You are given a 0-indexed integer array nums
. You are allowed to permute nums
into a new array perm
of your choosing.
We define the greatness of nums
be the number of indices 0 <= i < nums.length
for which perm[i] > nums[i]
.
Return the maximum possible greatness you can achieve after permuting nums
.
Example 1:
Input: nums = [1,3,5,2,1,3,1] Output: 4 Explanation: One of the optimal rearrangements is perm = [2,5,1,3,3,1,1]. At indices = 0, 1, 3, and 4, perm[i] > nums[i]. Hence, we return 4.
Example 2:
Input: nums = [1,2,3,4] Output: 3 Explanation: We can prove the optimal perm is [2,3,4,1]. At indices = 0, 1, and 2, perm[i] > nums[i]. Hence, we return 3.
Constraints:
1 <= nums.length <= 105
0 <= nums[i] <= 109
Problem 1: Maximum Valid Connections in a Directed Tree
You are given a directed tree with n nodes, where each node is labeled from 0 to n-1. The tree structure is defined by three arrays:
- from_nodes and to_nodes, where from_nodes[i] represents the parent node of to_nodes[i], forming a directed edge (from_nodes[i] → to_nodes[i]).
- cost, where cost[i] represents the cost associated with the edge (from_nodes[i] → to_nodes[i]).
- Additionally, you are given an array value of length n, where value[i] represents the value associated with node i.
Valid Connection Criteria:
A connection (u → v) is valid if and only if:
- u is an ancestor of v in the tree (i.e., there is a directed path from u to v).
- The cost of the connection cost[i] is strictly less than value[v].
Output:
Return the maximum number of valid connections in the tree.
Problem 2: House Coloring with Constraints
There are n houses along a street, and we need to color each house using one of three colors while following two constraints:
- Adjacent houses must have different colors.
- Symmetric houses must have different colors. Specifically:
- The 1st and nth house must have different colors.
- The 2nd and (n-1)th house must have different colors.
- The 3rd and (n-2)th house must have different colors, and so on.
Input:
- N (even integer) representing the number of houses.
Output:
- Return the total number of valid colorings modulo 10⁷.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
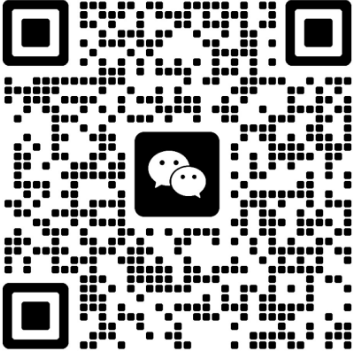