Amazon’s technical interviews are renowned for their emphasis on structured problem-solving, requiring candidates to demonstrate their algorithmic thinking under pressure. One such recent problem was the Parking Garage Simulation, a challenge designed to test data structure manipulation and logical reasoning. With CSOAhelp, we guided a candidate through this intricate problem, ensuring they not only solved it but did so with a deep understanding.
The Problem Statement
I own a parking garage that provides valet parking services.
Customers pulling up to the entrance are either rejected if the garage is full or given a ticket they can use to retrieve their car. The garage layout consists of different parking bays: Small, Medium, and Large. The task was to build a program to manage car arrivals and departures, ensuring that parking bays are efficiently allocated and tracking ticket usage.
Garage Layout:
- 1 Small bay
- 1 Medium bay
- 2 Large bays
The program must handle a sequence of actions involving arrivals and departures. Each action is represented as a tuple:
("arrival", <car_size>)
: A car arrives at the garage. The size can be Small, Medium, or Large.("depart", <ticket_number>)
: A car leaves the garage based on the ticket number.
Example
Input Garage Layout:
[1, 1, 2]
First Sequence Actions:
[("arrival", "small"), ("arrival", "large"), ("arrival", "medium"), ("arrival", "large"), ("arrival", "medium")]
Expected Output:
[1, 2, 3, 4, "reject"]
Second Sequence Actions:
[("arrival", "small"), ("arrival", "large"), ("arrival", "medium"), ("arrival", "large"), ("depart", 3), ("arrival", "medium")]
Expected Output:
[1, 2, 3, 4, 5]
Challenges Candidates Faced
- Managing Parking Bays with Different Sizes: The candidate needed to track parking availability for each bay size and ensure proper allocation rules.
- Handling Departures with Ticket Management: Departures required linking tickets to parking bays, updating availability efficiently.
- Edge Cases: They had to account for rejections when the garage was full and correctly handle ticket-based departures.
CSOAhelp’s Role
At CSOAhelp, we don’t just provide solutions—we empower candidates to think algorithmically. For this problem, we offered:
- Structured Algorithm Design:
- Explained the importance of using a dictionary to map car sizes to bay indices for efficient lookups.
- Suggested maintaining a ticket-to-size mapping for seamless handling of departures.
- Annotated Code Walkthrough:
- Highlighted key functions, like how to handle arrivals and departures.
- Provided edge-case testing scenarios to ensure robustness.
- Interview Simulation:
- Simulated a real Amazon interview environment.
- Challenged the candidate with follow-up questions to evaluate scalability and adaptability.
- Optimized Solutions:
- Introduced an O(1) approach for ticket issuance and bay updates.
- Showcased code refactoring tips for better readability and maintenance.
Key Takeaways from the Solution
Algorithm Outline:
- Use a dictionary to manage parking bays by size:
{"small": 0, "medium": 1, "large": 2}
. - Maintain a counter for tickets and a map to track ticket-to-size associations.
- For each action:
- On arrival: Check availability, allocate a bay, and issue a ticket.
- On departure: Update availability using the ticket-to-size map.
Code Sample:
def assign_parking(garage_layout, sequence):
size_to_idx = {"small": 0, "medium": 1, "large": 2}
result = []
ticket = 1
ticket_to_size = dict()
for req in sequence:
req_type, car_type = req
if req_type == "arrival":
size_idx = size_to_idx[car_type]
if garage_layout[size_idx] > 0:
garage_layout[size_idx] -= 1
result.append(ticket)
ticket_to_size[ticket] = car_type
ticket += 1
else:
result.append("reject")
elif req_type == "depart":
ticket_number = car_type
garage_layout[size_to_idx[ticket_to_size[ticket_number]]] += 1
return result
Conclusion
Amazon’s algorithmic challenges require not just technical knowledge but the ability to think on your feet and adapt to evolving problems. With CSOAhelp, candidates receive comprehensive support—from understanding problem statements to excelling in follow-up questions.
If you’re preparing for your next technical interview, let CSOAhelp be your partner in success! Contact us today for tailored solutions and unparalleled guidance.
This version focuses on showcasing CSOAhelp's value while emphasizing the structured and strategic approach taken to tackle the problem.
如果您也想在面试中脱颖而出,欢迎联系我们。CSOAHelp 提供全面的面试辅导与代面服务,帮助您成功拿到梦寐以求的 Offer!
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
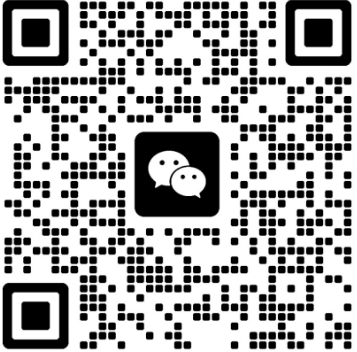