- Factorial Calculation
Implement a function factorial(n)
that calculates the factorial of a given number n
. Choose the correct implementation.
function factorial(n) {
if (n <= 1) {
return 1;
}
return <code>
}
Pick ONE option
return n * factorial(n - 1)
return factorial(n) * n
- Prime Number Check
Write a function isPrime
that returns true
if a number n
is prime and false
otherwise. Select the correct implementation.
function isPrime(n) {
if (n <= 1) {
return false;
}
for (i = 2; i < n; i++) {
if (<code>) {
return false;
}
}
return true;
}
Pick ONE option
if (n % i === 0)
- Balancing Server Loads with Dynamic Data Structure
A web server distributes load across multiple servers to balance traffic. To ensure real-time load balancing, the server with the least load should be dynamically selected as requests arrive. Which implementation method best achieves real-time selection of the least-loaded server?
Pick ONE option
initialize array loads
sort loads in ascending order
return first element as least-loaded server
initialize min-heap loads
for each request:
server = extract_min loads
process request on server
re-insert server with updated load
- Real-Time Navigation System Using Dijkstra’s Algorithm
A company developing a real-time navigation system needs an efficient algorithm to find the shortest path from a user’s location to a destination across a large network of roads and intersections. The system must update dynamically as new road conditions and traffic data are received.
Which of the following pseudocode implementations best suits the requirement for finding the shortest path in a weighted graph?
Pick ONE option
initialize empty queue path
enqueue start node to path
while path is not empty:
node = dequeue path
if node is destination:
return shortest path
add all connected nodes to path
initialize empty set visited
initialize stack path
push start node to path
while path is not empty:
node = pop path
if node is destination:
return path
for each neighbor of node:
if neighbor not in visited:
push neighbor to path
initialize priority queue pq
initialize distances to infinity for all nodes
set distance of start node to 0
add start node to pq
while pq is not empty:
node = extract_min pq
for each neighbor of node:
if distance to neighbor > distance to node + edge weight:
update distance to neighbor
add neighbor to pq
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
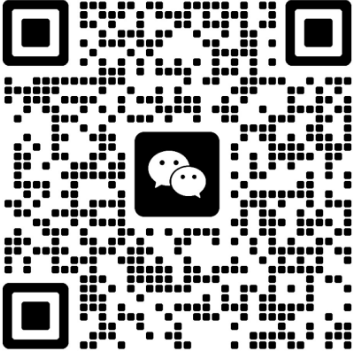