Question 1: Pascal Triangle Encryption
In order to ensure maximum security, Amazon employs multiple encryption methods to protect user data. One method involves encrypting numbers using a scheme called "Pascal Triangle".
Encryption Process:
- Given an array of digits, sum each pair of adjacent digits.
- Take the rightmost digit (least significant digit) of each addition for the next step.
- Repeat the process, reducing the number of digits by 1 in each step, until only 2 digits remain.
- The resulting sequence of 2 digits forms the encrypted number.
Task:
Given the initial sequence of digits, find the encrypted number. You should return a string representing the encrypted number.
Example:
Input: numbers = [4, 5, 6, 7]
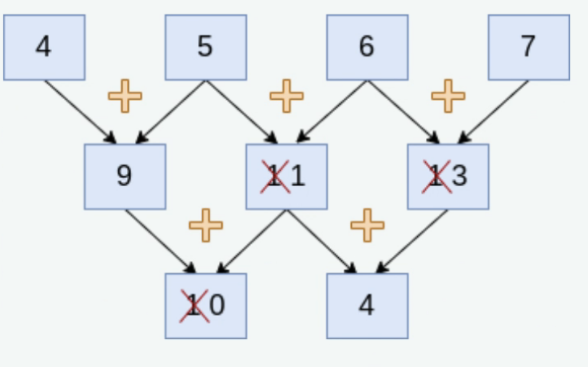
Encryption steps:
- Step 1:
[4+5=9, 5+6=1, 6+7=3]
→[9, 1, 3]
- Step 2:
[9+1=0, 1+3=4]
→[0, 4]
Output: "04"
Function Description:
Complete the function findNumber
in the editor below.
Parameters:
int numbers[n]
: An array of digits.
Returns:
string
: The encrypted number represented as 2 characters.
Constraints:
2 <= len(numbers) <= 5000
0 <= numbers[i] <= 9
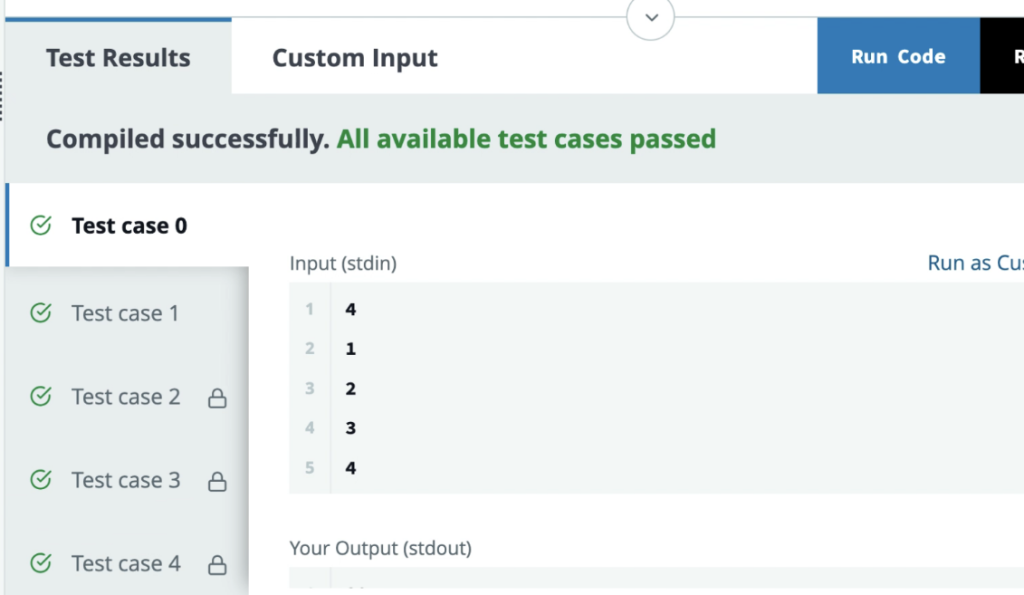
Question 2: Transforming Arrays
Amazon CodeCraft presents a challenge involving two integer lists, initialList
and finalList
:
finalList
contains unique integers.initialList
may have duplicates.
Task:
Determine the minimum number of insertions required to transform the initialList
into a subsequence of the finalList
.
Rules:
- You can insert any positive integer at any position in the
initialList
(including at the start or end). - A subsequence is defined as a sequence derived by deleting zero or more elements without changing the order of the remaining elements.
Example:
Input:
n = 3, finalList = [5, 1, 3]
m = 5, initialList = [9, 4, 2, 3, 4]
Steps:
- Insert
5
intoinitialList
:[5, 9, 4, 2, 3, 4]
- Insert
1
intoinitialList
:[5, 9, 4, 1, 2, 3, 4]
Resulting subsequence: [5, 1, 3]
Output: 2
(minimum insertions required)
Function Description:
Complete the function minimumInsertions
in the editor below.
Parameters:
int finalList[n]
: The target array.int initialList[m]
: The input array.
Returns:
int
: The minimum number of insertions required.
Constraints:
1 <= n, m <= 10^4
1 <= finalList[i], initialList[i] <= 10^5
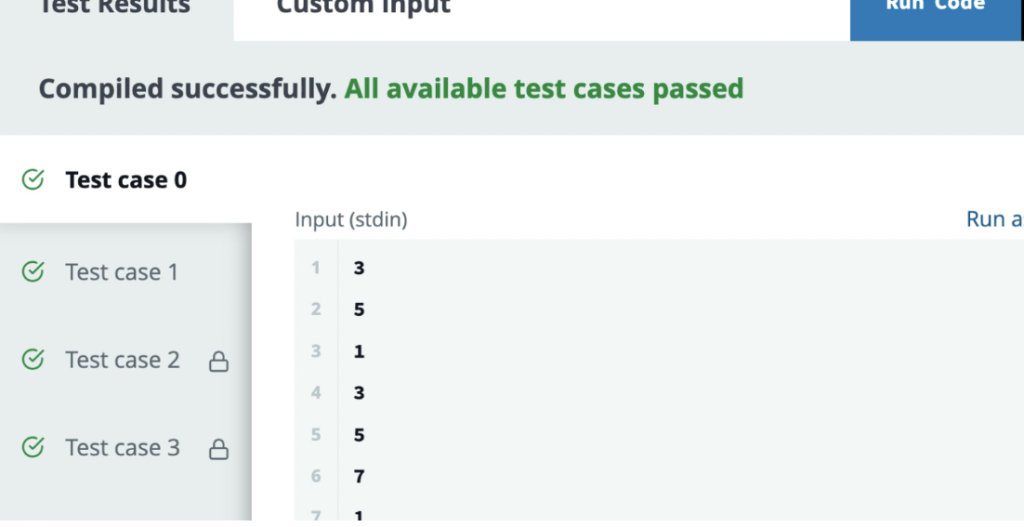
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
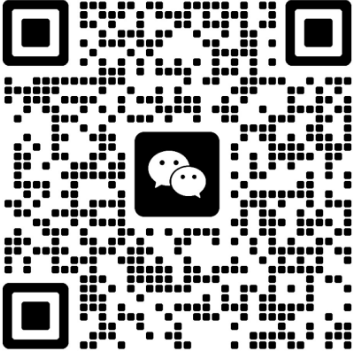