题目介绍
面试官展示了一道题目,并解释道:“这道题目要求你处理一个仅包含小写英文字母的字符串。你可以在一次操作中将字符串中的任意一个字符更改为另一个字符。我们要判断在执行一或两次操作后,是否能使这个字符串变成回文。”
应聘者:“明白了。我可以先问一个问题吗?输入的字符串只包含小写字母吗?”
面试官:“是的,字符串只包含小写字母。”
The interviewer presented a problem and explained: "This problem requires you to handle a string consisting of only lowercase English letters. You can change any character in the string to any other character in one operation. We need to determine if the string can be turned into a palindrome by performing exactly one or two operations."
Candidate: "Got it. Can I ask a question first? Does the input string contain only lowercase letters?"
Interviewer: "Yes, the string contains only lowercase letters."
解题思路
候选人:“我认为可以使用双指针技术来解决这个问题。我们可以用两个指针,一个从字符串的左端开始,另一个从右端开始,逐步向中间移动,检查每一对字符是否匹配。”
面试官:“能详细说明一下具体步骤吗?”
候选人:“好的。我们从左边和右边同时扫描字符串,每次检查左右指针指向的字符是否相同。如果不同,我们将计数器加1。当计数器大于2时,我们就知道需要超过两次操作,因此返回false。如果扫描完整个字符串后,计数器不超过2,则说明最多需要两次操作即可变成回文,返回true。”
面试官:“嗯,这样的算法时间复杂度是多少?”
候选人:“时间复杂度是O(n),因为我们需要扫描整个字符串一遍。空间复杂度是O(1),因为我们只需要常量级的额外空间来存储计数器和指针。”
Candidate: "I think we can solve this problem using a two-pointer technique. We can use two pointers, one starting from the left end of the string and the other from the right end, moving towards the center to check each pair of characters."
Interviewer: "Can you explain the specific steps in detail?"
Candidate: "Sure. We start by initializing a counter to record the number of mismatched character pairs. Then, we use two pointers, one pointing to the beginning and the other to the end of the string. If the characters pointed to by the two pointers do not match, we increment the mismatch counter. If the mismatch counter exceeds 2, we return false because it's impossible to turn the string into a palindrome with just two operations. If the mismatch counter is 2 or less after scanning the entire string, we return true."
Interviewer: "That sounds clear. What's the time complexity of this algorithm?"
Candidate: "The time complexity is O(n) because we need to scan the entire string once. The space complexity is O(1) because we only need a constant amount of extra space to store the counter and the pointers."
链表变种
面试官:“很好。如果现在字符串变成了链表,你会如何修改你的算法?”
应聘者:“当然。首先,我们使用快慢指针找到链表的中点。然后,反转链表的后半部分。接着,我们用一个指针从链表头部开始,另一个指针从反转后的链表头部开始,比较两个指针指向的节点值。如果不匹配,计数器加1。当计数器超过2时,返回false。否则,遍历完整个链表后,返回true。”
面试官:“你能再具体一些吗?比如说,如果我们不想额外使用O(n)的空间,还有其他方法吗?”
应聘者:“当然可以。我们可以在不使用额外空间的情况下反转链表的后半部分。具体来说,我们可以先找到链表的中点,然后从中点开始反转链表的后半部分。在反转过程中,我们保持两个指针,一个指向链表头部,另一个指向反转后的链表头部,逐一比较节点值。如果节点值不匹配,我们增加计数器。如果计数器超过2,返回false。否则,返回true。”
面试官:“那如果我们使用栈呢?会不会更简单?”
应聘者:“是的,使用栈也可以实现。我们可以将链表的前半部分节点值依次压入栈中,然后从中点开始逐一弹出栈顶元素,与链表后半部分的节点值进行比较。如果节点值不匹配,我们增加计数器。如果计数器超过2,返回false。否则,返回true。不过这种方法的空间复杂度是O(n),因为需要额外的栈来存储节点值。”
面试官:“对,使用栈的空间复杂度是O(n)。你提到反转链表的方法更高效,那么这个方法的空间复杂度是多少?”
应聘者:“如果只反转链表的后半部分,空间复杂度是O(1),因为我们只需要常量级的额外空间来存储几个指针。”
面试官:“非常好,你对链表的处理也很到位。”
nterviewer: "Good. Now, if the string were a linked list, how would you modify your algorithm?"
Candidate: "Of course. First, we can use a fast and slow pointer to find the midpoint of the linked list. Then, we reverse the second half of the linked list. Next, we use one pointer starting from the head of the list and another starting from the head of the reversed second half to compare the nodes' values. If they don't match, we increment the mismatch counter. If the counter exceeds 2, we return false. Otherwise, we return true after traversing the entire list."
Interviewer: "Can you be more specific? For example, if we want to avoid using O(n) additional space, is there another method?"
Candidate: "Absolutely. We can reverse the second half of the linked list in place without using extra space. Specifically, after finding the midpoint of the list, we reverse the second half starting from the midpoint. During the reversal, we maintain two pointers, one pointing to the head of the list and the other pointing to the head of the reversed second half, to compare the nodes' values one by one. If the values don't match, we increment the counter. If the counter exceeds 2, we return false. Otherwise, we return true."
Interviewer: "What if we use a stack? Wouldn't that be simpler?"
Candidate: "Yes, using a stack is also a viable solution. We can push the node values of the first half of the linked list onto a stack, then starting from the midpoint, we pop the stack and compare the popped values with the node values of the second half of the list. If the values don't match, we increment the counter. If the counter exceeds 2, we return false. Otherwise, we return true. However, this method's space complexity is O(n) because we need extra space to store the stack."
Interviewer: "Right, the space complexity of using a stack is O(n). You mentioned that reversing the linked list in place is more efficient. What's the space complexity of that method?"
Candidate: "If we only reverse the second half of the linked list in place, the space complexity is O(1) because we only need a constant amount of extra space to store a few pointers."
Interviewer: "Very good. You handled the linked list scenario well."
经过我们的强力面试辅助,候选人通过这些面试题的解析和沟通,面试官不仅了解了候选人的编程能力,也看到了我在解决问题过程中清晰的思路和有效的沟通技巧。这些不仅有助于应对 微软的面试,同时也能提升我们解决实际编程问题的能力。祝大家面试顺利!
如果你也需要我们的面试辅助服务,请立即联系我们。
With our powerful interview assistance, candidates can effectively analyze and communicate their solutions to these interview questions. This not only demonstrates their programming abilities to the interviewer but also showcases their clear problem-solving approach and effective communication skills. These abilities are valuable not only for Microsoft interviews but also for solving real-world programming problems. We wish you all the best in your interviews!
If you need our interview assistance services, please contact us immediately.
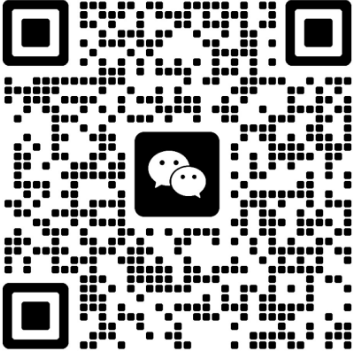