Task 1
that, given two arrays of integers D and C, and an integer P, returns the maximum total number of orders that can be fulfilled.
Examples:
- Given D = [5, 11, 1, 3], C = [6, 1, 3, 2] and P = 7, the function should return 2. The customers at distances 1 and 3 will have their orders fulfilled and 3 + 2 = 5 monitors will be delivered.
- Given D = [10, 15, 1], C = [10, 1, 2] and P = 3, the function should return 1. Only the order for the customer at distance 1 will be fulfilled. There will not be enough monitors in the store for the customer at distance 10. Therefore, orders for customers at distances 10 and 15 will not be fulfilled.
- Given D = [11, 18, 1], C = [9, 18, 8] and P = 7, the function should return 0.
- Given D = [1, 4, 2, 5], C = [4, 9, 2, 3] and P = 19, the function should return 4.
Write an efficient algorithm for the following assumptions:
- N is an integer within the range [1..100,000];
- each element of arrays D and C is an integer within the range [1..1,000,000,000];
- P is an integer within the range [0..1,000,000,000].
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
Task 2
You are given a matrix A representing a chessboard with N rows and M columns. Each square of the chessboard contains an integer representing a points-based score. You have to place two rooks on the chessboard in such a way that they cannot attack each other and the sum of points on their squares is maximal. Rooks in chess can attack each other only if they are in the same row or column.
For example, given a matrix as in the following picture:
0 1
0 1 4
1 2 3
we can place rooks in two different ways:
- One rook on A[0][0] = 1 and another rook on A[1][1] = 3. The sum of points on these squares is 4.
- One rook on A[0][1] = 4 and another rook on A[1][0] = 2. The sum of points on these squares is 6.
Your task is to find the maximum sum of two squares of the chessboard on which the rooks can be placed. In the example above, the answer is 6. We cannot, for example, place the rooks at A[0][1] and A[1][1] (whose sum is 7), as they would attack each other.
Write a function:
Task 3
Let's consider the following infinite sequence:
0, 1, 1, 2, 3, 5, 8, 13, 12, 7, 10, 8, 9, ...
The 0th element is 0 and the 1st element is 1. The successive elements are defined recursively. Each element is the sum of the separate digits of the two previous elements.
Write a function:
def solution(N)
that, given an integer N, returns the N-th element of the above sequence.
Examples:
- Given N = 2, the function should return 1.
- Given N = 6, the function should return 8.
- Given N = 10, the function should return 10.
Write an efficient algorithm for the following assumptions:
- N is an integer within the range [0..1,000,000,000].
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
Task 4
the maximum number of different cars that can be produced in the upcoming month by assigning cars to assembly lines in an optimal way.
Examples:
- Given H = [1, 1, 3], X = 1 and Y = 1, the answer should be 2. Only cars whose assembly time requires 1 hour can be constructed.
- Given H = [6, 5, 5, 4, 3], X = 8, Y = 9, the answer should be 4. The cars that need 3 and 5 hours can be produced on the first assembly line while the second car that needs 5 hours and the car that needs 4 hours can be produced using the second line.
- Given H = [6, 5, 3, 8], X = 8, Y = 8, the answer should be 5. The car that needs 5 hours can be produced on the second line and the four other cars can be produced on the first line.
- Given H = [5, 5, 4, 6], X = 8, Y = 8, the answer should be 2. Only one car can be produced on each line.
Notice that the examples above can have many possible assignments of cars to the assembly lines.
Write an efficient algorithm for the following assumptions:
- N is an integer within the range [1..1,000];
- each element of array H is an integer within the range [1..1,000];
- X and Y are integers within the range [1..500].
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
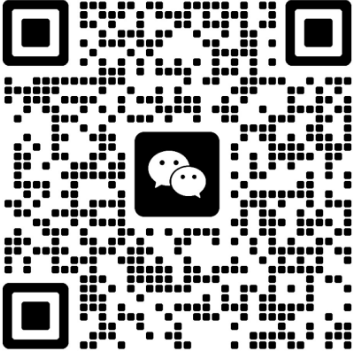