Question
The current selected programming language is Python3. We emphasize the submission of a fully working code over partially correct but efficient code. Use of certain header files are restricted. Once submitted, you cannot review this problem again. You can use print
to debug your code. The print
may not work in case of syntax/runtime error. The version of Python being used is 3.4.3
Emma wishes to give her father a bouquet for his birthday. She asks for help from her mother Rosy. Rosy gives N flower sticks numbered 1 to N to Emma and tells her to arrange them in the bouquet in a particular order. She asks Emma to arrange the first K flower sticks in the order of increasing length and the remaining sticks in the order of decreasing length.
Write an algorithm to find the final arrangement of the flower sticks in the bouquet.
Input
- The first line of the input consists of an integer –
flowerStick_size
, representing the number of flower sticks (N) - The second line consists of N space-separated integers –
flowerStick[1]
,flowerStick[2]
...flowerStick[N]
, representing the length of the flower sticks - The last line consists of an integer –
random
, representing the number K given by Rosy to Emma
Output
Print N space-separated integers representing the final arrangement of the flower sticks in the bouquet.
Constraints
0 ≤ random ≤ flowerStick_size
0 < flowerStick_size < 10⁶
Example
Input
8
1 7 5 11 46 23 16 8
3
Output
5 7 11 46 23 16 10 8
Explanation
Emma has to arrange the first three flower sticks in an increasing order of the length and remaining sticks in the decreasing order of the length.
The final order of flower sticks in the bouquet is [5, 7, 11, 46, 23, 16, 10, 8]
.
Question
The current selected programming language is Python3. We emphasize the submission of a fully working code over partially correct but efficient code. Use of certain header files are restricted. Once submitted, you cannot review this problem again. You can use print
to debug your code. The print
may not work in case of syntax/runtime error. The version of Python being used is 3.4.3
The city authorities conduct a study of the houses in a residential area for a city planning scheme. The area is depicted in an aerial view and divided into an N x M grid. If a grid cell contains some part of a house roof, then it is assigned the value 1; otherwise, the cell represents a vacant plot and is assigned the value 0. Clusters of adjacent grid cells with value 1 represent a single house. Diagonally placed grids with value 1 do not represent a single house. The area of a house is the number of 1s that it spans.
Write an algorithm to find the area of the largest house.
Input
- The first line of the input consists of two space-separated integers –
rows
andcols
representing the number of rows (N) and the number of columns in the grid (M), respectively - The next N lines consist of M space-separated integers representing the grid
Output
Print an integer representing the area of the largest house.
Constraints
The elements of the grid consist of 0s and 1s only.
Example
Input
5 5
0 0 0 0 0
0 1 1 0 0
0 0 0 0 0
0 0 1 1 0
0 1 0 0 0
Output
3
Explanation
The area of the biggest house is 3.
So, the output is 3.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
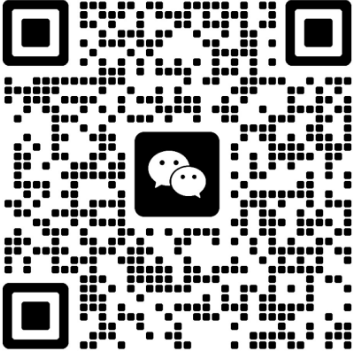