Implement a postfix calculator in C++
Question Description
The postfix calculator application:
- Write a postfix calculator application in C++.
- The only operators you need to implement are '+', '-', '*', and '/'. However, your implementation should make it relatively easy to add more operators, such as 'pow', 'sqrt' or etc. Feel free to implement these additional operators should you feel adventurous.
- The calculator should accept floating point numbers and output floating point numbers (i.e. when the input is
2 3 /
the result is0.66667
). - The calculator will read a postfix expression contained in a single line from stdin and output the result to stdout.
- You can use a different container to implement it instead of using a stack and be able to explain why.
- After each evaluation, the calculator must prompt for another expression, continuing until the user enters the text “exit” (without the quotes).
- The calculator must output an error message if the expression contains an error. For example, characters like
a
or?
or~
will generate error messages. Invalid postfix expressions must also generate error messages. For example, the expression2 + 3
is not a valid postfix expression. There are four types of error messages:
"Invalid operand"
"Invalid operator"
"Too many operands"
"Not enough operands"
Note: Your application will need to return these exact strings to pass some of the test cases.
- A testing methodology should be considered. You do not necessarily need to write tests, but you should be able to talk about how you would test.
- You may consult the web for library reference only, but copying a solution from websites or LLM (e.g. ChatGPT, Claude, etc) will be recognized, especially since none of them are particularly good.
Basic Algorithm:
For each token 'ch'
in the postfix expression, do:
- if
'ch'
is an operatorop
, then
a :=
pop first element from stackb :=
pop second element from the stackres := op(b, a)
- push
res
into the stack
- else if
'ch'
is an operand, then
- add
ch
into the stack
Provided Test Cases:
The test cases given below are not comprehensive, but rather represent a small sample to illustrate the expected functionality.
Input | Expected Output |
---|---|
2 3 / | 0.666667 |
2.0 6 - | -4 |
200.0 -5.0 * | -1000 |
error | Invalid operator |
100 3 4 + 1 | Too many operands |
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
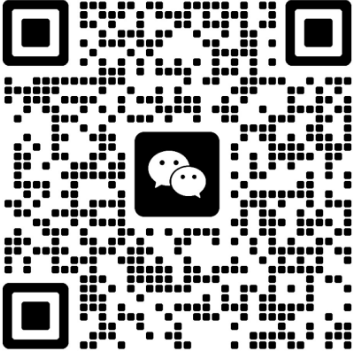