Google’s technical interviews are known for their high quality, fast pace, and deep follow-up questions. Candidates not only need to write correct code but must also articulate their thought process clearly and confidently respond to in-depth questions from interviewers. Many candidates can easily solve LeetCode problems at home, but in a real interview setting, nervousness, disorganized thinking, or unclear explanations often lead to suboptimal performance and missed opportunities. CSOAHELP’s real-time coding and transcript assistance is designed to address these challenges, ensuring that candidates perform at their best during the interview, from code implementation to verbal expression.
In a real Google interview, a candidate was asked to solve the following problem:
"Given a binary tree, compute the diameter of the tree. Diameter: the longest path between any two nodes."
Example input:
1
/ \
2 3
/ \
4 5
The core of this problem is to determine the diameter of a binary tree, which represents the longest path between any two nodes, not necessarily passing through the root. Candidates need to quickly grasp the problem, implement an efficient solution, and refine their approach in response to follow-up questions from the interviewer. Under high pressure, many candidates encounter the following difficulties:
- Disorganized thinking: Struggling to come up with a solution quickly, getting confused with recursive base cases or subproblem decomposition.
- Code flaws: Failing to correctly implement recursive traversal for both left and right subtrees, leading to incorrect outputs for certain test cases.
- Time complexity issues: Writing an O(N²) brute-force solution without optimizing it to the more efficient O(N) approach.
- Unclear explanations: Becoming nervous when asked to explain optimizations, leading to a poor impression.
CSOAHELP provides real-time coding assistance in such interviews, helping candidates structure their thoughts, refine their code, and offering transcript assistance to ensure their explanations are logical and precise.
The key insight in solving this problem is that the tree diameter is calculated as the sum of the maximum depths of the left and right subtrees. A recursive DFS approach allows for efficient computation while keeping track of the maximum diameter. The optimal solution is as follows:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def diameter_of_binary_tree(root):
max_diameter = [0]
def depth(node):
if not node:
return 0
left_depth = depth(node.left)
right_depth = depth(node.right)
max_diameter[0] = max(max_diameter[0], left_depth + right_depth)
return max(left_depth, right_depth) + 1
depth(root)
return max_diameter[0]
During real interviews, many candidates can write a basic recursive solution, but they may struggle when the interviewer asks follow-up optimization questions. For example:
- Interviewer: What is the time complexity of your code? Can you optimize it further?
- Candidate (stuck, unable to respond quickly)
- Interviewer: If the tree depth is on the order of 10^5, how would you handle recursion depth limits?
- Candidate (unprepared, unable to provide a smooth answer)
- Interviewer: If this were an N-ary tree instead of a binary tree, how would you modify your approach?
- Candidate (nervous, struggling with explanation, breaking logical flow)
If a candidate cannot provide satisfactory answers to these key questions, their evaluation score may decrease, resulting in a failed interview. CSOAHELP’s real-time transcript assistance helps candidates prepare standardized answers in advance, ensuring they respond fluently and confidently in the interview. Example responses include:
- "My solution uses recursion and depth-first search (DFS), with a time complexity of O(N), where N is the total number of nodes in the binary tree."
- "If the tree depth is very large, Python’s recursion limit may be exceeded. We can switch to an iterative approach or manually increase the recursion limit using
sys.setrecursionlimit()
." - "For an N-ary tree, we need to modify the
depth
function to iterate through all child nodes instead of limiting it to left and right subtrees."
At companies like Google, Amazon, and Meta, writing correct code alone is not enough. True competitiveness comes from:
- Quickly structuring thoughts under pressure
- Avoiding implementation mistakes during coding
- Clearly articulating responses when questioned by interviewers
- Optimizing code efficiency within limited time constraints
Many candidates fail in interviews not because they lack problem-solving skills, but due to disorganized thinking, unclear explanations, and minor coding mistakes, which ultimately cause interviewers to doubt their capabilities. The value of CSOAHELP’s real-time coding and transcript assistance lies in ensuring candidates not only write correct code but also express themselves confidently, think clearly, and respond precisely, ultimately securing their offer!
If you are preparing for technical interviews at Google, Waymo, Meta, or Amazon, CSOAHELP’s remote real-time interview assistance is your most reliable choice. It not only helps optimize your code but also provides live coding support, structured thinking guidance, and verbal expression coaching, ensuring you handle all challenges with confidence and secure your dream offer!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
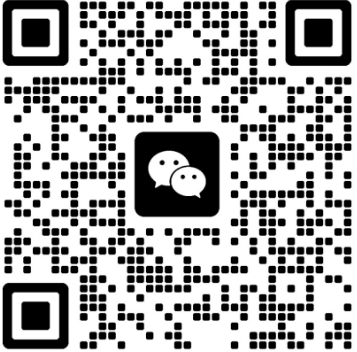