cost = [1, 1, 3, 4]
time = [3, 1, 2, 3]
Explanation:
- The first task must be scheduled on the paid server for a cost of 1 and takes 3 units of time to complete.
- In the meantime, the other three tasks are executed on the free server for no cost.
- The free server takes only 1 unit to complete each task.
- Total cost:
1
.
Function Description
Complete the function getMinCost
with the following parameters:
def getMinCost(n: int, cost: List[int], time: List[int]) -> int:
Returns
int
: The minimum cost required to complete all tasks.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
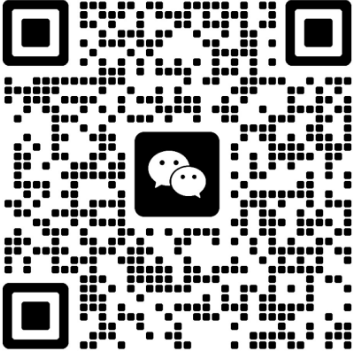