Code Question 1
Amazon developers are creating a new security module for Amazon Web Services. One of its functions is to obfuscate incoming messages. The obfuscation method is to select some substring of the message and right rotate that substring by 1 position one time only. The substring is selected in such a way that the resulting obfuscated message is alphabetically the smallest message that can be formed using this operation.
Here, right rotating any string a by 1 position is to shift every character of string a in the right direction by 1 position and remove the last character and append it to the beginning of string a. For example, a = "ahssd", after right rotation by 1 position string a changes to "dahss".
Given the input message as a string, find the obfuscated message.
Note: A string x is alphabetically smaller than string y, if either x is a prefix of y (and x ≠ y), or there exists such i (0 ≤ i < min(|x|, |y|)), that x[i] < y[i], and for any j (0 ≤ j < i) x[j] = y[j]. Here, |a| denotes the length of the string a.
Example
message = "aahhab"
These are some of the possible strings:
Starting Index | Ending Index | Substring | After Right Rotation |
---|---|---|---|
2 | 5 | hhab | aabhha |
0 | 2 | aah | haahhab |
1 | 2 | ah | ahahhab |
2 | 4 | hha | aaahhb |
Rotating the substring "hha" produces "ahh". Replace "hha" in the original string to get "aaahhb", the alphabetically smallest message that can be formed. Return "aaahhb".
Function Description
Complete the function findObfuscateMessage in the editor below.
findObfuscateMessage has the following parameter:
string message
: the input message
Returns
string
: the encrypted message
Code Question 2
Amazon's database doesn’t support very large numbers, and hence, numbers are stored as a string of binary characters, '0' and '1'. Accidentally, a '!' was entered in some positions, and it is unknown whether they should be '0' or '1'.
The string of incorrect data consists of the characters '0', '1', and '!', where '!' represents an unknown character. The '!' can be replaced with either '0' or '1'. Due to some internal faults, errors are generated every time the characters '0' and '1' appear together as '01' or '10' in any subsequence of the string. It is observed that the number of errors a subsequence '01' generates is xx, while a subsequence '10' generates yy errors.
Determine the minimum total errors generated. Since the answer can be very large, return it modulo 109+710^9 + 7.
Example
errorString = "10!11"
x = 2
y = 3
- If the '!' at index 3 is replaced with '0', the string is "10101". The number of times the subsequence 01 occurs is 3 at indices (1, 2), (1, 4), and (3, 4). The number of times the subsequence 10 also is 3, indices (0, 1), (0, 3), and (2, 3). The number of errors is 3×x+3×y=6+9=153 \times x + 3 \times y = 6 + 9 = 15.
- If the '!' is replaced with '1', the string is "10111". The subsequence 01 occurs 3 times and 10 occurs 1 time. The number of errors is 3×x+1×y=6+3=93 \times x + 1 \times y = 6 + 3 = 9.
The minimum number of errors is min(9,15)mod (109+7)=9\min(9, 15) \mod (10^9 + 7) = 9.
Note: A subsequence of a string is obtained by omitting zero or more characters from the original string without changing their order.
Hint: It can be proved that (a+b)%c=((a%c)+(b%c))%c(a + b) \% c = ((a \% c) + (b \% c)) \% c where a, b, and c are integers and % represents the modulo operation.
Function Description
Complete the function getMinErrors in the editor below.
getMinErrors has the following parameters:
string errorString
: a string of characters '0', '1', and '!'int x
: the number of errors generated for every occurrence of subsequence 01int y
: the number of errors generated for every occurrence of subsequence 10
Returns
int
: the minimum number of errors possible, modulo 109+710^9 + 7
CSOAhelp长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
CSOAhelp consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
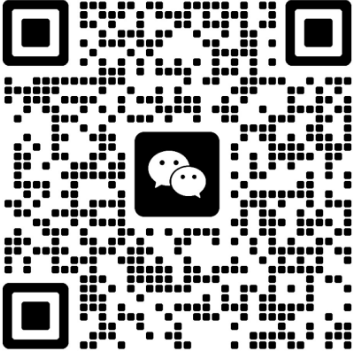