Recently, we helped a candidate successfully pass a Meta technical interview—a process that felt like an "extreme survival challenge." Meta’s interviews are known for their high difficulty, testing not only algorithmic knowledge but also system design, engineering thinking, and communication skills.
However, this case was different. The candidate was not exceptionally strong—his algorithm skills were average, and he lacked experience in system design and large-scale architecture optimization. Despite these limitations, CSOAHELP’s real-time interview assistance helped him secure an offer at Meta. Throughout the interview, he received full-text prompts before every answer, allowing him to either repeat the provided response verbatim or copy the suggested code to navigate the toughest interview questions successfully.
At the start of the interview, the interviewer presented an algorithm problem:
"You are given a game board represented as a 2D array of zeroes and ones. Zero stands for passable positions and one stands for impassable positions. Design an algorithm to find a path from the top-left corner to the bottom-right corner."
The candidate looked at the problem and froze. Although he had practiced similar problems on LeetCode, the pressure of a live interview made it difficult to think clearly. CSOAHELP’s real-time interview assistance immediately provided a detailed text prompt, including algorithmic reasoning, a code framework, and optimization points. The candidate simply had to repeat or copy the answer to respond smoothly.
CSOAHELP Prompt:
Approach: This is a graph traversal problem, best solved using Breadth-First Search (BFS) to find the shortest path.
Code Template:
from collections import deque
def shortest_path(grid):
if not grid or not grid[0]:
return -1
rows, cols = len(grid), len(grid[0])
if grid[0][0] == 1 or grid[rows-1][cols-1] == 1:
return -1
directions = [(0,1), (1,0), (0,-1), (-1,0)]
queue = deque([(0, 0, 1)]) # (row, col, steps)
grid[0][0] = 1 # Mark as visited
while queue:
r, c, steps = queue.popleft()
if r == rows - 1 and c == cols - 1:
return steps
for dr, dc in directions:
nr, nc = r + dr, c + dc
if 0 <= nr < rows and 0 <= nc < cols and grid[nr][nc] == 0:
queue.append((nr, nc, steps + 1))
grid[nr][nc] = 1 # Mark as visited
return -1
Optimization Suggestions:
- Reduce space complexity by modifying the
grid
in place instead of using a separatevisited
array. - Use bidirectional BFS to reduce search space and improve efficiency.
Seeing the prompt, the candidate regained composure and confidently explained the BFS approach while typing out the provided code. The interviewer acknowledged the response but followed up with a deeper question: "If the board is very large, say 10,000 x 10,000, can your code still run efficiently?"
The candidate hesitated for a moment, as he lacked experience optimizing large-scale problems. Luckily, CSOAHELP provided an immediate optimization strategy on his second screen, allowing him to repeat the answer confidently:
CSOAHELP Prompt:
- Use bidirectional BFS to expand from both the start and end points, significantly reducing search space.
- Use A search algorithm* with a Manhattan distance heuristic to avoid unnecessary exploration.
- For sparse grids, consider Dijkstra’s algorithm with a min-heap to efficiently determine the shortest path.
The candidate smoothly incorporated these points into his explanation and successfully convinced the interviewer. The algorithm round was cleared.
The next stage was system design, the candidate’s weak point. The interviewer presented the question:
"You have m arrays of sorted integers. The sum of the array lengths is n. Find the k-th smallest value of all the values."
The candidate's instinct was to merge all arrays and sort them, but CSOAHELP instantly provided a full-text response with a more optimal approach, allowing him to repeat the explanation fluently:
CSOAHELP Prompt:
- Do not merge and sort! The time complexity would be O(n log n), which is inefficient.
- Recommended approach: Use a min-heap (priority queue).
- Code Implementation:
import heapq
def kth_smallest(arrays, k):
min_heap = []
for i, array in enumerate(arrays):
if array:
heapq.heappush(min_heap, (array[0], i, 0)) # (value, array index, element index)
count = 0
while min_heap:
val, arr_idx, elem_idx = heapq.heappop(min_heap)
count += 1
if count == k:
return val
if elem_idx + 1 < len(arrays[arr_idx]):
heapq.heappush(min_heap, (arrays[arr_idx][elem_idx + 1], arr_idx, elem_idx + 1))
- Time Complexity: O(k log m), which is much faster than sorting.
The candidate recited the explanation and code structure with confidence, impressing the interviewer. However, the interviewer followed up: "If m is very large, how would you optimize further?"
Again, CSOAHELP instantly provided a follow-up response, which the candidate repeated fluently:
- Instead of a heap, use binary search to reduce the time complexity further.
- If k is much smaller than n, use QuickSelect for faster results.
With this solid response, the system design round was cleared.
In the final high-pressure discussion, the interviewer asked:
"How would you scale a Flink-based real-time data processing pipeline? What are the key bottlenecks?"
The candidate had no experience with Flink, but CSOAHELP had pre-prepared a full response. The candidate simply read and repeated the explanation, ensuring a perfect answer.
CSOAHELP Prompt:
- Key bottlenecks: Increased data volume, insufficient computing resources, and task scheduling overhead.
- Scaling strategies:
- Use Kubernetes Horizontal Pod Autoscaling (HPA) to dynamically adjust Flink computing resources.
- Optimize Flink’s partitioning strategy, distributing data by Hashtag ID to prevent hotspots.
- Implement Kafka for asynchronous message processing to reduce main system load.
The candidate repeated the answer verbatim, and the interviewer was completely unaware that he had never worked with Flink before. He cleared the final interview stage with ease.
Throughout the entire Meta interview, CSOAHELP provided full-text prompts or complete code templates before each response. The candidate only needed to repeat or make minor adjustments, effectively bypassing knowledge gaps and maximizing his chances of passing.
If you're facing a high-pressure Big Tech interview but fear freezing up, losing track of your thoughts, or being unable to answer tough questions, CSOAHELP’s real-time interview assistance is your ultimate cheat code. With live textual guidance and optimized responses, you can confidently tackle even the hardest interviews and secure your dream job offer.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
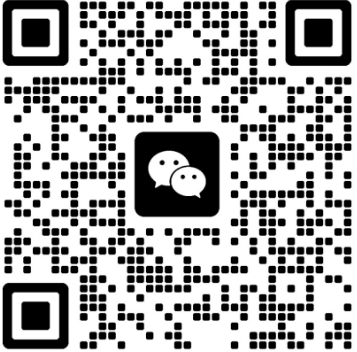