Imagine a video game where the player controls a character to go through several levels. The character has an initial health value of initialHealth
and this value changes as the player goes through levels of the game.
You are given an array of integers deltas
, defining each health value change. Specifically, the i
th level (0
-indexed) changes the character's current health value by deltas[i]
. Note that whenever the current health value becomes less than 0
, it immediately gets set to 0
. Similarly, whenever the current health value becomes greater than 100
, it immediately gets set to 100
.
Your task is to return the character's final health value after the player goes through all levels of the game.
Note: You are not expected to provide the most optimal solution, but a solution with time complexity not worse than O(deltas.length)
will fit within the execution time limit.
Example
For initialHealth = 12
and deltas = [-4, -12, 6, 2]
, the output should be solution(initialHealth, deltas) = 8
.
Let's consider each level:
- At the beginning, the current health value is
currentHealth = initialHealth = 12
. - After completing the
0
th level, the current health value becomescurrentHealth + deltas[0] = 12 + (-4) = 8
. - After completing the
1
st level, the current health value becomescurrentHealth + deltas[1] = 8 + (-12) = -4
, but since the health value cannot be less than0
, it gets set to0
. - After completing the
2
nd level, the current health value becomescurrentHealth + deltas[2] = 0 + 6 = 6
. - After completing the
3
rd level, the current health value becomescurrentHealth + deltas[3] = 6 + 2 = 8
, which is the final answer.
Input/Output
[execution time limit] 0.5 seconds (cpp)
[memory limit] 1 GB
[input] integer initialHealth
- An integer representing the initial health value of the player.
- Guaranteed constraints:
0 ≤ initialHealth ≤ 100
.
[input] array.integer deltas
- An array of integers representing changes to the current health value after the player completes each level.
- Guaranteed constraints:
1 ≤ deltas.length ≤ 100
,-100 ≤ deltas[i] ≤ 100
.
[output] integer
- An integer representing the final health value of the player.
[C++] Syntax Tips
// Prints help message to the console
// Returns a string
string helloWorld(string name) {
cout << "This prints to the console when you Ru";
return "Hello, " + name;
}
Imagine a fantasy card duel between two strategists. Each player has a deck, playerDeckA
and playerDeckB
, and each card showcases a mystical creature with a power value between 1
to 10
. During each round, the top cards from both players' decks determine the victor of that round based on these simple rules:
- Each player reveals the top card of their deck.
- If the creature's power from
playerDeckA
is greater than or equal to the one fromplayerDeckB
, the first player claims victory in that round, securing both cards to the bottom ofplayerDeckA
withplayerDeckB
's card at their card's bottom. - If the creature's power from
playerDeckA
is less than the one fromplayerDeckB
, the second player claims both cards, placing them at the bottom ofplayerDeckB
withplayerDeckA
's card at their card's bottom. - The game ends when a player's deck is empty, leaving them unable to draw another creature for battle.
Determine how many rounds this duel lasts. It's guaranteed that the showdown will conclude at some point.
Note: You are not expected to provide the most optimal solution, but a solution with time complexity not worse than O(playerDeckA.length²)
will fit within the execution time limit.
Example
For playerDeckA = [5]
and playerDeckB = [2]
, the output should be solution(playerDeckA, playerDeckB) = 1
.
Breakdown of each round:
- Round 1:
playerDeckA
plays a5
, whereasplayerDeckB
presents a2
. The decks temporarily become empty.- Since
5 ≥ 2
,5
and2
move to the bottom ofplayerDeckA
. - Afterwards,
playerDeckA = [5, 2]
andplayerDeckB = []
. - With
playerDeckB
empty, the duel is over, resulting in a single round:1
.
For playerDeckA = [1, 2]
and playerDeckB = [3, 1]
, the output should be solution(playerDeckA, playerDeckB) = 6
.
Input/Output
[execution time limit] 0.5 seconds (cpp)
[memory limit] 1 GB
[input] array.integer playerDeckA
- An array of integers denoting the first player's deck from top to bottom.
- Guaranteed constraints:
1 ≤ playerDeckA.length ≤ 100
,1 ≤ playerDeckA[i] ≤ 10
.
[input] array.integer playerDeckB
- An array of integers denoting the second player's deck from top to bottom.
- Guaranteed constraints:
playerDeckB.length = playerDeckA.length
,1 ≤ playerDeckB[i] ≤ 10
.
[output] integer
- Returns the total number of rounds the duel lasts.
[C++] Syntax Tips
// Prints help message to the console
// Returns a string
string helloWorld(string name) {
cout << "This prints to the console when you Run Tests" << endl;
return "Hello, " + name;
}
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
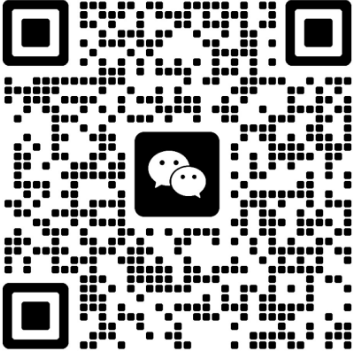