In the world of technical interviews, you might think that companies primarily test algorithms and coding skills. However, the reality is that they assess your ability to handle pressure, articulate your thoughts clearly, and think on your feet. Today, we will revisit a candidate’s real interview experience at Cresta and reveal how CSOAHELP’s real-time interview assistance service provided structured guidance, allowing the candidate to confidently navigate an unfamiliar problem and pass the interview.
L, a software engineer with a few years of Python experience, received an interview invitation from Cresta. He was confident that the questions would focus on data structures and algorithms, so he diligently reviewed common LeetCode problems. However, as soon as the interview started, the first question caught him off guard:
You are tasked with implementing a library for managing file storage in a cloud-based application.
The library should allow users to upload, retrieve, and delete files efficiently.
Additionally, the library should provide a way to list all files with metadata such as file size and upload timestamp.
Requirements:
- upload_file(file_name: str, file_content: bytes) -> str
- get_file(file_id: str) -> bytes
- delete_file(file_id: str) -> bool
- list_files() -> List[Dict[str, Any]]
L saw the question, and his heart skipped a beat. He had never implemented a full-fledged file storage system before, and for a moment, he struggled to organize his response. Worse yet, the interviewer's expectant silence added pressure, making his mind go blank.
At that moment, CSOAHELP’s real-time interview assistance service displayed a structured response framework on his screen. He quickly gathered his thoughts and confidently repeated the structured explanation:
"We need a storage system to manage files, so I would use a dictionary to store file data and another dictionary to store metadata. Each file should have a unique ID, which can be generated using
uuid
. Additionally, we need to track the filename, size, and upload timestamp. This approach allows us to efficiently upload, retrieve, and delete files in O(1) time complexity."
The interviewer nodded in acknowledgment, signaling him to proceed with writing the code. Before L began coding, CSOAHELP provided a complete code implementation, ensuring he could understand and articulate the solution without getting stuck on minor details.
import uuid
import datetime
class FileStorage:
def __init__(self):
self.files = {} # Store file data
self.metadata = {} # Store file metadata
def upload_file(self, file_name, file_content):
file_id = str(uuid.uuid4()) # Generate a unique ID
file_size = len(file_content)
upload_time = datetime.datetime.utcnow().isoformat() # Record upload time
self.files[file_id] = file_content # Store file content
self.metadata[file_id] = {
"file_id": file_id,
"file_name": file_name,
"file_size": file_size,
"upload_time": upload_time
}
return file_id
L smoothly recited the code and added some personal insights, demonstrating confidence in the approach. The interviewer nodded, signaling him to implement the get_file()
method.
L quickly referenced CSOAHELP’s complete code and immediately delivered the solution:
def get_file(self, file_id):
return self.files.get(file_id, None) # O(1) lookup, returns None if the file is not found
The interviewer then challenged him, asking, “If this system stores a million files, how would you optimize the get_file()
method?”
With CSOAHELP’s structured guidance, L confidently responded:
"Since dictionary lookups have O(1) time complexity, querying one million files remains efficient. However, if we consider distributed storage, we could use Redis caching to minimize direct disk lookups and improve performance further."
The interviewer nodded approvingly and proceeded with the next question.
Next, the interviewer asked L to implement the delete_file()
method. L referenced CSOAHELP’s complete code implementation and effortlessly wrote the function:
def delete_file(self, file_id):
if file_id in self.files:
del self.files[file_id] # Delete file content
del self.metadata[file_id] # Remove metadata
return True
return False
The interviewer then raised the difficulty level by asking, “How would you modify delete_file()
to retain metadata after deletion?”
L promptly referenced CSOAHELP’s optimized solution and explained the modification:
def delete_file(self, file_id):
if file_id in self.files:
del self.files[file_id]
self.metadata[file_id]["file_size"] = 0
self.metadata[file_id]["deleted"] = True # Mark as deleted instead of removing metadata
return True
return False
The interviewer seemed pleased with this response and moved on to the final method, list_files()
. L referenced CSOAHELP’s solution and implemented it smoothly:
def list_files(self):
return list(self.metadata.values())
After implementing the function, L further added:
"This function runs in O(N) time complexity since it retrieves metadata for all files. If needed, we could optimize it by using database indexing or caching layers to improve query efficiency."
The interviewer smiled, clearly satisfied, and then posed one last question: “How would you adapt this system for cloud storage, such as AWS S3?”
L referred to CSOAHELP’s expansion-oriented response and answered confidently:
"If we migrate to AWS S3, we could store file content in S3 and update the
metadata
dictionary to include S3 URLs instead of storing files in memory. Additionally, we could use boto3 for file uploads and downloads, while leveraging AWS Lambda triggers to update metadata automatically."
The interviewer’s expression indicated he was impressed. The interview concluded on a positive note, with L leaving a strong final impression.
Throughout this interview, L did not rely on sheer improvisation. Instead, he used CSOAHELP’s real-time assistance to access a structured response framework, pre-written code, and optimized solutions. This enabled him to confidently respond to unfamiliar questions and articulate his answers effectively.
If you’re worried about freezing up during an interview or struggling to articulate your thoughts, CSOAHELP is your silent mentor, helping you stay calm and secure that offer with ease!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
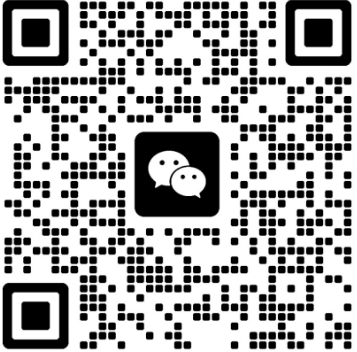