If you're preparing for interviews at top firms like Citadel or aiming to break into hedge funds and quantitative trading companies on Wall Street, you might think algorithm problems are just a warm-up. The real challenge lies in staying composed under pressure and articulating the most optimal solution within a short time frame. Today, we’ll walk through a real Citadel remote interview experience where a candidate encountered a classic stock trading problem and how CSOAHELP’s real-time interview assistance ensured he successfully passed the interview.
The interviewer shared his screen in the Zoom meeting and presented the following stock trading problem:
// You are given an array prices where prices[i] is the price of a
// given stock on the ith day.
// Find the maximum profit you can achieve. You may complete at most
// two transactions.
// Note: You may not engage in multiple transactions simultaneously (i.
// e., you must sell the stock before you buy again).
// Example 1:
// Input: prices = [3,3,5,0,0,3,1,4]
// Output: 6
// Explanation: Buy on day 4 (price = 0) and sell on day 6 (price = 3),
// profit = 3-0 = 3.
// Then buy on day 7 (price = 1) and sell on day 8 (price = 4), profit =
// 4-1 = 3.
// Example 2:
// Input: prices = [1,2,3,4,5]
// Output: 4
// Explanation: Buy on day 1 (price = 1) and sell on day 5 (price = 5),
// profit = 5-1 = 4.
// Note that you cannot buy on day 1, buy on day 2 and sell them later,
// as you are engaging multiple transactions at the same time. You must
// sell before buying again.
The interviewer smiled and said, "You’ve probably seen this problem before. It’s a classic stock trading question. But this time, you can complete at most two transactions. Let's see how you solve it."
The candidate saw the question and had some ideas in mind, but the pressure of the interview made it difficult to decide where to begin. At that moment, CSOAHELP’s real-time interview assistance provided structured hints on a secondary screen, ensuring he didn’t miss critical points or get lost in his explanation.
After seeing the hints, the candidate took a deep breath and started explaining his approach: "This problem can be solved using dynamic programming (DP). We need to maintain four states: the lowest cost of the first buy, the maximum profit of the first sell, the lowest cost of the second buy, and the maximum profit of the second sell."
While explaining, he wrote down the initial state of the variables in the code editor:
def maxProfit(prices):
if not prices:
return 0
firstBuy, firstSell = float('-inf'), 0
secondBuy, secondSell = float('-inf'), 0
"firstBuy tracks the lowest cost of the first transaction, firstSell records the maximum profit after the first sell, secondBuy tracks the lowest cost of the second transaction, and secondSell stores the maximum profit after the second sell."
"Next, we iterate through the price array and update these four states step by step."
for price in prices:
firstBuy = max(firstBuy, -price)
firstSell = max(firstSell, firstBuy + price)
secondBuy = max(secondBuy, firstSell - price)
secondSell = max(secondSell, secondBuy + price)
return secondSell
He continued to explain the logic: "firstBuy = max(firstBuy, -price) represents the cost of the first buy, aiming to buy at the lowest price possible. firstSell = max(firstSell, firstBuy + price) calculates the maximum profit from the first transaction. secondBuy = max(secondBuy, firstSell - price) finds the minimum cost of the second buy while considering the profit from the first sell. secondSell = max(secondSell, secondBuy + price) computes the final maximum profit after two transactions."
The interviewer nodded in acknowledgment and then asked, "What is the time complexity of your solution? Do you see any room for optimization?"
The candidate briefly paused, and CSOAHELP quickly provided a hint on the secondary screen. He immediately responded: "The time complexity of this solution is O(n) because we iterate through the prices array only once. The space complexity is O(1) since we only use a fixed number of variables without additional storage. This is already an optimal solution, but we could improve code readability by using more intuitive variable names."
The interviewer seemed satisfied and followed up with a more challenging question: "What if we allow at most k transactions? How would you extend your solution?"
The candidate took a moment to think, and CSOAHELP instantly provided guidance. He confidently replied: "If we allow up to k transactions, we can use a DP table where dp[i][j] represents the maximum profit on day i with at most j transactions. To optimize space complexity, we can use rolling arrays."
He added, "For real-world financial applications, we may also need to optimize using sliding window techniques to handle high-frequency trading scenarios more efficiently."
The interviewer nodded approvingly and said, "That’s a great answer."
After the interview ended, the candidate took a deep breath of relief. He knew that without CSOAHELP’s real-time interview assistance, he might have missed key points, struggled to articulate his solution, or even failed to answer correctly under the pressure.
At Citadel, the interview isn’t just about solving an algorithmic problem. It’s an assessment of your ability to think critically, communicate effectively, and demonstrate a structured approach to problem-solving.
If you’re aiming for Citadel, Two Sigma, Jane Street, or similar top-tier financial tech firms, CSOAHELP’s real-time interview assistance ensures that you remain confident, articulate, and structured during your interview.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
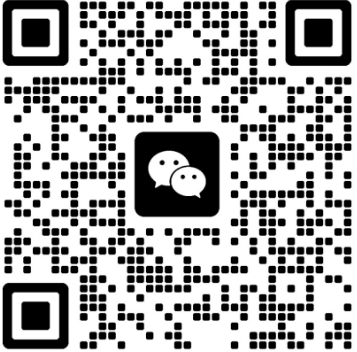