面对Bloomberg这样全球领先的科技公司时,技术面试常常令人感到压力倍增。然而,CSOAHELP通过独特的面试辅助服务,为候选人提供了全程支持,助力他们从容应对复杂的技术挑战。
面试场景还原
此次Bloomberg的面试由两个主要技术问题组成,每个问题都考察了候选人的算法设计能力和代码实现技巧。
问题1: Counting Ships in a Grid
问题描述
"You work on a project that has to implement a new ship discovering technology. You are provided with the function:
struct Point {
const int x_;
const int y_;
Point(int x, int y) : x_(x), y_(y) {}
};
bool hasShips(const Point& bottom_left, const Point& top_right);
// Returns true if there are 1 or more ships within the area with corners bottom_left and top_right
// Returns false if there are no ships within the area
Using the hasShips function, implement the function:
int countShips(const Point& bottom_left, const Point& top_right);
// Returns the number of ships that are within the area with corners bottom_left and top_right
"你正在参与一个开发新型船只探测技术的项目。以下是提供的函数:
struct Point {
const int x_;
const int y_;
Point(int x, int y) : x_(x), y_(y) {}
};
bool hasShips(const Point& bottom_left, const Point& top_right);
需要实现以下函数:
int countShips(const Point& bottom_left, const Point& top_right);
此函数应返回由bottom_left
和top_right
定义的区域内船只的数量。
候选人在面试开始后,首先小心翼翼地向面试官确认题目中hasShips
函数是否已经实现。CSOAHELP的老师迅速为候选人准备了清晰的复述文案:
"在题目中提到已经提供了
struct Point
和hasShips
函数,那么我是否还需要自己实现它们?因为代码中并没有提供。"
候选人复述这一内容后,面试官确认这些函数已经实现,候选人无需重复开发。这个澄清问题不仅显得候选人思路清晰,也帮助其快速进入问题核心。
接下来,候选人需要设计实现countShips
函数的算法。CSOAHELP老师在后台第一时间生成了分治法的代码框架,并提示候选人:
"让我们将网格划分为四个象限,并递归统计每个部分的船只数量。如果某个象限没有船只,则可以跳过进一步的递归。"
候选人根据提示,与面试官描述了解决方案的基本思路:
- 如果当前网格为空(
hasShips
返回false),直接返回0。 - 如果当前网格是一个点(左下角等于右上角),调用
hasShips
返回结果。 - 否则,将网格划分为四个象限,对每个象限递归统计船只数量,并将结果累加。
在实现过程中,候选人遇到了一些困难。例如,当网格被划分为单点时,如何确保代码逻辑正确。此时,老师通过即时文字指导候选人:
"如果区域缩小为一个点,并且
hasShips
返回true,那么直接返回1。"
随着代码逐步完成,候选人开始向面试官解释时间复杂度。为了确保表述专业,CSOAHELP老师提供了详细的复杂度分析:
"递归关系是T(n) = 4T(n/4) + O(1),因此时间复杂度为O(log n),假设
hasShips
的复杂度为O(1)。"
这一回答获得了面试官的高度认可,候选人顺利通过了第一个问题。
完整代码实现框架
**完整代码实现框架**
public int countShips(Point bottom_left, Point top_right) {
// 如果网格内没有船只,返回0
if (!hasShips(bottom_left, top_right)) {
return 0;
}
// 如果网格缩小为一个点
if (bottom_left.x_ == top_right.x_ && bottom_left.y_ == top_right.y_) {
return 1;
}
// 计算中点坐标
int mid_x = (bottom_left.x_ + top_right.x_) / 2;
int mid_y = (bottom_left.y_ + top_right.y_) / 2;
// 分别递归四个象限
return countShips(bottom_left, new Point(mid_x, mid_y)) +
countShips(
new Point(mid_x + 1, bottom_left.y_),
new Point(top_right.x_, mid_y)) +
countShips(
new Point(bottom_left.x_, mid_y + 1),
new Point(mid_x, top_right.y_)) +
countShips(new Point(mid_x + 1, mid_y + 1), top_right);
}
问题2: Word Segmentation
问题描述
"给定一个包含单词的字典和一个不包含空格的输入字符串,编写一个方法返回插入空格后的字符串。"
示例输入
输入: "bloombergisfun", ["bloom", "bloomberg", "is", "fun"]
输出: "bloomberg is fun"
场景细节
面对第二个问题,候选人起初显得有些犹豫。CSOAHELP老师立即通过后台生成了问题解析,并提示候选人逐步解决:
"这个问题的核心是检查如何将输入字符串分割成字典中的单词。我们可以使用回溯法,并通过记忆化技术(memoization)提高效率。"
候选人根据提示,向面试官解释了自己的计划:
- 使用一个递归函数,尝试从输入字符串中提取每一个前缀,检查是否在字典中。
- 如果前缀有效,则递归检查剩余字符串是否可以分割。
- 使用一个备忘录(memoization)存储子问题的结果,避免重复计算。
关键实现步骤
CSOAHELP老师同步为候选人生成了代码框架:
public String wordBreak(String s, List wordDict) {
Set wordSet = new HashSet<>(wordDict);
Map memo = new HashMap<>();
return backtrack(s, wordSet, memo);
}
private String backtrack(String s, Set wordSet, Map memo) {
if (s.isEmpty()) {
return "";
}
if (memo.containsKey(s)) {
return memo.get(s);
}
for (int i = 1; i <= s.length(); i++) {
String prefix = s.substring(0, i);
if (wordSet.contains(prefix)) {
String suffixResult = backtrack(s.substring(i), wordSet, memo);
if (suffixResult != null) {
String result = prefix + (suffixResult.isEmpty() ? "" : " ") + suffixResult;
memo.put(s, result);
return result;
}
}
}
memo.put(s, null);
return null;
}
候选人一边实现代码,一边通过CSOAHELP老师的提示解释各个细节。在运行测试用例时,候选人成功通过所有检查,顺利完成第二个问题。
服务亮点
- 逐字稿记录与实时生成代码 CSOAHELP老师在整个面试过程中,记录了候选人与面试官的所有互动,并根据实时需求为候选人生成完整的代码框架。
- 精准的语言与技术支持 候选人每一次的提问和回答几乎都由老师在后台起草和润色,确保用词精准且表达专业。
- 实时纠错与优化 面试中任何逻辑卡顿或表达不清的环节,老师都能通过实时提示为候选人补充关键信息,确保表现流畅。
总结
通过CSOAHELP的全程支持,候选人在此次Bloomberg面试中充分展现了自己的技术能力和逻辑思维深度。从逻辑设计到代码实现,每一步都在老师的专业支持下完成。这不仅是一场成功的面试,更是团队与候选人共同努力的结果!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
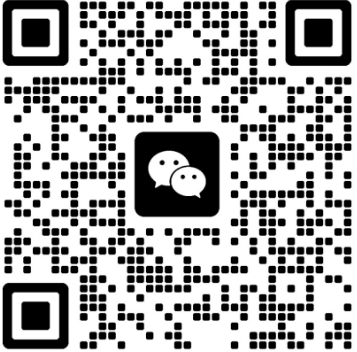