⏱ 110 minutes for 2 tasks
Important Notes from Microsoft
- As professionals, we expect you will not "copy and paste" from other sources or plagiarize your answers from the internet. Codility validates all candidate solutions for similar solutions and will flag those answers directly to Microsoft.
- Attention to detail is key! Make sure to read the questions thoroughly and address ALL of the components.
Task 1
A word is diverse if it does not contain three equal consecutive letters (for example, "aaba" is diverse, but "aaab" is not).
What is the alphabetically smallest diverse word that can be built using exactly A letters "a", B letters "b", and C letters "c"?
A, B, and C are chosen in such a way that it is always possible to build such a word.
Write a function:
def solution(A, B, C):
That, given three integers A, B, and C, returns the alphabetically smallest diverse word that can be built.
Examples
- Given A = 3, B = 1, and C = 0, the function should return:
"aaba" - Given A = 1, B = 4, and C = 4, the function should return:
"abbcbcbcc" - Given A = 1, B = 3, and C = 0, the function should return:
"babb"
Write an efficient algorithm under the following assumptions:
- A, B, and C are integers within the range [0..100,000].
- It is always possible to build a diverse word.
- The length of the answer (A + B + C) is an integer within the range [1..100,000].
Task 2
There are N patients waiting to visit the doctor, and S slots available for appointments. Each patient has two preferred slots (one primary and one secondary).
Your task is to determine whether it is possible to assign all patients to their preferred slots so that no two patients are assigned to the same slot.
Write a function:
def solution(A, B, S):
That, given arrays A and B (each of length N) and an integer S, determines whether it is possible to assign all patients to their preferred slots.
Examples
- Given A = [1, 2, 3], B = [1, 2, 3], and S = 3, the function should return:
True
Example assignment: [1 → Slot 1, 2 → Slot 2, 3 → Slot 3]. - Given A = [3, 2, 3, 1], B = [1, 3, 1, 2], and S = 3, the function should return:
False
There are only three slots available, but there are four patients with overlapping preferences. - Given A = [2, 5, 6, 5], B = [5, 4, 2, 2], and S = 8, the function should return:
True
Example assignment: [5 → Slot 4, 6 → Slot 2, etc.]. - Given A = [1, 2, 1, 6, 8, 7, 8], B = [2, 3, 4, 7, 7, 8, 7], and S = 10, the function should return:
False
Write an efficient algorithm under the following assumptions:
- N is an integer within the range [1..100,000].
- S is an integer within the range [2..100,000].
- Each element of arrays A and B is an integer within the range [1..S].
- No patient has two preferences for the same slot, i.e., A[i] ≠ B[i].
CSOAhelp长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
CSOAhelp consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
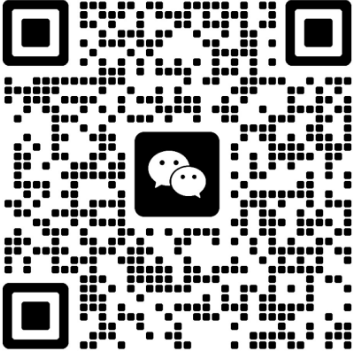