Hewlett Packard Enterprise (HPE) is making headlines again! They just secured a $1 billion contract to provide AI servers for X (formerly Twitter). In this competitive bidding process, HPE outperformed Dell and Supermicro to land this major deal. But what makes HPE's computing power so strong that top companies are willing to invest billions? Their tech talent plays a crucial role. Getting a developer role at HPE isn't easy—their interview process is tough. Let’s take a look at a real HPE interview question.
Problem Description:
Given a non-negative integer a
, compute and return the truncated integer of the square root of a
.
Note: You are not allowed to use any built-in exponent function or operator, such as pow(a, 0.5)
or a ** 0.5
.
Example:
Input: a = 4
Output: 2
Input: a = 8
Output: 2
Explanation: The square root of 8 is 2.82842...
, and since the decimal part is truncated, 2
is returned.
Constraints:
0 <= a <= 2^31 - 1
At first glance, this problem seems simple, but it actually involves the classic binary search algorithm. A brute-force solution would involve iterating from 1
upwards, checking for the largest x
where x * x <= a
, but this approach is inefficient and extremely slow for large numbers.
CSOAHelp provides direct guidance:
- Use binary search to optimize the computation, starting with
left = 0
andright = a / 2 + 1
. - Compute
mid
and check ifmid * mid
is less than or equal toa
. - If
mid * mid <= a
, it might be the answer, so moveleft
to the right; otherwise, moveright
to the left. - The final
right
value is the answer.
Following CSOAHelp’s instructions, the candidate explains to the interviewer: “The integer part of the square root is a monotonically increasing function, so we can use binary search to efficiently find the result. We define the search range, pick the middle value mid
, and check if mid * mid <= a
. If true, we move left
to the right to test larger values; otherwise, we adjust right
to narrow the range. Eventually, the largest mid
satisfying mid * mid <= a
is our answer.”
The interviewer nods in agreement—solid reasoning.
With CSOAHelp’s assistance, the candidate quickly implements the solution:
def sqrt(a):
if a == 0:
return 0
left, right = 1, a // 2 + 1
while left <= right:
mid = (left + right) // 2
if mid * mid == a:
return mid
elif mid * mid < a:
left = mid + 1
else:
right = mid - 1
return right
The candidate explains the code: “We first handle the special case where a = 0
, returning 0
immediately. Then, we define left = 1, right = a // 2 + 1
as the search range. Using binary search, we pick mid
and check if mid * mid == a
. If so, we return mid
; if mid * mid < a
, mid
might be the answer, so we shift left
rightward; otherwise, we shift right
leftward. Finally, right
holds the correct integer square root.”
The interviewer follows up: “Why is binary search better than brute force?”
CSOAHelp provides the explanation: “The brute-force approach has a time complexity of O(sqrt(a))
, while binary search is O(log a)
, making it significantly faster for large numbers.”
The candidate restates: “The brute-force method iterates from 1
to a
, leading to a time complexity of O(sqrt(a))
, which is slow. However, binary search eliminates half the search space at each step, resulting in O(log a)
, making it far more efficient for large values.”
The interviewer nods in approval and adds: “In large-scale computing, algorithm optimization is crucial—binary search is a prime example.”
Throughout the interview, CSOAHelp provides real-time guidance, allowing the candidate to confidently explain the logic, write the code, and answer follow-up questions without hesitation. By the end of the interview, the candidate successfully passes, demonstrating strong algorithmic skills and efficient problem-solving ability.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
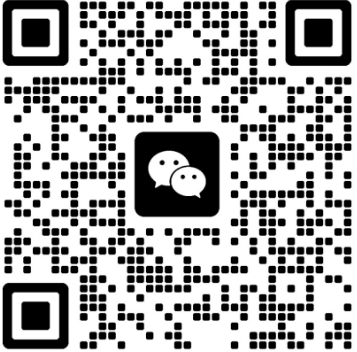