Amazon's technical interviews are known to be challenging. Do you worry about blanking out during a critical coding question, even though you’ve practiced similar problems? Are you concerned that an interviewer’s follow-up questions about optimizations might leave you speechless? Have you ever started writing code during an interview, only to get stuck halfway due to forgotten edge cases?
These are common fears among many Amazon candidates. Today, we’ll share the real experience of a candidate who successfully navigated his Amazon interview with the help of CSOAHELP’s remote interview assistance. You’ll see how structured guidance and real-time support can make all the difference.
The candidate, whom we’ll refer to as A, had two years of software development experience. While he had practiced LeetCode problems, he wasn’t entirely confident in his ability to clearly articulate solutions under pressure. After receiving the interview invite, he realized that nervousness could impact his performance and sought assistance from CSOAHELP’s remote interview service. Before the interview, the CSOAHELP team conducted a mock session, familiarizing him with Amazon’s interview style and preparing structured guidance to ensure he could respond smoothly during the real interview.
The interview began with a quick introduction, followed by an algorithm question. The interviewer presented the Number of Islands problem:
Number of Islands Problem
Given an m x n 2D binary gridgrid
which represents a map of'1'
s (land) and'0'
s (water), return the number of islands.
An island is surrounded by water and is formed by connecting adjacent lands horizontally or vertically.
You may assume all four edges of the grid are all surrounded by water.
Example 1:
grid = [
["1","1","1","1","0"],
["1","1","1","1","0"],
["1","1","1","0","0"],
["0","0","0","0","0"]
]
Output:
1
Example 2:
grid = [
["1","1","0","0","0"],
["1","1","0","0","0"],
["0","0","1","0","0"],
["0","0","0","1","1"]
]
Output:
3
At first, A recognized that this was a classic graph traversal problem requiring Depth-First Search (DFS) or Breadth-First Search (BFS). However, due to nervousness, he struggled to articulate his thoughts clearly. CSOAHELP’s remote assistant immediately provided a structured breakdown of the solution, allowing A to repeat it naturally.
“This problem can be solved using graph traversal, where each '1'
represents a land cell in a grid-based map. The goal is to count the number of connected island regions. We iterate through the entire grid
, and whenever we encounter a '1'
, we initiate a DFS or BFS search to explore all connected '1'
s, marking them as visited to avoid double-counting. The number of islands corresponds to the number of times we start a new DFS/BFS search.”
To ensure clarity, CSOAHELP further provided a full code template. A read the explanation aloud and entered the following code:
def numIslands(grid):
if not grid:
return 0
def dfs(i, j):
if i < 0 or j < 0 or i >= len(grid) or j >= len(grid[0]) or grid[i][j] == "0":
return
grid[i][j] = "0"
dfs(i + 1, j)
dfs(i - 1, j)
dfs(i, j + 1)
dfs(i, j - 1)
count = 0
for i in range(len(grid)):
for j in range(len(grid[0])):
if grid[i][j] == "1":
dfs(i, j)
count += 1
return count
The interviewer nodded in approval but quickly followed up: “What is the time complexity of your solution? Do you see any ways to optimize it?”
A moment of silence followed as A struggled to come up with an optimization approach. He hadn’t studied alternative solutions in-depth and felt unsure how to proceed. CSOAHELP’s remote assistant immediately suggested a key optimization technique: Union-Find (Disjoint Set Union, DSU). A saw the prompt and confidently responded:
“The current approach runs in O(m × n) time complexity because each grid cell is visited at most once. However, in large grids, the recursion depth of DFS may cause stack overflow. A more optimized solution would use Union-Find, which efficiently manages connected components and allows rapid merging.”
The interviewer asked, “Can you explain how Union-Find works and implement it?”
A saw CSOAHELP’s full implementation of Union-Find and entered the following code:
class UnionFind:
def __init__(self, size):
self.parent = list(range(size))
self.rank = [0] * size
def find(self, x):
if self.parent[x] != x:
self.parent[x] = self.find(self.parent[x]) # Path compression
return self.parent[x]
def union(self, x, y):
rootX = self.find(x)
rootY = self.find(y)
if rootX != rootY:
if self.rank[rootX] > self.rank[rootY]:
self.parent[rootY] = rootX
elif self.rank[rootX] < self.rank[rootY]:
self.parent[rootX] = rootY
else:
self.parent[rootY] = rootX
self.rank[rootX] += 1
def numIslands(grid):
if not grid:
return 0
rows, cols = len(grid), len(grid[0])
uf = UnionFind(rows * cols)
for i in range(rows):
for j in range(cols):
if grid[i][j] == "1":
index = i * cols + j
if i > 0 and grid[i-1][j] == "1":
uf.union(index, (i-1) * cols + j)
if j > 0 and grid[i][j-1] == "1":
uf.union(index, i * cols + (j-1))
return len(set(uf.find(i * cols + j) for i in range(rows) for j in range(cols) if grid[i][j] == "1"))
The interviewer was impressed with A’s clear explanation and well-structured code. Ultimately, A successfully passed this round of the interview.
Amazon interviews are not easy, but CSOAHELP’s remote assistance ensures that candidates never get stuck in high-pressure situations. By providing structured explanations, real-time code assistance, and follow-up question guidance, CSOAHELP helps candidates present their best selves in interviews. If you want to ensure a smooth and confident interview experience, CSOAHELP is your most reliable partner for success!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
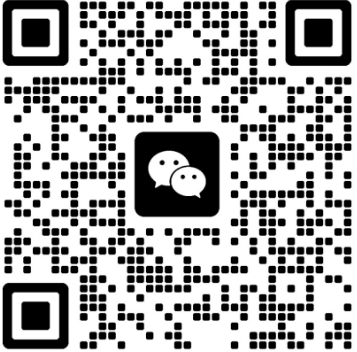