Technical interviews at top tech companies like DoorDash often pose significant challenges. Particularly when an unexpected problem is presented, candidates not only need to remain calm but also demonstrate clear logic and structured thinking within limited time. For this candidate, the situation was even more daunting—he was unprepared and felt momentarily overwhelmed after hearing the problem. Yet, with CSOAHELP’s real-time support, he regained his confidence, successfully completed the interview, and earned the interviewer’s appreciation.
The Interview Question: Finding the Maximum Path Between "Alive Nodes" in a Tree
The problem was stated as follows:
What if the tree we are given can have "alive nodes" at non-leaf nodes as well?
Find the maximum path between any two alive nodes within the tree.
A maximum path may only have the two alive nodes without any other alive nodes in between.
For example, the alive nodes are highlighted with asterisks.
Example tree:
5
/ \
2* 0
/ \ / \
100* 50* 4 15*
The maximum path is: 100 + 2 + 50 = 152
Initial Nervousness and Confusion
When the interviewer finished explaining the problem, the candidate was momentarily at a loss. This question was beyond his preparation, with specific conditions that added complexity:
- The concept of "alive nodes" required unique handling compared to standard path problems.
- Paths must be restricted to two "alive nodes," with no other alive nodes in between.
- The interviewer expected not only a solution but also a clear explanation of time complexity and optimization strategies.
As pressure mounted, CSOAHELP’s real-time guidance became a lifeline, providing crucial direction and helping the candidate refocus.
CSOAHELP’s Real-Time Support: From Confusion to Confidence
While the candidate was still processing the problem, CSOAHELP’s backend team quickly analyzed the question and provided concise keyword hints on the candidate’s secondary screen. These hints served as a guiding light, helping the candidate progressively organize his thoughts.
Breaking Down the Problem: From Complexity to Clarity
When the interviewer reiterated the problem, CSOAHELP’s timely hint appeared:
"Focus on identifying alive nodes and calculating all valid paths between them."
The candidate immediately grasped the crux of the problem and confidently responded:
"I can first identify all the 'alive nodes' and then calculate all valid paths between them to find the maximum sum."
The interviewer acknowledged this approach, allowing the candidate to relax slightly and proceed methodically.
Algorithm Guidance: Core Implementation
As the candidate contemplated implementation, CSOAHELP provided a clear framework:
"Use DFS to traverse the tree, track alive nodes, and calculate path sums."
Following this guidance, the candidate explained:
"I will use Depth-First Search (DFS) to traverse the tree and check each node to determine if it is an 'alive node.' If a path contains only two alive nodes, I will update the maximum path sum."
The interviewer nodded in agreement, encouraging the candidate to continue.
Complexity Analysis: A Clear and Structured Answer
When the interviewer asked about the algorithm’s efficiency, CSOAHELP promptly delivered precise hints:
"Traversal is O(n), where n is the number of nodes; path calculations add constant overhead per node."
The candidate confidently replied:
"The entire tree traversal is O(n) since each node is visited once. Path calculations are done in constant time, so the overall complexity remains linear."
This explanation earned the interviewer’s approval.
Final Code Implementation
With CSOAHELP’s support, the candidate successfully wrote and demonstrated the following code:
class TreeNode:
def __init__(self, val=0, left=None, right=None, alive=False):
self.val = val
self.left = left
self.right = right
self.alive = alive
def maxAlivePath(root):
max_path = float('-inf')
def dfs(node):
nonlocal max_path
if not node:
return 0
left_sum = dfs(node.left)
right_sum = dfs(node.right)
if node.alive:
if node.left and node.left.alive:
max_path = max(max_path, left_sum + node.val)
if node.right and node.right.alive:
max_path = max(max_path, right_sum + node.val)
return node.val
return node.val + max(left_sum, right_sum)
dfs(root)
return max_path
Code Explanation
- Depth-First Search is used to traverse the entire tree.
- At each node, it checks whether the node is "alive" and calculates path sums, updating the maximum as necessary.
- The global variable
max_path
stores the highest path sum found.
Turning the Interview Around
After completing the code, the candidate confidently presented the results. When the code successfully ran and produced the correct output, the interviewer’s initially serious demeanor softened into a smile.
The interviewer said, "Your algorithm is well thought out, and the code is efficient. Can you elaborate on why you chose DFS?"
At that moment, CSOAHELP’s suggestion appeared: "Highlight DFS efficiency and suitability for tree structures." The candidate smoothly replied:
"DFS is ideal for traversing tree structures as it allows us to calculate subtree path sums effectively. When encountering alive nodes, we can directly update the maximum path sum, making the algorithm both simple and efficient."
The interviewer continued, "If the tree is very large, say with millions of nodes, can this algorithm still perform efficiently?"
CSOAHELP instantly provided guidance: "Mention time and space complexity, emphasizing linear traversal and recursive stack space." The candidate answered:
"Yes, the algorithm will perform efficiently. With a time complexity of O(n), where n is the number of nodes, each node is visited only once. Space complexity is primarily determined by the recursion stack, which in the worst case is O(h), where h is the tree’s height."
Satisfied, the interviewer asked, "What if we wanted to allow paths to include at most one non-alive node? How would you adjust your algorithm?"
The candidate paused briefly, then replied:
"In this case, we could introduce a flag during recursion to indicate whether a non-alive node has been included in the path. If not, we allow adding one; if already included, we skip that path." CSOAHELP’s timely hint, "Simplify state transitions for path tracking," made the response more precise.
The interviewer smiled, "Good, it seems you’ve thought about potential extensions. Can you summarize the time and space complexity of your code?"
The candidate concluded:
"The time complexity is O(n) since each node is processed once. Space complexity is O(h), where h is the height of the tree, mainly due to the recursion stack. For balanced trees, it reduces to O(log n)."
Behavioral Question Discussion
The interviewer asked, "Can you share a time when you faced a complex problem and how you solved it under pressure?"
The candidate reflected and answered:
"In a tight project deadline, my team encountered performance bottlenecks with the main service. With only two days until launch, we analyzed the issue, optimized database indexing, and implemented distributed caching, reducing response time by over 50%. This experience taught me the importance of staying calm and systematically breaking down problems."
The interviewer responded with a smile, "That’s impressive. Today’s interview is complete, and your performance has been excellent."
When the candidate closed the meeting software, he exhaled deeply. This interview had tested his composure, adaptability, and analytical skills. With CSOAHELP’s timely support, he overcame initial nervousness, demonstrated technical depth, and showcased his problem-solving abilities. It was not just a test but a significant learning and growth opportunity that left him more confident about his future and his potential.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
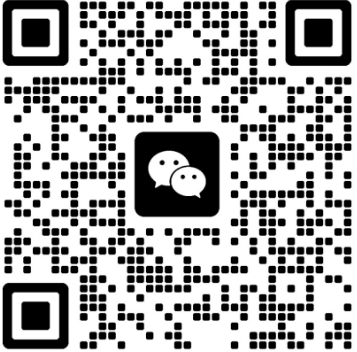