Find Maximum Calories
A user is using the Amazon fitness tracker, and they are engaged in a jumping exercise routine. The user is positioned on the ground, and there are n
stones, each placed at different heights. The height of the i
th stone is represented by height[i]
meters.
The goal is to maximize the calorie burn during this exercise, and the calories burned when jumping from the i
th stone to the j
th stone is calculated as (height[i] - height[j])^2
.
The user intends to practice jumping on each stone exactly once but can choose the order in which they jump. Since the user is looking to optimize their calorie burn for this single session, the task is to determine the maximum amount of calories that can be burned during the exercise.
Formally, given an array height
of size n
, representing the height of each stone, find the maximum amount of calories the user can burn.
Note that the user can jump from any stone to any stone, and the ground's height is 0 and once user jumps to stone from ground, he/she can never go back to ground.
Function Description
Complete the function findMaximumCalories
in the editor below.
findMaximumCalories
has the following parameters:
int height[n]
: the height of each stone
Returns
long
: the maximum amount of calories the user can burn.
Example 1:
Input: height = [5, 2, 5]
Output: 43
Explanation: The user will jump in the following way: Ground → 3rd stone → 2nd stone → 1st stone The calories she will burn can be calculated as:= (0-height[2])^2 + (height[2]-height[1])^2 + (height[1]-height[0])^2
= 25 + 9 + 9
= 43
It can be shown it is not possible to burn more than 43 units of calories, hence, the answer is 43.
Constraints:
1 ≤ n ≤ 10^5
1 ≤ height[i] ≤ 46340
Calculate Warehouse Efficiency (Probably for Supply Chain related NG positions 🙂
The supply chain manager at one of Amazon's warehouses wants to measure the efficiency of the way parcels are shipped. The volume of each parcel is represented in the array parcelWeights
. Each day, the first and last parcels in the array parcelWeights
are shipped until all of them are dispatched.
The manager comes up with metrics to calculate warehouse efficiency. Each day before shipping, any parcel in the warehouse is chosen and its volume is added to the sum of total efficiency. A parcel can only be chosen once.
Given the array parcelWeights
, find the maximum possible efficiency of the warehouse.
Example 1:
Input: parcelWeights = [4, 4, 8, 5, 3, 2]
Output: 17
Explanation:
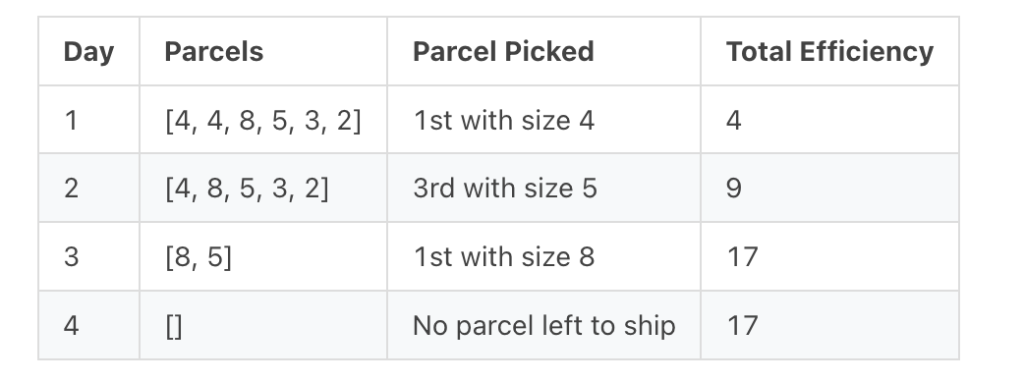
Example 2:
Input: parcelWeights = [2,1,8,5,6,2,4] Output: 23 Explanation: In case 1, the order of picked parcels would produce an answer of 4 + 2 + 8 + 5 = 19, which is incorrect. The correct selection of parcels would be in scenario 2, where the answer will be 4 + 6 + 8 + 5
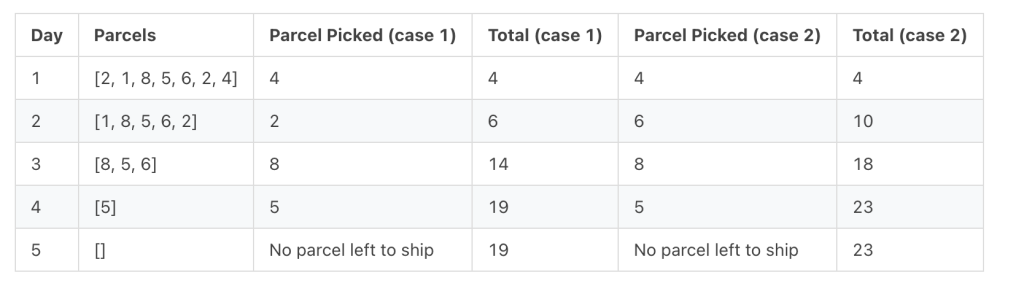
Constraints:
1<=n<=2x10^5 0<=parcle_weights[i]<=10^9
Sorting Strings Based on Custom Character Order
AWS provides several utilities for language processing. Develop a helper service to sort a list of strings based on a custom order of characters.
Detailed Description: You are given a custom alphabet system that consists of exactly kkk characters. The alphabetical order is provided as a string of distinct characters, order
. An array arr
contains nnn strings, and you must sort these strings according to the given special ordering. Each string in arr
contains only characters from order
.
Note: A string x=x1x2...xnx = x_1x_2...x_nx=x1x2...xn is lexicographically smaller than string y=y1y2...ymy = y_1y_2...y_my=y1y2...ym, if:
- n<mn < mn<m, and x1=y1,x2=y2,...,xm=ymx_1 = y_1, x_2 = y_2, ..., x_m = y_mx1=y1,x2=y2,...,xm=ym, or
- There exists a number rrr (r<n,r<mr < n, r < mr<n,r<m) such that x1=y1,x2=y2,...,xr=yrx_1 = y_1, x_2 = y_2, ..., x_r = y_rx1=y1,x2=y2,...,xr=yr, and xr+1<yr+1x_{r+1} < y_{r+1}xr+1<yr+1.
Example:
Given k=5k = 5k=5, order = "7BbAz"
, n=4n = 4n=4, arr = ["Abb", "A7z", "Z7AAAA", "BbbABB"]
, the comparisons are as follows:
String1 | String2 | Larger String | Explanation |
---|---|---|---|
Abb | A7z | Abb | The first characters are the same, but 7 comes before b in order . |
Abb | Z7AAAA | Z7AAAA | Z comes after A. |
Abb | BbbABB | Abb | A comes after B. |
A7z | Z7AAAA | Z7AAAA | Z comes after A. |
A7z | BbbABB | A7z | A comes after B. |
Z7AAAA | BbbABB | Z7AAAA | Z comes after B. |
Sorted Result: ["BbbABB", "A7z", "Abb", "Z7AAAA"]
Function Description:
Complete the function customSortString
with the following parameters:
- string
order
: The custom order alphabet of kkk characters. - string[]
arr
: The strings to sort.
Returns:
- string[]: The sorted array of strings.
Constraints:
- The number of characters in
order
(kkk) ranges from 1 to 62. - The number of strings in
arr
(nnn) ranges from 1 to 100,000. - The total length of all strings in
arr
does not exceed 1,000,000. - All characters in
arr[i]
are present inorder
. - All characters in
order
are distinct and consist of alphanumeric characters (a-z
,A-Z
,0-9
).
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
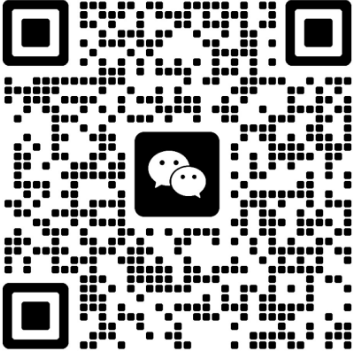