Question 1: Assign Lockers to Boxes
Problem Statement:
Given a set of lockers that can be one of three sizes - s, m, l
and boxes that can be one of three sizes - s, m, l
- Implement the "assignLockerToBox" and "getLockerForBox" methods.
Assignment rule is that you should prefer same sized lockers/boxes,
but if there are no available same sized lockers,
smaller boxes can go in bigger lockers.
Input: []
Solution Analysis and Approach
This problem focuses on efficient matching and prioritization. The goal is to assign lockers to boxes based on their sizes while adhering to specific constraints.
Solution Steps:
- Data Structure Choice:
Use three queues to manage available lockers of sizess
,m
, andl
. This allows efficient retrieval and allocation. - Assignment Logic:
- First, try to assign a locker of the same size as the box.
- If no locker of the same size is available, attempt to assign the next larger size.
- Complexity Analysis:
- Time Complexity:
O(1)
for each allocation, as accessing a queue is constant time. - Space Complexity:
O(n)
, wheren
is the number of lockers.
- Time Complexity:
Example Walkthrough
Input:
Lockers: [s, s, m, l, l]
Boxes: [s, m, l, s, s]
Process:
- Box
s
-> Assign lockers
. - Box
m
-> Assign lockerm
. - Box
l
-> Assign lockerl
. - Box
s
-> Assign another lockers
. - Box
s
-> Assign lockerl
(as no small lockers remain).
Output:
Assignments: [s -> s, m -> m, l -> l, s -> s, s -> l]
Question 2: Find All Duplicates in an Array
Problem Statement:
Given an integer array nums of length n where all
the integers of nums are in the range [1, n] and
each integer appears once or twice,
return an array of all the integers that appears twice.
Solution Analysis and Approach
This problem leverages the array itself as a hash map, using the relationship between numbers and indices to efficiently detect duplicates.
Solution Steps:
- Index Mapping:
For each number in the array, compute its corresponding index (abs(num) - 1
).- If the value at that index is positive, flip it to negative to mark it as visited.
- If it is already negative, the current number is a duplicate.
- Complexity Analysis:
- Time Complexity:
O(n)
since each element is processed once. - Space Complexity:
O(1)
as no additional space is used.
- Time Complexity:
Example Walkthrough
Input:
nums = [4, 3, 2, 7, 8, 2, 3, 1]
Process:
- Start with index
abs(4) - 1 = 3
. Flipnums[3]
to-7
. - Index
abs(3) - 1 = 2
. Flipnums[2]
to-2
. - Index
abs(2) - 1 = 1
. Flipnums[1]
to-3
. - Index
abs(7) - 1 = 6
. Flipnums[6]
to-3
. - Index
abs(8) - 1 = 7
. Flipnums[7]
to-1
. - Index
abs(2) - 1 = 1
.nums[1]
is already negative →2
is a duplicate. - Index
abs(3) - 1 = 2
.nums[2]
is already negative →3
is a duplicate.
Output:
[2, 3]
CSOAHelp’s Role in Candidate Success
During the interview, CSOAHelp provided the following guidance to the candidate:
- Solution Optimization:
Suggested the use of efficient data structures like queues and in-place marking to reduce space and time complexity. - Edge Case Handling:
Emphasized the importance of addressing edge cases, such as empty input arrays or duplicate values. - Real-Time Feedback:
Assisted with breaking down the problem into smaller, manageable steps for better clarity and delivery.
Conclusion
These Amazon interview questions demonstrate the importance of efficient algorithms and real-time problem-solving skills. Thanks to CSOAHelp, the candidate confidently tackled these challenges, showcasing their technical expertise and logical thinking.
如果您也想在面试中脱颖而出,欢迎联系我们。CSOAHelp 提供全面的面试辅导与代面服务,帮助您成功拿到梦寐以求的 Offer!
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
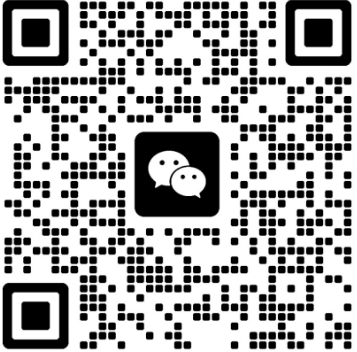