Think about a team chat with numerous users writing messages in it. The chat supports two types of actions:
["MESSAGE", "<timestamp>", "<mentions>"]
- Messages a set of users. The format looks as follows.
<mentions>
string can contain the following space-separated tokens:id<number>
where<number>
is an integer in range[1:999]
- mentioning of individual users.ALL
- mentioning all users.HERE
- mentioning active users.
["OFFLINE", "<timestamp>", "<id>"]
- Makes a user with a given id inactive for
60
time ticks – the user will be active again at time<timestamp> + 60
. It is guaranteed that the user with given<id>
will be active when this action is applied.
- Makes a user with a given id inactive for
Note: all the events are sorted by their timestamp.
Examples of events
["MESSAGE", "0", "id1"]
– mentioning user with idid1
.["MESSAGE", "9", "HERE id3"]
– mentioning all the active users and user with idid3
.["MESSAGE", "5", "ALL"]
– mentioning all the users.["MESSAGE", "100", ""]
– message, without mentioning any user.["OFFLINE", "200", "id3"]
– making user with idid3
inactive.
Please note, that mentions do not contain any other text, but only a list of ids or aliases separated by a space.
Your task is to calculate mention statistics. Given a list of users in the group chat and a list of chat events, count the number of message events that each user is mentioned in.
Return the results in an array of strings, with each string following this format: ["user id|mentions count"]
. The array should be sorted lexicographically by user id in ascending order.
Note: You are not expected to provide the most optimal solution, but a solution with time complexity not worse than O(members.length * events.length)
will fit within the execution time limit.
Example
Formembers = ["id42", "id158", "id23"]
andevents = [ ["MESSAGE", "0", "ALL id158 id42"], ["OFFLINE", "1", "id158"], ["MESSAGE", "5", "id158 id158"], ["OFFLINE", "3", "id23"], ["MESSAGE", "6", "HERE id158 id42 id23"], ["MESSAGE", "61", "HERE"] ]
the output should be solution(members, events) = ["id158-4", "id23-2", "id42-3"]
.
Explanation:
- In the first event at time
0
, all users are mentioned withALL
alias. Note thatid158
andid42
are mentioned twice – once byALL
alias and once by their ids, but they should be counted once. - In the second event at time
1
the userid158
becomes inactive. - In the second event at time
1
the userid158
becomes inactive. - In the third event at time
2
the userid158
is mentioned and they get notified even when inactive. - In the third event at time
2
the userid158
is mentioned twice, but it should be counted once. - In the fourth event at time
3
the userid23
becomes inactive. - In the fifth event at time
6
, all active users andid158
,id42
, andid23
are mentioned. Note thatid158
andid23
are mentioned by their username, so they will be notified even when they are inactive, andid42
is mentioned twice – once byHERE
alias and once by their id, but it should be counted once. - In the last event at time
61
, the user withid158
is back online again as they have been offline for60
time ticks already. Here all the active users are mentioned – which areid42
andid158
.
So, the output should be ["id158-4", "id23-2", "id42-3"]
.
Input/Output
[execution time limit] 0.5 seconds (cpp)
[memory limit] 1 GB
[input] array.string members
- Member id list.
- Guaranteed constraints:
2 ≤ members.length ≤ 50
,3 ≤ members[i].length ≤ 5
.
[input] array.array.string events
- Events occurred in chronological order. Note, that timestamps can be in range
[0:200]
. - Guaranteed constraints:
1 ≤ events.length ≤ 100
.
- Events occurred in chronological order. Note, that timestamps can be in range
[output] array.string
- Return an array of strings containing all user ids from
members
, with count of each user id mentions acrossevents
(described above) separated by-
sign. This array should be sorted lexicographically by user id in ascending order.
- Return an array of strings containing all user ids from
[C++] Syntax Tips
// Prints help message to the console
// Returns a string
string helloWorld(string name) {
cout << "This prints to the console when you Run Tests" << endl;
return "Hello, " + name;
}
For a grid of black and white cells with rows
rows and cols
columns, you're given an array black
that contains the [row, column]
coordinates of all the black cells in the grid.
Your task is to compute how many 2 × 2
submatrices of the grid contain exactly blackcount
black cells for each 0 ≤ blackcount ≤ 4
. As a result, you will return an array of 5
integers, where the i
th element is the number of 2 × 2
submatrices with exactly i
black cells.
It is guaranteed that black cell coordinates in the black
array are pairwise unique, so the same cell is not colored twice.
Example
For rows = 3
, cols = 3
, and black = [[0, 0], [0, 1], [1, 0]]
, the output should be solution(rows, cols, black) = [1, 2, 0, 1, 0]
.
Input/Output
[execution time limit] 0.5 seconds (cpp)
[memory limit] 1 GB
[input] integer rows
- An integer representing the number of rows in the grid.
- Guaranteed constraints:
2 ≤ rows ≤ 10⁵
.
[input] integer cols
- An integer representing the number of columns in the grid.
- Guaranteed constraints:
2 ≤ cols ≤ 10⁵
.
[input] array.array.integer black
- An array of black cells. Each black cell is represented as a
2-element
array[row, column]
. Black cells are guaranteed to be all unique. - Guaranteed constraints:
0 ≤ black.length ≤ 500
,black[i].length = 2
,0 ≤ black[i][0] < rows
,0 ≤ black[i][1] < cols
.
- An array of black cells. Each black cell is represented as a
[output] array.integer64
- An array of
5
integers, where thei
th element is the number of2 × 2
submatrices in the grid withi
black cells.
- An array of
[C++] Syntax Tips
// Prints help message to the console
// Returns a string
string helloWorld(string name) {
cout << "This prints to the console when you Run Tests" << endl;
return "Hello, " + name;
}
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
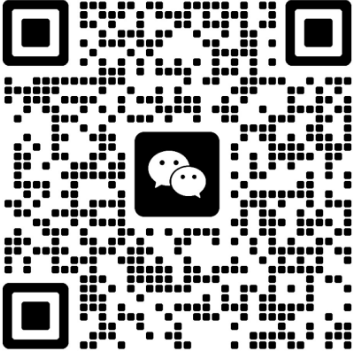