Background
Google is renowned for its challenging yet innovative interview process. This time, I faced an intriguing problem about building a word predictor using a Bigram model. The task focused on testing skills in natural language processing, algorithm optimization, and API design. Here, I’ll walk you through my experience, from clarifying the requirements to discussing solutions and tackling follow-up questions.
You will design and build a word predictor. This word predictor will take some text as training data. You need to provide an API which accepts a word as input and then outputs the most likely next word based on the training data.
The prediction model can be constructed with various different heuristics, but initially, it should be built based on bigram frequency and should optimize for the fastest prediction time.
Examples
Training Data:
[ ["I", "am", "Sam"],
["Sam", "I", "am"],
["I", "like", "green", "eggs", "and", "ham"],
["My", "friends", "like", "green", "shirts"],
]
Predictions:
predict("Sam") => "I"
predict("I") => "am"
predict("like") => "green"
Interview Process
1. Clarifying the Problem
The interviewer began with the following problem statement:
You need to design and implement a word predictor that takes some training text as input. The predictor should accept a single word as input and return the most likely next word based on the training data. The initial implementation should use a Bigram model and prioritize fast prediction time.
I clarified several details to ensure I understood the scope correctly:
- Format of training data: Is the input always a 2D list of text?
- Interviewer: Yes, the input will be a list of sentences, where each sentence is represented as an array of words.
- Prediction logic priority: Should it always be based on word frequency, or can custom weights be applied?
- Interviewer: For now, it should strictly rely on word frequency.
- Handling unmatched words: What if the input word has no matching next word?
- Interviewer: In that case, return an empty string
""
.
- Interviewer: In that case, return an empty string
This step was essential to prevent scope creep and keep my solution aligned with the problem requirements.
2. Discussing the Solution
I outlined my approach step-by-step, and the interviewer probed deeper into each aspect:
- Building the Bigram Frequency Dictionary
- I proposed iterating through the training data to count the occurrences of all consecutive word pairs. The results would be stored in a nested dictionary like:python复制代码
{ "I": {"am": 2, "like": 1}, "am": {"Sam": 1}, }
- Interviewer: How would you handle large datasets efficiently?
- I mentioned using
collections.Counter
to simplify frequency counting and leveraging generators to minimize memory usage.
- I mentioned using
- I proposed iterating through the training data to count the occurrences of all consecutive word pairs. The results would be stored in a nested dictionary like:python复制代码
- Designing the Prediction Function
- Given an input word, I would query the nested dictionary to retrieve the list of potential next words and their frequencies. The function would return the word with the highest frequency.
- Interviewer: How can you ensure the query is fast?
- I explained that dictionary lookups in Python have an average time complexity of O(1)O(1)O(1), making this approach efficient.
- Handling Edge Cases
- If the input word doesn’t exist in the dictionary, return
""
. - If there’s a tie in word frequencies, any one of the highest-frequency words can be returned.
- If the input word doesn’t exist in the dictionary, return
3. Implementation Description
Though the interview didn’t require writing code, I described my implementation steps clearly:
- Data Preprocessing
- Iterate through the training data to count the frequency of every adjacent word pair (Bigram).
- Use a
defaultdict
to handle nested dictionary structures seamlessly.
- Prediction Logic
- Input a single word, retrieve its next-word frequency map, and return the word with the highest frequency.
- If no match is found, return
""
.
- Complexity Analysis
- Preprocessing: O(N)O(N)O(N), where NNN is the total number of words in the training data.
- Prediction: O(1)O(1)O(1) for dictionary lookups.
4. Follow-Up Questions
After I described the basic implementation, the interviewer asked some additional questions to test my understanding and explore potential improvements:
- Extending to Trigrams or Higher N-grams
- How would you modify the solution to predict based on a sequence of words (e.g., Trigrams)?
- I explained that we could extend the dictionary structure to include sequences, e.g.,:python复制代码
{ "I am": {"Sam": 1}, "am Sam": {"I": 1}, }
- Interviewer: What challenges might arise?
- Storage requirements would grow exponentially with longer contexts, and the data sparsity issue might degrade the prediction quality.
- I explained that we could extend the dictionary structure to include sequences, e.g.,:python复制代码
- How would you modify the solution to predict based on a sequence of words (e.g., Trigrams)?
- Supporting Multithreading or Distributed Computing
- How would you scale this solution for massive datasets?
- I suggested splitting the training data into chunks and processing each chunk in parallel. For distributed systems, tools like MapReduce could be employed to handle the frequency aggregation.
- How would you scale this solution for massive datasets?
- Optimizing for Real-Time Use
- The interviewer asked about techniques to optimize the model for real-time predictions.
- I proposed precomputing and caching frequently queried results and using a Trie data structure instead of a dictionary to reduce memory overhead.
- The interviewer asked about techniques to optimize the model for real-time predictions.
5. Final Summary
I concluded the interview by summarizing the solution’s strengths and limitations:
- Strengths: Simple implementation, fast prediction due to dictionary lookups, easily extensible for small datasets.
- Limitations: Limited to Bigram contexts, performance degradation with sparse or large datasets.
- Future Optimizations:
- Adding smoothing techniques (e.g., Laplace smoothing) to handle unseen word pairs better.
- Incorporating more sophisticated language models for improved accuracy.
- Leveraging Trie structures for reduced memory usage.
Interview Takeaways
This interview was a great blend of algorithm design and practical implementation. While the problem itself was relatively straightforward, the follow-up questions added depth and revealed the importance of scalability and optimization in real-world scenarios.
If you’re preparing for Google interviews, here are some tips:
- Brush up on algorithms and data structures: Focus on hashmaps, trees, and their real-world applications.
- Understand time complexity: Be ready to analyze and optimize your solution at every step.
- Practice communication: Explaining your thought process clearly is just as important as solving the problem itself.
This experience reinforced the importance of balancing clarity, efficiency, and scalability in technical problem-solving. I hope my insights help you on your journey to acing the Google interview! Good luck!
Thanks to the rigorous preparation provided by CSOAHelp's Interview Coaching and VO Support, the candidate excelled in this challenging interview. They confidently tackled each question, earning the interviewer’s praise and securing a solid opportunity for their future career. For aspiring candidates, this demonstrates the value of structured preparation and expert guidance.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
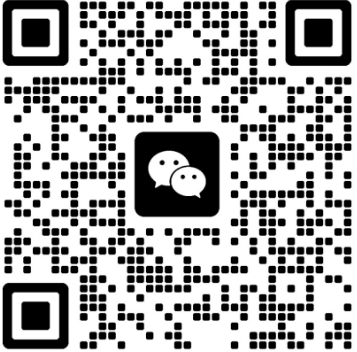