Problem Description
Scenario:
Stripe processes payments for merchants, starting with Authorization Requests, which represent the intent to transfer funds from an end user’s credit card to a merchant. The system processes these requests and determines the Outcome. Currently, the system’s policy is to automatically APPROVE all requests.
Input and Output Details
Input Format:
Authorization requests are received in the following format:
timestamp_seconds,unique_id,amount,card_number,merchant
Field Descriptions:
timestamp_seconds
: The timestamp of the request in seconds (positive integer).unique_id
: A unique identifier for the request.amount
: The authorized amount (decimal value greater than zero).card_number
: The user’s credit card number.merchant
: The name of the merchant.
Input Example:
5,R1,5.60,4242424242424242,bobs_burgers
10,R2,500.00,4242111111111111,a_corp
Output Format:
The output is a human-readable report with the following fields:
timestamp unique_id amount outcome
- timestamp: The time the request was received.
- unique_id: The unique identifier of the request.
- amount: The authorized amount.
- outcome: The result of the request, which in this case is always
APPROVE
.
Output Example:
5 R1 5.60 APPROVE
10 R2 500.00 APPROVE
Solution Breakdown
1. Input Parsing
Objective:
Parse the input data and extract relevant fields into structured data.
Steps:
- Split the input string into lines, where each line represents an authorization request.
- Parse each line by splitting it using commas (
','
). - Map the extracted values to corresponding fields:
timestamp
: Convert to an integer.unique_id
: Retain as a string.amount
: Convert to a floating-point number.card_number
: Retain as a string.merchant
: Retain as a string.
- Store the parsed data as a list of dictionaries for ease of access.
Example Parsing:
Input Line: 5,R1,5.60,4242424242424242,bobs_burgers
Parsed Output:
{
"timestamp": 5,
"unique_id": "R1",
"amount": 5.60,
"card_number": "4242424242424242",
"merchant": "bobs_burgers"
}
2. Sorting the Data
Objective:
Ensure all requests are ordered chronologically by their timestamp
.
Steps:
- Use the
sorted()
function with thetimestamp
field as the sorting key. - Maintain stable sorting for requests with the same timestamp.
Example Sorted Data:
Before Sorting:
[
{"timestamp": 10, "unique_id": "R2", "amount": 500.00, ...},
{"timestamp": 5, "unique_id": "R1", "amount": 5.60, ...}
]
After Sorting:
[
{"timestamp": 5, "unique_id": "R1", "amount": 5.60, ...},
{"timestamp": 10, "unique_id": "R2", "amount": 500.00, ...}
]
3. Formatting the Output
Objective:
Generate a human-readable report with the specified output format.
Steps:
- For each record in the sorted data, create a formatted string using:
"{timestamp} {unique_id} {amount:.2f} APPROVE"
- Ensure
amount
is displayed with two decimal places using{:.2f}
. - Append the formatted strings to a list for the final output.
5 R1 5.60 APPROVE
10 R2 500.00 APPROVE
Complexity Analysis
Time Complexity:
- Input Parsing:
O(n)
, wheren
is the number of input lines. - Sorting:
O(n log n)
, for sorting the parsed data bytimestamp
. - Formatting:
O(n)
, for generating output strings.
Overall Time Complexity: O(n log n)
Space Complexity:
- The parsed data requires
O(n)
space. - Additional space for storing formatted strings is also
O(n)
.
Overall Space Complexity: O(n)
Example Walkthrough
Input:
5,R1,5.60,4242424242424242,bobs_burgers
10,R2,500.00,4242111111111111,a_corp
Steps:
- Parsing the Input:
[ {"timestamp": 5, "unique_id": "R1", "amount": 5.60, ...}, {"timestamp": 10, "unique_id": "R2", "amount": 500.00, ...} ]
- Sorting by Timestamp:
[ {"timestamp": 5, "unique_id": "R1", "amount": 5.60, ...}, {"timestamp": 10, "unique_id": "R2", "amount": 500.00, ...} ]
- Formatting Output:
5 R1 5.60 APPROVE 10 R2 500.00 APPROVE
CSOAHelp’s Role in the Candidate’s Success
During the interview, CSOAHelp provided the following critical support to the candidate:
- Step-by-Step Guidance:
- Helped break down the problem into manageable components: parsing, sorting, and formatting.
- Suggested optimal data structures like dictionaries and lists for efficient data handling.
- Performance Optimization:
- Recommended the use of in-built sorting functions for minimal complexity.
- Advised on maintaining precision in floating-point operations.
- Real-Time Debugging:
- Provided on-the-spot feedback for edge cases like empty input or duplicate timestamps.
Conclusion
This Stripe interview question evaluates a candidate’s ability to process structured data, perform efficient sorting, and generate formatted output. With CSOAHelp’s assistance, the candidate confidently tackled the problem, demonstrating strong problem-solving and coding skills.
If you need interview coaching or real-time assistance, contact CSOAHelp! We offer comprehensive support to help you secure your dream job.
如果您也想在面试中脱颖而出,欢迎联系我们。CSOAHelp 提供全面的面试辅导与代面服务,帮助您成功拿到梦寐以求的 Offer!
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
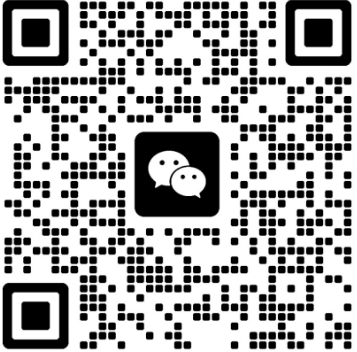