AND is a standard bitwise operation. For example, given κ = 12 (binary representation 01100) and ι = 21 (binary representation 10101) we obtain:
01100 AND
10101 =
-----
00100
The AND operation can be extended to N integers, for example:
01100 AND
10101 AND
00100 =
-----
00100
Because AND of 01100 (first argument) and 10101 (second argument) is 00100, and AND of this number with 00100 (third argument) is also 00100.
Write a function
class Solution {
public int solution(int[] A);
}
that, given an array A consisting of N integers, returns the size of the largest possible subset of A such that AND of all its elements is greater than 0.
You are given an array A of N integers, representing the maximum heights of N skyscrapers to be built.
Your task is to specify the actual heights of the skyscrapers, given that:
- The height of the K-th skyscraper should be positive and not bigger than A[K];
- No two skyscrapers should be of the same height;
- The total sum of the skyscrapers' heights should be the maximum possible.
Write a function:
class Solution {
public int[] solution(int[] A);
}
that, given an array A of N integers, returns an array B of N integers where B[K] is the assigned height of the K-th skyscraper satisfying the above conditions.
If there are several possible answers, the function may return any of them. You may assume that it is always possible to build all skyscrapers while fulfilling all the requirements.
Examples:
- Given A = [1, 2, 3], your function should return [1, 2, 3], as all of the skyscrapers may be built to their maximum height.
- Given A = [9, 4, 3, 7, 7], your function may return [9, 4, 3, 7, 6]. Note that [9, 4, 3, 6, 7] is also a valid answer. It is not possible for the last two skyscrapers to have the same height. The height of one of them should be 7 and the other should be 6.
- Given A = [2, 5, 4, 5, 5], your function should return [1, 2, 3, 4, 5].
Write an efficient algorithm for the following assumptions:
- N is an integer within the range 1 ≤ N ≤ 50,000.
- Each element of A is an integer within the range 1 ≤ A[K] ≤ 50,000.
You are given a positive integer N. Your task is to find the smallest integer greater than N that does not contain two identical consecutive digits.
For example, given N = 1765, the smallest integer greater than N is 1766. However, in 1766 the last two digits are identical. The next integer, 1767, does not contain two identical consecutive digits, and is the smallest integer greater than 1765 that fulfills the condition. Note that the second and fourth digits in 1767 can both be 7 as they are not consecutive.
Write a function:
class Solution {
public int solution(int N);
}
that, given an integer N, returns the smallest integer greater than N that does not contain two identical consecutive digits.
Examples:
- Given N = 55, the function should return 56. It is the smallest integer greater than 55 and it does not contain two consecutive digits that are the same.
- Given N = 1765, the function should return 1767, as explained above.
- Given N = 98, the answer should be 101. Both 99 and 100 contain two identical consecutive digits, but 101 does not.
- Given N = 44432, the answer should be 45010.
- Given N = 3298, the answer should be 3401.
Write an efficient algorithm for the following assumptions:
- N is a positive integer within a reasonable range.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
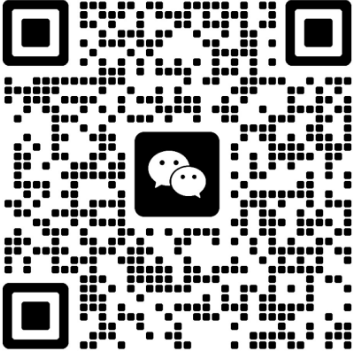