Meta 面试:算法挑战详解
场景: 在一次Meta的技术面试中,我遇到了一系列算法挑战。整个面试过程中,面试官通过交互式的问题和澄清,测试了我在压力下解决问题的能力。以下是这次面试中的三道题目及其详细讨论和解答。
Scenario: During a technical interview with Meta, I was presented with a series of algorithmic challenges. Throughout the interview, the interviewer tested my ability to solve problems under pressure through interactive questions and clarifications. Below are three problems from the interview, along with detailed discussions and solutions.
问题1:局部最小值的查找
问题描述: 给定一个整数数组,找到数组中的任意一个局部最小值。局部最小值被定义为数组中比邻居元素小于或等于的整数。
示例:
输入: [5, 9, 11, 14, 7, 8]
输出: 5 或 7
面试对话:
面试官: “在开始之前,我想了解一下,你对于局部最小值的理解是什么?”
我: “局部最小值是在数组中比相邻元素小或等于的元素。比如在数组 [5, 9, 11, 14, 7, 8] 中,5 和 7 都是局部最小值。”
面试官: “很好。你会考虑用什么方法来解决这个问题?”
我: “一种简单的方法是遍历数组并检查每个元素是否比它的邻居小。这种方法的时间复杂度是 O(n)。不过,我在想是否可以通过二分法来优化这个过程,减少搜索空间。”
面试官: “有趣,能详细解释一下你的二分法思路吗?”
我: “当然。基本上,我们可以在数组中间选择一个元素,如果它不是局部最小值,那么至少有一侧(左侧或右侧)包含局部最小值。我们可以递归地或者迭代地缩小搜索范围,这样时间复杂度可以降到 O(log n)。”
面试官: “很棒,这个思路是非常合理的。”
Problem 1: Finding a Local Minimum
Problem Description: Given an array of integers, find any one local minimum from the array. A local minimum is defined as an integer in the array that is less than or equal to its neighbors.
Example:
Input: [5, 9, 11, 14, 7, 8]
Output: 5 or 7
Interview Conversation:
Interviewer: “Before we start, could you explain your understanding of a local minimum?”
Me: “A local minimum is an element in an array that is smaller than or equal to its neighboring elements. For example, in the array [5, 9, 11, 14, 7, 8], both 5 and 7 are local minima.”
Interviewer: “Great. How would you approach solving this problem?”
Me: “One straightforward approach is to iterate through the array and check if each element is smaller than its neighbors. This approach has a time complexity of O(n). However, I’m considering whether a binary search could optimize this process and reduce the search space.”
Interviewer: “Interesting, can you elaborate on your binary search approach?”
Me: “Sure. Essentially, we can select a middle element in the array. If it’s not a local minimum, then at least one side (left or right) must contain a local minimum. We can recursively or iteratively narrow down the search range, reducing the time complexity to O(log n).”
Interviewer: “Excellent, that’s a very logical approach.”
问题2:连续子数组和目标值
问题描述: 给定一个整数序列和一个目标和,确定是否存在一个连续的子数组,其元素之和等于目标值。
示例:
输入: [1, 3, 1, 4, 23], 目标: 8
输出: True(因为 3 + 1 + 4 = 8)
面试对话:
面试官: “你认为如何有效地查找一个连续子数组,使得其和等于给定的目标值?”
我: “我首先会想到用滑动窗口的技术,这样我们可以在 O(n) 的时间内解决这个问题。”
面试官: “滑动窗口技术?你能详细说明一下吗?”
我: “当然。我们可以使用两个指针,一个指向子数组的开始位置,另一个指向结束位置。我们从头开始扫描数组,逐渐扩大窗口。如果窗口内的和大于目标值,我们就缩小窗口;如果小于目标值,我们就扩大窗口。当窗口内的和等于目标值时,我们就找到了一个解。”
面试官: “这样做的时间和空间复杂度如何?”
我: “时间复杂度是 O(n),因为每个元素最多被处理两次。空间复杂度是 O(1),因为我们只用了常量级的额外空间。”
面试官: “听起来是个不错的方案。”
Problem 2: Continuous Subarray Sum to Target
Problem Description: Given a sequence of integers and a target sum, determine whether there exists a continuous subarray that sums up to the target.
Example:
Input: [1, 3, 1, 4, 23], Target: 8
Output: True (because 3 + 1 + 4 = 8)
Interview Conversation:
Interviewer: “How do you think you could efficiently find a continuous subarray that sums up to the target value?”
Me: “I would consider using a sliding window technique, which would allow us to solve the problem in O(n) time.”
Interviewer: “Sliding window technique? Can you explain that in more detail?”
Me: “Sure. We can use two pointers, one indicating the start of the subarray and the other indicating the end. We begin scanning the array, expanding the window. If the sum of the window exceeds the target, we shrink it by moving the start pointer. If the sum equals the target, we’ve found our solution.”
Interviewer: “What about the time and space complexity of this approach?”
Me: “The time complexity is O(n) because each element is processed at most twice, and the space complexity is O(1) since only a few extra variables are used.”
Interviewer: “Sounds like a solid plan.”
问题3:二维迷宫中的最短路径
问题描述: 你被给定了一个由0和1组成的二维游戏板,0表示可通过的位置,1表示不可通过的位置。设计一个算法,找到从左上角到右下角的最短路径。
示例:
输入:
0 0 0 0 0 0 0
0 1 0 1 0 1 0
0 1 0 1 1 0 1
1 1 1 0 0 0 0
输出: [(0, 0), (0, 1), (0, 2), (1, 2), (2, 2), (3, 2), (4, 2), (4, 3), (4, 4), (4, 5), (4, 6)]
面试对话:
面试官: “对于这个问题,你会考虑使用什么样的方法?”
我: “这是一个典型的图搜索问题,最直接的方法是使用广度优先搜索(BFS),因为它能够保证找到最短路径。”
面试官: “为什么你认为BFS更适合这个问题?”
我: “因为BFS在遍历时会逐层扩展,第一次到达目标节点时的路径长度就是最短的。相比之下,深度优先搜索(DFS)可能会先探索到一条较长的路径。”
面试官: “确实如此。那么你会如何实现BFS呢?”
我: “我们可以使用一个队列来存储当前的路径节点,然后逐步扩展到邻居节点,直到找到出口。每次扩展时,我们记录路径长度。”
面试官: “很好,这听起来是一个有效的解决方案。”
Problem 3: Shortest Path in a 2D Maze
Problem Description: You are given a game board represented as a 2D array of zeroes and ones. Zero stands for passable positions and one stands for impassable positions. Design an algorithm to find the shortest path from the top-left corner to the bottom-right corner.
Example:
Input:
0 0 0 0 0 0 0
0 1 0 1 0 1 0
0 1 0 1 1 0 1
1 1 1 0 0 0 0
Output: [(0, 0), (0, 1), (0, 2), (1, 2), (2, 2), (3, 2), (4, 2), (4, 3), (4, 4), (4, 5), (4, 6)]
Interview Conversation:
Interviewer: “What approach would you take to solve this problem?”
Me: “This is a classic graph traversal problem. The most straightforward approach would be to use Breadth-First Search (BFS) because it guarantees the shortest path.”
Interviewer: “Why do you believe BFS is the best fit for this problem?”
Me: “BFS expands level by level, ensuring that the first time it reaches the target, the path is the shortest. In contrast, Depth-First Search (DFS) might explore longer paths first.”
Interviewer: “Indeed. How would you implement BFS?”
Me: “We can use a queue to store the current path nodes, and progressively extend the path to neighboring nodes until we find the exit. Each extension records the path length.”
Interviewer: “Great, this sounds like an effective solution.”
在这次Meta的面试中,通过这三道题目,我展示了对常见算法问题的理解和解决能力。每道题目都有不同的挑战,但都可以通过合理的算法策略来解决。
如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
In this Meta interview, I demonstrated my understanding of common algorithmic problems and my problem-solving abilities. Each problem presented different challenges, but all could be solved through logical algorithm strategies.
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
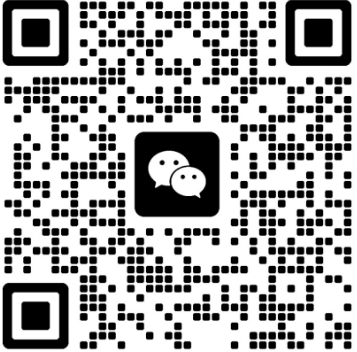