Code Question 1: Server Channel Quality
Amazon's AWS provides fast and efficient server solutions. The developers want to stress-test the quality of the servers' channels. The following conditions must be met:
- Each packet must be sent via a single channel.
- Each channel must transfer at least one packet.
The quality of a transfer for a channel is defined by the median of the sizes of all data packets sent through that channel.
- Note: The median of an array is:
- The middle element if the array is sorted in non-decreasing order.
- If the number of elements is even, the median is the average of the two middle elements.
Task:
Find the maximum possible sum of the qualities of all channels. If the answer is a floating-point value, round it to the next higher integer.
Example:
Input:
packets = [1, 2, 3, 4, 5]
channels = 2
Steps:
- At least one packet must be sent through each of the 2 channels.
- A maximal solution is to transfer packets
{1, 2, 3, 4}
through channel 1 and{5}
through channel 2.
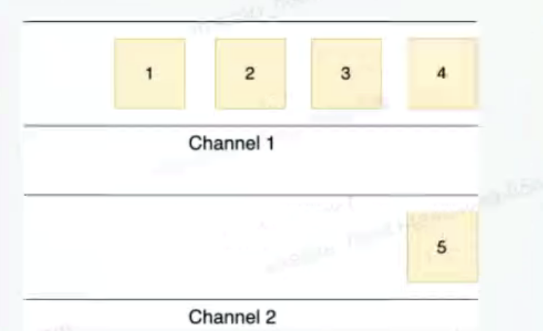
Quality:
- Median of channel 1 =
(2 + 3) / 2 = 2.5
- Median of channel 2 =
5
Sum = 2.5 + 5 = 7.5
, rounded up to 8
.
Output: 8
Function Description:
Complete the function findMaximumQuality
in the editor below.
Parameters:
int packets[n]
: An array of packet sizes.int channels
: The number of channels.
Returns:
long int
: The maximum sum possible.
Constraints:
1 <= len(packets) <= 5 × 10^5
1 <= packets[i] <= 10^9
1 <= channels <= len(packets)
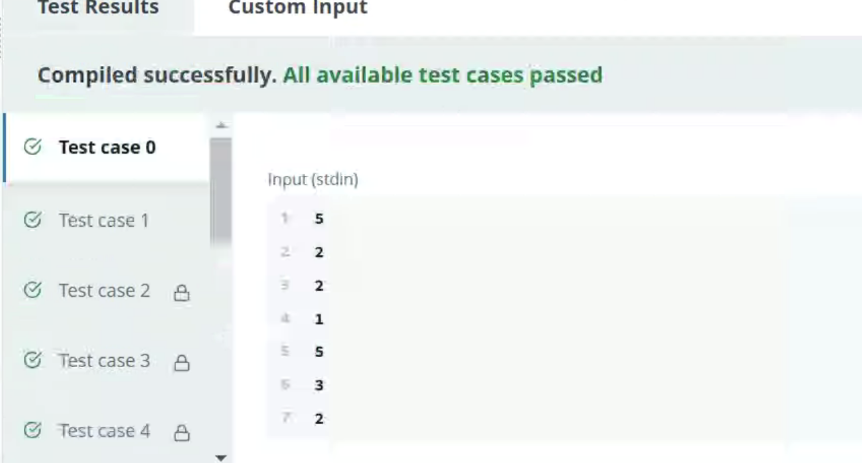
Code Question 2: Lexicographical Array Generator
Your team is tasked with creating an array generator service to assist with software testing.
Input Parameters:
arr[n]
: An array ofn
positive integers.state
: A binary string of lengthn
where:- If
state[i] = '1'
, thenarr[i]
is available. - If
state[i] = '0'
, thenarr[i]
is blocked.
- If
m
: The number of operations to perform.
Task: To create an integer array S
, perform the following operation exactly m
times:
- Choose any
arr[i]
that is available (state[i] = '1'
). - Append the value in
arr[i]
toS
. - For all
state[j] = '0'
such thatstate[j-1] = '1'
, changestate[j]
to1
.
Return the lexicographically largest sequence S
that can be obtained after m
operations.
Example:
Input:
arr = [10, 5, 7, 6]
state = "1010"
m = 2

Steps:
- Start with
state = "1010"
. Available elements:10, 7
. - Choose
10
→ Append toS
. Updatestate = "1110"
. - Choose
7
→ Append toS
.
Resulting S
: [10, 7]
.
Output: [10, 7]
Function Description:
Complete the function generateNewArray
in the editor below.
Parameters:
int arr[n]
: An array of integers.string state
: A binary string indicating availability.int m
: The number of operations.
Returns:
int[m]
: The lexicographically largest sequenceS
.
Constraints:
1 <= n, m <= 10^5
1 <= arr[i] <= 10^9
|state| = n
state[i]
is either'0'
or'1'
.- There is at least one
'1'
instate
initially.
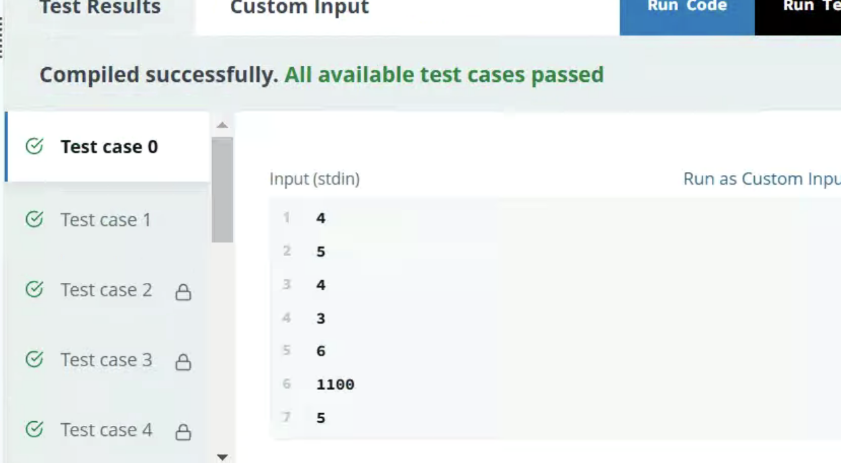
CSOAhelp长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
CSOAhelp consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
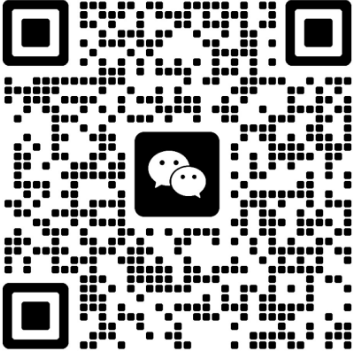