Minimum Flips to Match Expected Binary Values in a Tree
Problem Statement
You are given an undirected tree with tree_nodes nodes, numbered from 0 to tree_nodes - 1, and rooted at node 0. Each node initially has a binary value (0 or 1) given by the array initial, and an expected binary value given by the array expected.
You can perform the following operation any number of times:
- Pick a node and flip its value along with all nodes in the subtree of
u
whereu % 2 == x % 2
. Flipping a value means toggling between 0 and 1.
Find the minimum number of operations needed so that the final binary values match expected.
Example 1
Input:
tree_nodes = 4
tree_from = [0, 0, 1]
tree_to = [1, 2, 3]
initial = [0, 1, 1, 0]
expected = [0, 1, 1, 1]
Output:
2
Explanation:
- Pick node 0 and flip it → initial =
[0, 1, 1, 1]
- Pick node 3 and flip it → initial =
[0, 1, 1, 0]
(matches expected)
Example 2
Input:
tree_nodes = 5
tree_from = [0, 1, 2, 4]
tree_to = [1, 2, 3, 1]
initial = [1, 1, 0, 1, 0]
expected = [1, 0, 1, 0, 1]
Output:
3
Problem Statement
You are given a list of products, where each product has a name, price, and weight. Your task is to determine how many duplicate products are in the list. A duplicate product is defined as a product that has the same name, price, and weight as another product in the list.
Function Signature
public static int numDuplicates(List name, List price, List weight)
Parameters
name
: A list of strings wherename[i]
represents the name of the product at indexi
.price
: A list of integers whereprice[i]
represents the price of the product at indexi
.weight
: A list of integers whereweight[i]
represents the weight of the product at indexi
.
Returns
- An integer denoting the number of duplicate products in the list.
Constraints
1 ≤ n ≤ 10⁶
1 ≤ price[i], weight[i] ≤ 1000
name[i]
consists of lowercase English letters (a-z) and has at most 10 characters.
Example 1
Input:
name = ["ball", "box", "ball", "ball", "box"]
price = [2, 2, 2, 2, 2]
weight = [1, 1, 1, 1, 3]
Output:
2
Explanation:
- Products at indices 0, 2, 3 have the same attributes (
name = "ball"
,price = 2
,weight = 1
). - There are 2 duplicates in this group.
- "box" at index 1, 4 have different weights, so they are unique.
Example 2
Input:
name = ["ball", "box", "lamp", "brick", "pump"]
price = [2, 2, 2, 2, 2]
weight = [2, 2, 2, 2, 2]
Output:
0
Explanation:
Each product has unique attributes, so there are no duplicates.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
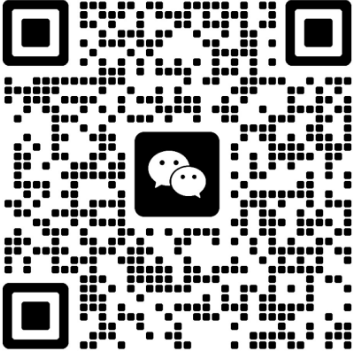