- Find First Duplicate in Array
You are given an array of integers. Your task is to find the first duplicate element in the array. A duplicate element is defined as an element that appears more than once in the array. If there are no duplicates, return null. Choose the code snippet that best solves this problem in terms of time complexity.
Pick ONE option
function findFirstDuplicate(arr) {
sort(arr)
for i from 1 to length(arr) - 1:
if arr[i - 1] == arr[i]:
return arr[i]
return null
}
function count(arr, target) {
count = 0
for each element in arr:
if element == target:
count = count + 1
return count
}
function findFirstDuplicate(arr) {
for each element in arr:
if count(arr, element) > 1:
return element
return null
}
function findFirstDuplicate(arr) {
hashmap = empty hashmap // Assume operations (insert, lookup) are O(1)
for each element in arr:
if element is in hashmap:
return element
insert element into hashmap with value true
return null
}
function findFirstDuplicate(arr) {
funshmap = empty hashmap // Assume operations (insert, lookup) are O(1)
for each element in arr:
if element in hashmap:
return hashmap[element]
else:
hashmap[element] = 1
return null
}
- Checking Sorted Order
Which of the following implementations correctly checks if an array arr
is sorted in ascending order while providing the best time and space complexity?
Pick ONE option
function isSorted(arr) {
if length of arr == 1 or length of arr == 0 {
return true
}
if arr[0] > arr[1] {
return false
}
result = [] of size length of arr - 1
for i = 1 to length of arr - 1 {
result[i - 1] = arr[i]
}
return isSorted(result)
}
function isSorted(arr) {
for i = 1 to length of arr - 1 {
if arr[i - 1] > arr[i] {
return false
}
}
return true
}
Write a function isPrime
that checks if a number num
is prime. Select the correct implementation.
function isPrime(num) {
if (num <= 1) {
return false;
}
for (i = 2; i < num; i++) {
if <code> {
return false;
}
}
return true;
}
Pick ONE option
if (num % i == 0)
if (num == 1)
if (num / i == 0)
Write a function fibonacci
that returns the Fibonacci number at a specific index n
. Choose the correct implementation.
function fibonacci(n) {
if (n <= 1) {
return n;
}
return fibonacci(n - 1) + <code>;
}
Pick ONE option
fibonacci(n - 2)
fibonacci(n)
n + fibonacci(n - 2)
n - fibonacci(n - 1)
Write a function countUnique
that counts the number of unique elements in an array arr
. Choose the correct implementation.
function countUnique(arr) {
var uniqueCount = 0;
var seen = {};
for (num in arr) {
if <code> {
uniqueCount += 1;
seen[num] = true;
}
}
return uniqueCount;
}
Pick ONE option
if (!seen[num])
if (num in seen)
if (seen[num] == 1)
if (num == count)
- Adding Connections
In a TikTok-like social media platform, users are either verified or unverified. Unverified users cannot interact with each other in any way — neither directly nor indirectly. This means that even if there is a chain of interactions connecting unverified users through verified users, such connections must be avoided.
You are given a graphical representation of the platform. There are total of user_nodes number of users their existing interactions are given in the form of two arrays, user_from and user_to, both of size m. This signifies that there is a connection between the users user_from[j] and user_to[j] for all 1 ≤ j ≤ m.
You are also given an array of unverified users, unverified. Find out the max number of new connections that can be added while ensuring that unverified users remain isolated from each other.
Note:
- The connections are bidirectional. ie. If user A has a connection to user B, user B also has a connection to user A.
- A user cannot have a connection to itself.
- There can be at most one connection between a given pair of users.
Example
Suppose user_nodes = 4, unverified = [1, 3],
user_from = [1], user_to = [2]
The optimal solution is to connect user 4 to users 1 and 2. This adds a total of 2 new connections. No more connections can be added as users 1 and 3 cannot have a path between them.
Hence, the answer is 2.
Function Description
Complete the function maxNewConnections in the editor below.
maxNewConnections has the following parameters:
- int user_nodes: the number of users
- int user_from[m]: the users at one end of each connection, m.
- int user_to[m]: the users at the other end of each connection.
- int unverified[k]: the users that are unverified.
Returns
- int: the maximum number of edges that can be added to the graph
Constraints
- 1 ≤ user_nodes ≤ 10⁵
- 0 ≤ m ≤ 10⁵
It is guaranteed that the graph does not have any two path connecting unverified users directly or indirectly.
Input Format For Custom Testing
Sample Case 0
Sample Input For Custom Testing
STDIN | FUNCTION |
---|---|
6 4 | user_nodes = 6, m = 4 |
1 1 2 4 | user_from = [1, 1, 2, 4] |
2 3 3 5 | user_to = [2, 3, 3, 5] |
2 | unverified size k = 2 |
2 4 | unverified = [2, 4] |
Sample Output
3
Explanation
3 new connections can be made. User 6 can be connected to Users 1, 2, and 3.
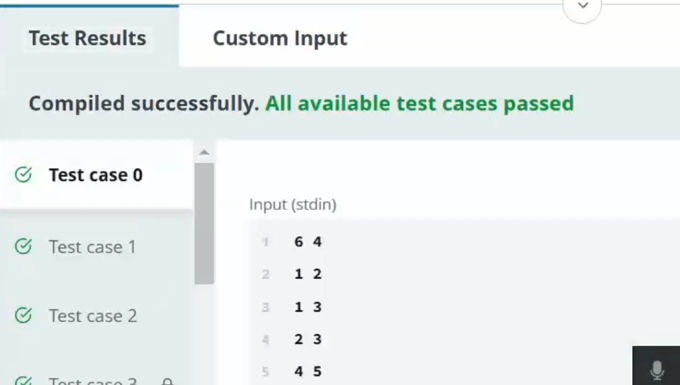
- JavaScript/TypeScript: Tracking Post Engagement by Region (Likes and Shares)
ByteDance, the parent company of TikTok, is looking for an efficient solution to analyze user engagement data across various social media posts. Given the massive volume of data from different countries, they developed a custom TaskManager class to handle the processing of this data and generate useful insights. The class analyzes post engagement by tracking the number of likes and shares across different regions, as well as globally, and then generates reports to help the platform understand its user engagement trends.
The TaskManager class works with posts that contain engagement data like the number of likes and shares from different countries. Each post is associated with a unique post ID, and engagement data for each country is logged.
Function Description
Complete the TaskManager class in the editor below. The class should have the following methods:
- addTask(): Adds engagement data (likes and shares) for a specific country to a given post identified by the postId. If the post does not already exist, a new post is created.
- analyzePostLikesAndShares(): Analyzes the engagement data for all posts. It finds the most liked and most shared countries for each post, as well as globally for all posts. It returns an object containing the detailed results for each post and the global engagement statistics.
- listOrder(): Calls analyzePostLikesAndShares to return the analysis results and then formats the output into a readable report. It returns a formatted string with the results for each post, as well as the global most liked and shared countries.
Example:
Input:
3
4
201 USA 300 100
201 UK 250 150
201 Ind 200 200
201 Can 100 50
202 USA 100 120
202 UK 300 180
202 Ind 150 90
203 USA 500 250
203 UK 400 200
203 Ind 300 150
203 Can 200 100
Output:
PostId: 201
Most liked countries in Post 201: USA, Ind
Most shared countries in Post 201: Ind
----------------------------
PostId: 202
Most liked countries in Post 202: UK
Most shared countries in Post 202: UK
----------------------------
PostId: 203
Most liked countries in Post 203: USA
Most shared countries in Post 203: USA
----------------------------
Global Most Liked Countries: USA
Global Most Shared Countries: USA
Returns:
- addTask(): This function does not return any value. It adds engagement data (likes and shares) to an existing post or creates a new post if it doesn’t already exist. The operation is purely side-effect-based.
- analyzePostLikesAndShares(): This function returns an object containing:
- postResults: An array of objects, each representing a post’s engagement analysis. Each object has:
- postId: The ID of the post.
- mostLikedCountries: A string listing the countries with the most likes for that post (comma-separated).
- mostSharedCountries: A string listing the countries with the most shares for that post (comma-separated).
- globalMostLikedCountries: A string listing the countries with the most likes across all posts (comma-separated).
- globalMostSharedCountries: A string listing the countries with the most shares across all posts (comma-separated).
- postResults: An array of objects, each representing a post’s engagement analysis. Each object has:
- listOrder(): This function returns an array of strings, which is a formatted report of the engagement analysis for each post and global statistics. The report includes:
- A summary of each post, showing its ID, most liked countries, and most shared countries.
- A global summary of the most liked and most shared countries across all posts.
Constraints:
- Number of posts, n ≤ 10⁵
- Number of countries, m ≤ 10⁵
- 100 ≤ postId ≤ 999
- Number of likes, likes ≤ 10³
- Number of shares, shares ≤ 10³
Output Format:
- List of countries with the most liked and shared posts
- postId
- Most liked countries in Post postId: list of Countries
- Most shared countries in Post postId: list of Countries
- A dashed line (
----------------------------
) - Global Most Liked Countries: list of Countries
- Global Most Shared Countries: list of Countries
Note:
When two or more countries have the same number of likes or shares, their names are stored in the order they are encountered in the iteration over the object properties.
Input Format For Custom Testing
Sample Case 0
Sample Input:
3
4
123 Ind 500 200
123 UK 120 50
123 Can 10 5
123 USA 90 100
124 Ind 50 150
124 UK 300 80
124 Can 150 30
124 USA 200 150
125 Ind 120 20
125 UK 250 60
125 Can 30 20
125 USA 180 120
Sample Output:
PostId: 123
Most liked countries in Post 123: Ind
Most shared countries in Post 123: Ind
----------------------------
PostId: 124
Most liked countries in Post 124: UK
Most shared countries in Post 124: Ind, USA
----------------------------
PostId: 125
Most liked countries in Post 125: UK
Most shared countries in Post 125: USA
----------------------------
Global Most Liked Countries: Ind, UK
Global Most Shared Countries: Ind, USA
Explanation:
- PostId 123 has "Ind" as the most liked and shared country.
- PostId 124 has the most liked country as "UK", and the most shared countries as "Ind" and "USA".
- PostId 125 has the most liked country as "UK" and the most shared country as "USA".
- The global most liked countries are "Ind" and "UK".
- The global most shared countries are "Ind" and "USA".
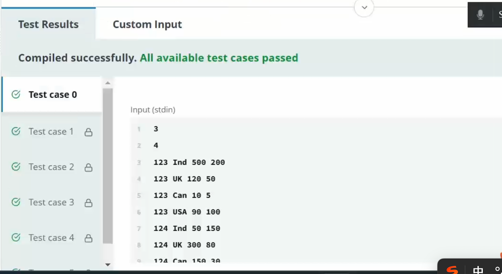
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
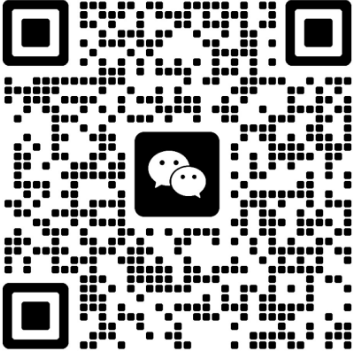