问题描述
在这道Uber的面试题中,要求给定一个二叉搜索树(BST)和一个正整数k,找到二叉搜索树中的第k大元素。候选人被要求理解和实现这一算法。例如,在下图所示的二叉搜索树中,如果k=3,输出应为15;如果k=5,输出应为4。
10
/ \
4 20
/ \ \
2 5 40
Given a Binary Search Tree (BST) and a positive integer k, find the k-th largest element in the Binary Search Tree.
For example, in the following BST, if k = 3, then output should be 15, and if k = 5, then output should be 4.
10
/ \
4 20
/ \ \
2 5 40
面试中的澄清
在面试过程中,候选人与面试官进行了详细的讨论,以澄清一些关键问题:
- 是否可以假设二叉树至少包含k个元素?
- 面试官确认可以假设二叉树至少包含k个元素。
- 如果树的大小小于k,应该返回什么?
- 返回-1可能会引起混淆,因为-1可能是树中的有效值。候选人建议在这种情况下抛出一个错误,面试官同意了这一建议。
- 是否需要定义树节点类?
- 面试官确认需要定义树节点类,以确保候选人能够构建和操作二叉搜索树。
Clarifications During the Interview
During the interview, the candidate and the interviewer discussed several key points to clarify the problem:
- Can we assume the binary tree contains at least k elements?
- The interviewer confirmed that this assumption is valid.
- What should be returned if the size of the tree is less than k?
- Returning -1 could be confusing since -1 might be a valid value in the tree. The candidate suggested throwing an error in such cases, which the interviewer agreed with.
- Do we need to define the TreeNode class?
- The interviewer confirmed that defining the TreeNode class is necessary to construct and manipulate the BST.
算法设计
候选人与面试官讨论了几种可能的解决方案,最终决定使用反向中序遍历的方法。该方法利用二叉搜索树的性质,从树的右子树开始遍历,这样可以按降序访问节点。
具体步骤如下:
- 从根节点(10)开始,向右子树移动到节点(20)。
- 继续向右移动到节点(40)。
- 节点(40)没有子节点,将计数器加1,此时计数器为1。
- 回到节点(20),将计数器加1,此时计数器为2。
- 移动到节点(20)的左子节点(15),将计数器加1,此时计数器为3,找到了第3大元素(15)。
Algorithm Design
The candidate and the interviewer discussed various approaches and ultimately decided on using the reverse in-order traversal method. This method leverages the properties of the BST by traversing the tree from the rightmost node to the leftmost node, effectively visiting nodes in descending order.
The detailed steps are as follows:
- Start at the root node (10) and move to the right child node (20).
- Continue moving to the right to node (40).
- Since node (40) has no children, increment the counter to 1.
- Move back to node (20) and increment the counter to 2.
- Move to the left child of node (20), which is node (15), and increment the counter to 3, finding the 3rd largest element (15).
算法复杂度分析
- 时间复杂度:最坏情况下为O(n),因为可能需要遍历所有节点。
- 空间复杂度:为O(h),其中h是树的高度。递归调用的栈深度与树的高度成正比。
Complexity Analysis
- Time Complexity: In the worst case, the time complexity is O(n) because we might need to traverse all nodes.
- Space Complexity: The space complexity is O(h), where h is the height of the tree. This is because the depth of the recursive call stack is proportional to the height of the tree.
延伸问题与优化
面试的后半段,面试官询问如何处理多个k值的问题。候选人提出,可以通过一次遍历找到多个k值对应的元素,从而避免多次遍历。具体思路如下:
- 将k值存储在一个数组中,并找出最大值max_k。
- 在进行反向中序遍历时,用一个计数器记录已访问的节点数。
- 当计数器值在k值数组中时,将对应节点值存储在结果字典中。
- 最终返回结果字典,其中包含所有k值对应的节点值。
Extensions and Optimizations
In the latter part of the interview, the interviewer asked how to handle multiple k values. The candidate proposed an extension to the current solution, allowing for the retrieval of multiple k-th largest elements with a single traversal, thus avoiding multiple traversals.
The key ideas are:
- Store the k values in an array and identify the maximum k value (max_k).
- Perform a reverse in-order traversal, maintaining a counter for the number of nodes visited.
- When the counter matches any value in the k array, store the corresponding node value in a results dictionary.
- Return the results dictionary containing all k-th largest elements.
面试结束时的提问
面试结束时,候选人可以向面试官提问,以更好地了解公司文化和职位期望。以下是一些具体的问题:
- 日常工作安排:候选人可以询问“能否分享一下这个职位的日常工作安排?团队的日常工作流程是怎样的?”
- 项目范围:候选人可以问“在这个岗位上,我会参与到哪些项目中?这些项目的主要目标和挑战是什么?”
- 职业发展机会:候选人可以问“公司如何支持员工的职业发展?是否有培训和发展计划?”
Questions to Ask the Interviewer
At the end of the interview, the candidate can ask the interviewer specific questions to gain better insight into the company culture and job expectations. Here are some examples:
- Daily Work Schedule: "Can you share what a typical day looks like for someone in this position? What is the team's daily workflow like?"
- Project Scope: "What kind of projects would I be working on in this role? What are the main goals and challenges of these projects?"
- Career Development Opportunities: "How does the company support employee career development? Are there training and development programs available?"
总结
本文详细讨论了Uber面试题中如何在二叉搜索树中找到第k大元素的算法设计与实现,并通过描述候选人与面试官的讨论过程,展示了思考问题和澄清问题的关键步骤。希望这篇博客能帮助更多的求职者理解这一经典问题的解决方案,并在面试中取得成功。
Conclusion
This article detailed the algorithm design and implementation for finding the k-th largest element in a BST, based on an Uber interview question. By recounting the candidate’s discussion with the interviewer, the article illustrates the importance of clarifying problem details and considering various solution approaches. Hopefully, this blog will help other job seekers understand and solve this classic problem, enhancing their preparation for technical interviews.
With our powerful interview assistance, candidates can effectively analyze and communicate their solutions to these interview questions. This not only demonstrates their programming abilities to the interviewer but also showcases their clear problem-solving approach and effective communication skills. These abilities are valuable not only for Uber interviews but also for solving real-world programming problems. We wish you all the best in your interviews!
If you need our interview assistance services, please contact us immediately.
面试辅导,面试代理,请联系我们。
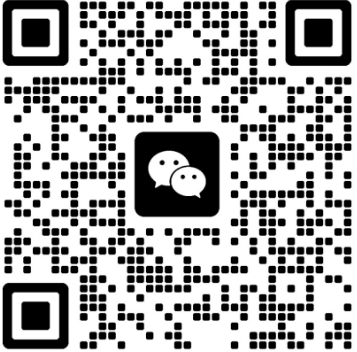