Problem 1: Minimum Days to Deliver Parcels
Problem Statement
Amazon Delivery Centers dispatch parcels every day. There are n
delivery centers, each having parcels[i]
parcels to be delivered.
On each day, an equal number of parcels are to be dispatched from each delivery center that has at least one parcel remaining. Find the minimum number of days needed to deliver all the parcels.
Function Description
Complete the function minDaysToDeliverParcels
in the editor below.
Parameters
int parcels[n]
: The number of parcels at each delivery center.
Returns
int
: The minimum number of days needed to deliver all the parcels.
Constraints
- 1 <= n <= 1,000,000
- 0 <= parcels[i] <= 1,000,000,000
Example
Input
parcels = [2, 3, 4, 3, 3]
Output
3
Explanation
All parcels can be delivered in a minimum of 3 days.
Sample Input for Custom Testing
STDIN
parcels[] size, n = 6
parcels = [3, 3, 3, 3, 3, 3]
Sample Output
1
Explanation
Each delivery center can dispatch all its parcels on the first day.
Problem 2: Maximum Sum of Array Difference
Problem Statement
At Amazon, the team at the fulfillment center is responsible for the packaging process. There is an array, item_weights
, of n
items to pack.
The team needs to create a new array, new_arr
, by removing exactly n / 3
items from item_weights
without changing the order of the remaining items.
The sum_arr
of array new_arr
is defined as:sum_arr = (sum of the first half of new_arr) - (sum of the second half of new_arr)
.
Given n
items and the array item_weights
, find the maximum sum_arr
possible.
Function Description
Complete the function getMaxSumarr
in the editor below.
Parameters
int item_weights[n]
: Item weights.
Returns
int
: The maximum possiblesum_arr
.
Constraints
- 3 <= n <= 100,000
- -10,000 <= item_weights[i] <= 10,000
- n is divisible by 3.
Example
Input
n = 3
item_weights = [3, 2, 1]
Output
2
Explanation
Array | Removed Element | New Array | sum_arr(new_arr) |
---|---|---|---|
[3, 2, 1] | Index 2 | [3, 1] | 3 - 1 = 2 |
[3, 2, 1] | Index 1 | [2, 1] | 2 - 1 = 1 |
[3, 2, 1] | Index 3 | [3, 2] | 3 - 2 = 1 |
The maximum score possible for sum_arr
is 2.
Sample Input for Custom Testing
STDIN
FUNCTION
n = 6
item_weights[] = [1, 3, 4, 7, 5, 2]
Sample Output
4
Explanation
Given n = 6, item_weights = [1, 3, 4, 7, 5, 2]:
- Remove elements 1, 3 to leave
new_arr = [4, 7, 5, 2]
. - sum_arr = (4 + 7) - (5 + 2) = 4.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
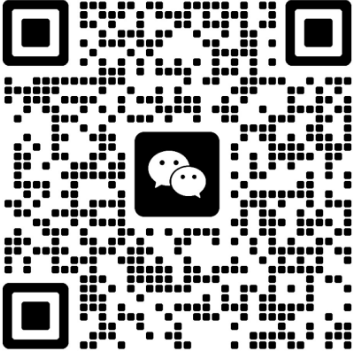