Minimum Operations to Ensure No Two Adjacent Characters Are Equal
Problem Statement
Given a lowercase character string str
of size N
, in one operation, any character can be changed into another character.
The goal is to find the minimum number of operations required such that no two adjacent characters are equal.
Examples
Example 1
Input:
str = "caaab"
Output:
1
Explanation:
- Change the second
'a'
to any other character (e.g.,'b'
), making the string"cabab"
. - No adjacent characters are equal.
- Minimum number of operations = 1.
Example 2
Input:
str = "xxxxxxx"
Output:
3
Explanation:
- Replace
'x'
at index 1, 3, and 5 with different characters (e.g.,'a'
,'b'
,'c'
). - Resulting string:
"xaxbxcx"
. - Minimum number of operations = 3.
Approach
- Identify contiguous substrings where all characters are the same.
- For each such substring of length
l
, at leastfloor(l / 2)
replacements are required. - Iterate over the string, grouping consecutive characters together.
- Compute
floor(l / 2)
for each group and sum up the values.
Time Complexity Analysis
- O(N) time complexity as we iterate over the string once.
- O(1) space complexity since we only use a few extra variables.
Algorithm
- Initialize
operations = 0
, which will store the total required operations. - Traverse the string to find contiguous segments of the same character.
- For each segment of length
l
, computefloor(l / 2)
and add it tooperations
. - Return the final
operations
count.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
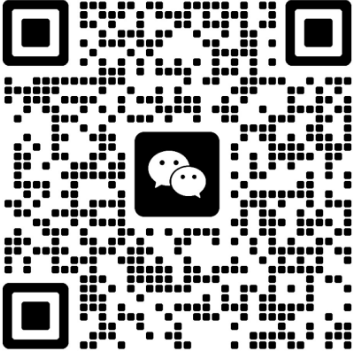